A Step-by-Step Guide to Creating a Login Page in ReactJS
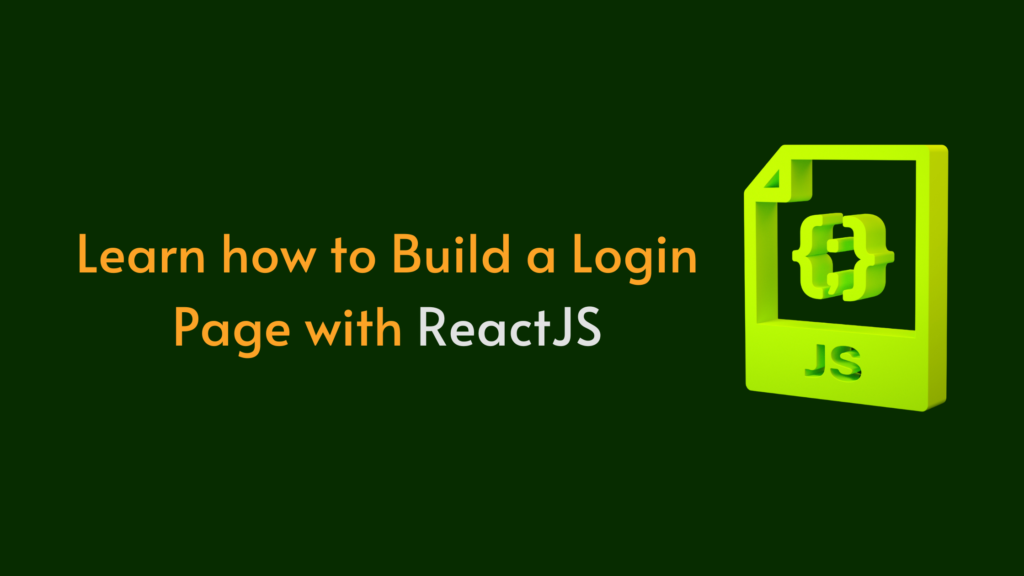
Introduction:
The login page is a fundamental element of any web application, providing users with secure access to their accounts. In this blog post, we’ll walk you through the process of building a login page using ReactJS. By the end of this guide, you’ll have a clear understanding of how to implement user authentication with a practical example.
Prerequisites
Before we start, ensure you have the following prerequisites in place:
- Basic understanding of ReactJS and JavaScript.
- Node.js and npm (Node Package Manager) installed on your machine.
- A code editor of your choice.
Step 1: Setting Up the Project
Begin by creating a new React application. Open your terminal and run the following commands:
npx create-react-app login-page
cd login-page
This will set up a new React project named “login-page.”
Step 2: Creating the Login Form
In the src folder of your project, locate the App.js file. Replace its contents with the following code:
import React, { useState } from ‘react’;
import ‘./App.css’;
function App() {
const [username, setUsername] = useState(”);
const [password, setPassword] = useState(”);
const handleLogin = () => {
// Perform login logic here
};
return (
<div className=”App”>
<h1>Login Page</h1>
<form>
<input
type=”text”
placeholder=”Username”
value={username}
onChange={(e) => setUsername(e.target.value)}
/>
<input
type=”password”
placeholder=”Password”
value={password}
onChange={(e) => setPassword(e.target.value)}
/>
<button type=”button” onClick={handleLogin}>
Login
</button>
</form>
</div>
);
}
export default App;
Step 3: Implementing Login Logic
In the handleLogin
function within App.js
, you would typically connect to a backend server for authentication. For this example, let’s simulate a successful login by checking hardcoded values:
const handleLogin = () => {
if (username === ‘user’ && password === ‘password’) {
alert(‘Login successful!’);
} else {
alert(‘Login failed. Please check your credentials.’);
}
};
Step 4: Styling the Login Page
Style your login page by editing the App.css
file:
.App {
text-align: center;
margin-top: 10vh;
}
form {
display: flex;
flex-direction: column;
gap: 10px;
margin-top: 20px;
}
input {
padding: 8px;
border: 1px solid #ccc;
border-radius: 4px;
}
button {
padding: 10px;
background-color: #007bff;
color: white;
border: none;
border-radius: 4px;
cursor: pointer;
}
Conclusion
Congratulations! You’ve successfully created a basic login page in ReactJS. This guide covers the essentials of building a login form, handling user input, and simulating login logic. As you delve deeper into ReactJS, you can integrate more advanced authentication methods and connect to real backend services for secure user authentication.
Leave a Reply