Exploring Java: A Comprehensive Overview
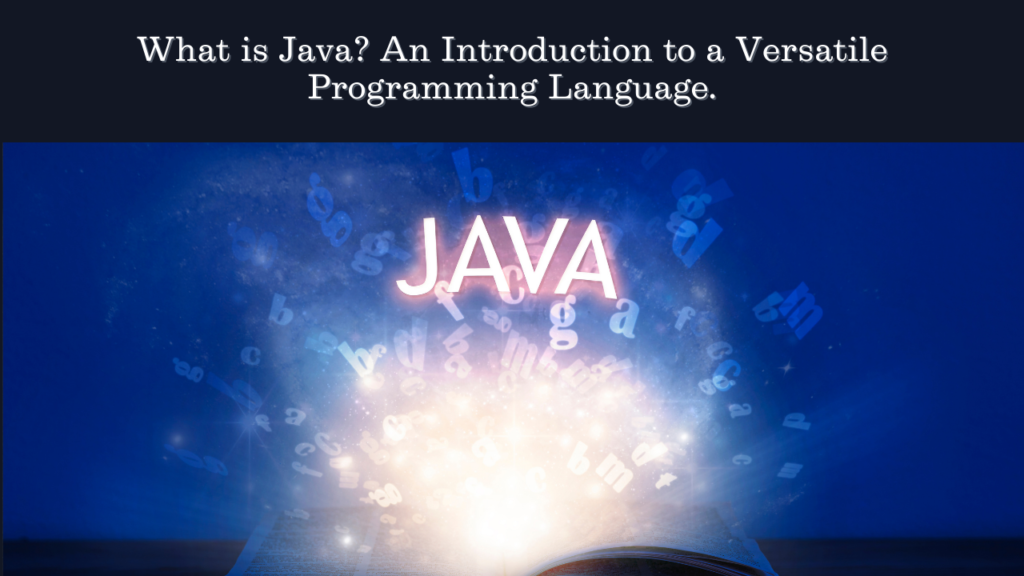
Introduction to Java
Java, developed by Sun Microsystems (now owned by Oracle Corporation), is a high-level, object-oriented programming language renowned for its portability, reliability, and security. It was designed to have minimal implementation dependencies, making it an excellent choice for cross-platform applications.
How is Java Used?
Java finds extensive applications across various domains:
- Web Development: Utilized in backend development using frameworks like Spring and Hibernate.
- Mobile Applications: Android app development relies heavily on Java.
- Enterprise Solutions: Java Enterprise Edition (EE) for robust and scalable enterprise applications.
- Scientific Computing: Used in data analysis and high-performance computing.
Features of Java
- Platform Independence: Runs on the Java Virtual Machine (JVM), allowing code to execute on any platform.
- Object-Oriented: Emphasizes modularity and reusability through objects and classes.
- Robust and Secure: Automatic memory management and built-in security features.
- Multi-threading: Supports concurrent execution for efficient handling of multiple tasks.
- Dynamic: Facilitates dynamic memory allocation and adaptability during runtime.
Difference Between Java and Advanced Java
Java | Advanced Java |
Core Java fundamentals | Advanced concepts and frameworks beyond basics |
Basic syntax and programming skills | Specialized topics like JDBC, Servlets, JSP, etc. |
Entry-level proficiency | In-depth knowledge for complex enterprise applications |
Standard libraries and utilities | Advanced frameworks for specific application needs |
General-purpose programming language | Targeted towards specialized, complex projects |
Other Tools Used with Java
- Integrated Development Environments (IDEs): Eclipse, IntelliJ IDEA, NetBeans facilitate coding and debugging.
- Build Automation Tools: Maven, Gradle streamline the build process.
- Version Control Systems: Git, SVN for efficient code management.
- Testing Frameworks: JUnit, TestNG for automated testing.
The Advantages of Upskilling in Java
- High Demand: Java skills are consistently in demand across industries.
- Versatility: Opportunities span multiple domains and job roles.
- Career Growth: Enhanced Java proficiency leads to better career prospects and higher salaries.
Java Basic Programs with Examples
- Hello World Program:
public class HelloWorld {
public static void main(String[] args) {
System.out.println(“Hello, World!”);
}
}
2. Calculator Program:
public class Calculator {
public static void main(String[] args) {
int num1 = 10;
int num2 = 5;
int sum = num1 + num2;
System.out.println(“Sum: ” + sum);
}
}
Advanced Java Programs with Examples
- Servlet Example:
import javax.servlet.*;
import java.io.*;
public class HelloWorldServlet extends GenericServlet {
public void service(ServletRequest request, ServletResponse response) throws ServletException, IOException {
response.setContentType(“text/html”);
PrintWriter out = response.getWriter();
out.print(“<html><body>”);
out.print(“<h1>Hello, Servlet!</h1>”);
out.print(“</body></html>”);
out.close();
}
}
Java Program Code Syntax Examples Below are some basic code syntax examples in Java:
- Variable Declaration:
int age = 25;
String name = “John Doe”;
double salary = 55000.50;
- Conditional Statements:
if (age >= 18) {
System.out.println(“You are an adult.”);
} else {
System.out.println(“You are a minor.”);
}
- Looping Constructs:
for (int i = 0; i < 5; i++) {
System.out.println(“Count: ” + i);
}
Conclusion
In conclusion, Java stands as a pillar in programming languages, renowned for its adaptability, reliability, and broad spectrum of applications. As a foundational language, its impact spans various industries, offering many opportunities for developers of all levels.
Java’s unique features, such as platform independence, robustness, and security, set it apart from many other programming languages. Its ability to run on the Java Virtual Machine (JVM) ensures compatibility across different platforms, making it an excellent choice for developing cross-platform applications.
The distinction between Java and Advanced Java lies in their scope and application. While Java covers core programming concepts and syntax, Advanced Java delves deeper into specialized frameworks and advanced topics, catering to the development of complex enterprise-level applications.
Upskilling in Java presents numerous advantages in today’s competitive job market. The high demand for Java developers, combined with its versatility across various domains like web development, mobile applications, enterprise solutions, and scientific computing, offers a vast array of career pathways.
From foundational “Hello World” programs to intricate servlets and beyond, mastering Java opens doors to creative problem-solving and innovation. The language’s ability to handle different programming paradigms and scale to meet diverse requirements solidifies its place as a valuable skill for aspiring and seasoned developers alike.
Whether you’re embarking on your programming journey or seeking to enhance your expertise, the world of Java programming remains an exciting and ever-evolving landscape offering endless opportunities for growth and innovation.
In a constantly evolving technological landscape, Java remains not just a language, but a testament to adaptability, resilience, and the ever-growing potential of the programming world.
Leave a Reply