Exploring Python Patterns: Enhancing Your Code Skills! 🚀
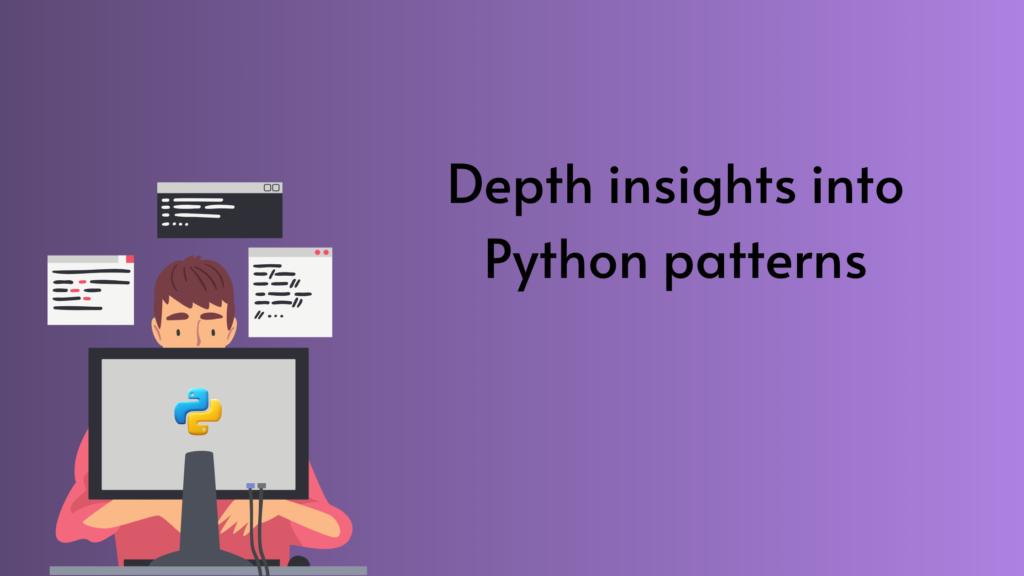
Python, often dubbed as one of the most readable and versatile programming languages, is a treasure trove of patterns and practices that can elevate your coding game. Whether you’re a seasoned developer or just starting your journey in the world of programming, understanding and implementing Python patterns can greatly enhance your code efficiency and maintainability. In this blog, we’ll take a deep dive into Python patterns, complete with examples, to help you level up your coding skills.
Why Python Patterns Matter
Patterns in Python are tried-and-tested solutions to common programming problems. They are essential for several reasons:
- Readability: Python is celebrated for its readability, and patterns make your code even more elegant and understandable.
- Efficiency: Using patterns can optimize your code, making it run faster and use fewer resources.
- Maintainability: Patterns encourage clean, modular code that’s easier to maintain and extend.
- Best Practices: Patterns embody best practices, helping you write code that aligns with industry standards.
Essential Python Patterns
Let’s explore some fundamental Python patterns with practical examples:
- Singleton Pattern: Ensures a class has only one instance, no matter how many times it’s instantiated.
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
return cls._instance
2. Factory Pattern: Centralizes object creation by providing a common interface.
class Dog:
def speak(self):
return “Woof!”
class Cat:
def speak(self):
return “Meow!”
def animal_sound(animal):
return animal.speak()
3. Decorator Pattern: Adds behavior to an object without altering its structure.
def bold_decorator(func):
def wrapper(*args, **kwargs):
return f”<b>{func(*args, **kwargs)}</b>”
return wrapper
@bold_decorator
def greet(name):
return f”Hello, {name}!”
4. Observer Pattern: Defines a one-to-many relationship between objects, allowing one object to notify others of state changes.
class Subject:
def __init__(self):
self._observers = []
def add_observer(self, observer):
self._observers.append(observer)
def notify_observers(self, message):
for observer in self._observers:
observer.update(message)
class Observer:
def update(self, message):
print(f”Received message: {message}”)
5. Strategy Pattern: Defines a family of algorithms, encapsulates each one, and makes them interchangeable.
class PaymentStrategy:
def pay(self, amount):
pass
class CreditCard(PaymentStrategy):
def pay(self, amount):
return f”Paid ${amount} with a credit card.”
class PayPal(PaymentStrategy):
def pay(self, amount):
return f”Paid ${amount} with PayPal.”
In conclusion, Python patterns are an invaluable asset in your journey to becoming a proficient and efficient Python developer. These time-tested solutions not only enhance the readability and maintainability of your code but also optimize its performance. Whether you’re creating a new project or refactoring existing code, Python patterns offer a structured and proven approach to problem-solving.
Throughout this blog, we’ve explored some fundamental Python patterns, complete with practical examples. From the Singleton Pattern, which ensures only one instance of a class, to the Strategy Pattern, allowing for interchangeable algorithms, each pattern serves a unique purpose and can be applied in a wide range of scenarios.
By integrating these patterns into your coding repertoire, you’ll not only write more elegant and structured code but also align with industry best practices. Python’s renowned readability becomes even more pronounced when patterns are intelligently employed.
As you continue your Python programming journey, keep exploring, experimenting, and learning. Stay tuned for more in-depth insights and examples on Python patterns in our upcoming blogs. With these tools in your kit, you’re well on your way to achieving Python mastery. Happy coding and enjoy your Python adventures! 🚀🐍💻
Leave a Reply