Master the Fundamentals of .NET Programming and Learn How to Create .NET Projects
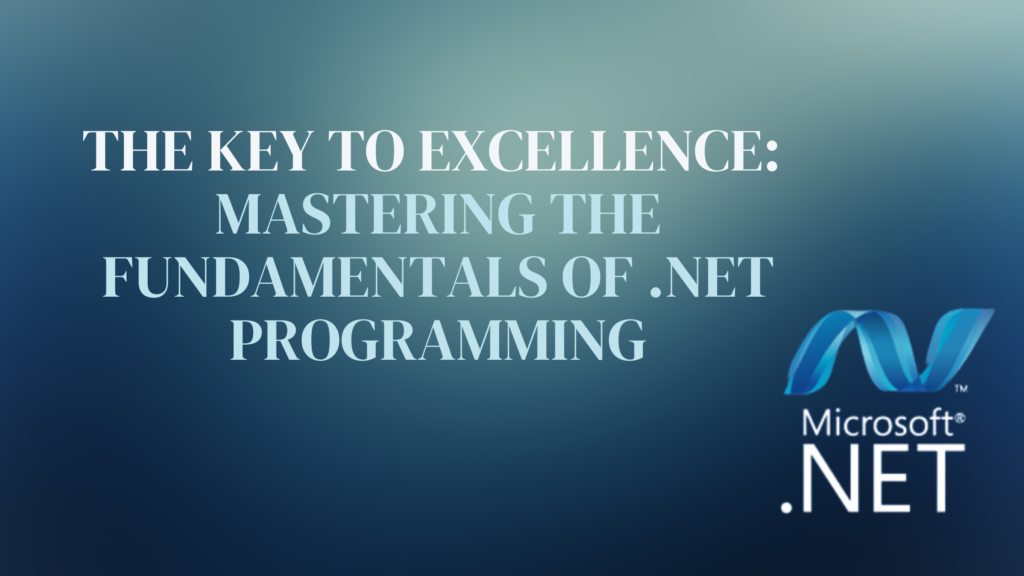
.NET is a powerful, versatile framework developed by Microsoft that allows developers to build a wide range of applications, including web, mobile, desktop, gaming, and cloud-based solutions. Its comprehensive ecosystem, cross-platform compatibility, and support for multiple programming languages make it a top choice for modern developers.
In this blog, we’ll cover the fundamentals of .NET programming and provide a step-by-step guide to creating .NET projects, complete with examples.
What is .NET?
.NET is an open-source developer platform that supports multiple programming languages like C#, F#, and Visual Basic. It provides the tools and libraries required to build high-quality applications.
Key features include:
- Cross-platform compatibility: Build applications for Windows, macOS, Linux, Android, and iOS.
- Unified ecosystem: One platform for different types of applications.
- Robust frameworks: Includes ASP.NET Core for web applications, Xamarin for mobile apps, and more.
- Built-in security: Features like authentication, authorization, and encryption to secure applications.
Fundamentals of .NET Programming
1. Programming Language Basics: C#
C# is the primary language used in .NET. Here are some core concepts:
- Hello World Example:
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine(“Hello, World!”);
}
}
· This basic example introduces the Main
method, the entry point for any .NET application.
· Object-Oriented Programming (OOP):
C# supports OOP principles such as encapsulation, inheritance, and polymorphism. For example:
class Animal
{
public string Name { get; set; }
public virtual void Speak()
{
Console.WriteLine(“Animal speaks”);
}
}
class Dog : Animal
{
public override void Speak()
{
Console.WriteLine(“Dog barks”);
}
}
class Program
{
static void Main(string[] args)
{
Animal myDog = new Dog { Name = “Buddy” };
myDog.Speak(); // Output: Dog barks
}
}
2. ASP.NET Core: Building Web Applications
ASP.NET Core is a high-performance framework for building web applications.
- Creating a Simple Web API:
dotnet new webapi -o MyAPI
cd MyAPI
dotnet run
This creates a RESTful API template. A simple controller might look like this:
using Microsoft.AspNetCore.Mvc;
[ApiController]
[Route(“[controller]”)]
public class WeatherForecastController : ControllerBase
{
[HttpGet]
public IEnumerable<string> Get()
{
return new string[] { “Sunny”, “Cloudy”, “Rainy” };
}
}
- Access the API by navigating to https://localhost:<port>/WeatherForecast.
3. Entity Framework Core: Working with Databases
Entity Framework Core (EF Core) is an ORM (Object-Relational Mapper) for interacting with databases using C#.
- Example: Creating a Model and Database Context:
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
public class AppDbContext : DbContext
{
public DbSet<Product> Products { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder.UseSqlite(“Data Source=products.db”);
}
}
- Adding and Querying Data:
using (var context = new AppDbContext())
{
context.Products.Add(new Product { Name = “Laptop”, Price = 999.99M });
context.SaveChanges();
var products = context.Products.ToList();
products.ForEach(p => Console.WriteLine(p.Name));
}
4. Cross-Platform Development: Xamarin
Use Xamarin to build mobile applications for iOS and Android. A basic Xamarin.Forms app might include:
- XAML for UI Design:
<ContentPage xmlns=”http://xamarin.com/schemas/2014/forms”
xmlns:x=”http://schemas.microsoft.com/winfx/2009/xaml”
x:Class=”MyApp.MainPage”>
<Label Text=”Welcome to Xamarin!”
VerticalOptions=”CenterAndExpand”
HorizontalOptions=”CenterAndExpand” />
</ContentPage>
- C# for Logic:
public partial class MainPage : ContentPage
{
public MainPage()
{
InitializeComponent();
}
}
Creating a .NET Project: A Practical Guide
Step 1: Install Prerequisites
- Download and install the .NET SDK and Visual Studio.
- For mobile apps, install Xamarin extensions.
Step 2: Choose the Application Type
Decide whether to create a web app, desktop app, API, or mobile app. For this example, let’s build a simple To-Do List Web App.
Step 3: Initialize the Project
Run the following command:
dotnet new mvc -o ToDoApp
cd ToDoApp
Step 4: Build the To-Do List App
- Model:
public class ToDoItem
{
public int Id { get; set; }
public string Task { get; set; }
public bool IsComplete { get; set; }
}
- Controller:
public class ToDoController : Controller
{
private static List<ToDoItem> toDoList = new List<ToDoItem>();
public IActionResult Index()
{
return View(toDoList);
}
[HttpPost]
public IActionResult Add(ToDoItem item)
{
toDoList.Add(item);
return RedirectToAction(“Index”);
}
}
- View (Razor):
<form method=”post” asp-action=”Add”>
<input type=”text” name=”Task” placeholder=”Enter a task” required />
<button type=”submit”>Add</button>
</form>
<ul>
@foreach (var item in Model)
{
<li>@item.Task – @item.IsComplete</li>
}
</ul>
Conclusion
.NET is a powerful framework that simplifies building applications for various platforms. By mastering C#, ASP.NET Core, and tools like EF Core and Xamarin, you’ll unlock the potential to create modern, scalable, and high-performing applications.
Start small, build projects, and explore advanced features as you grow. The key is continuous learning and practical implementation. Embrace the power of .NET and bring your ideas to life! 🚀
🌟 Boost Your Career with .NET Training at Ignisys IT! 🌟
Are you ready to master .NET programming and unlock endless career opportunities? Join Ignisys IT for expert-led training on:
✅ C# Fundamentals
✅ ASP.NET Core Development
✅ Entity Framework & Database Integration
✅ Building Real-World .NET Projects
💡 Whether you’re a beginner or a pro looking to upgrade, we’ve got you covered!
📩 Enroll now and take the first step toward becoming a .NET expert.
Leave a Reply