Becoming a React Developer in 2024: A Step-by-Step Guide with strong basic foundation and examples
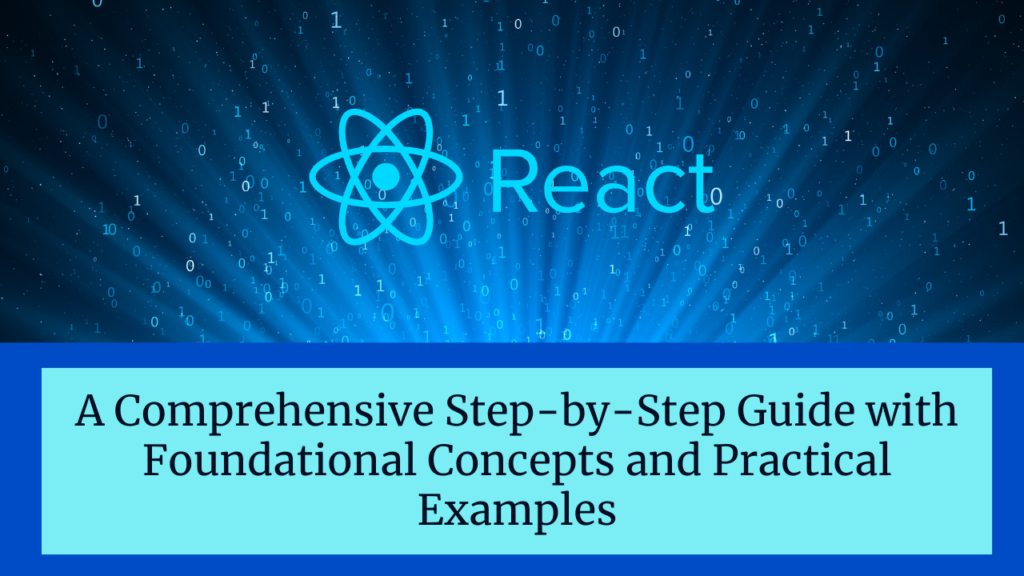
React.js remains one of the most popular libraries for building dynamic and responsive user interfaces. As the demand for React developers continues to grow, especially with the constant evolution of web technologies, mastering React in 2024 is a promising career move. Here’s a comprehensive guide to help you navigate your journey to becoming a proficient React developer.
1. Understand the Basics of HTML, CSS, and JavaScript
Before diving into React, it’s crucial to have a solid understanding of the core web technologies: HTML, CSS, and JavaScript. These languages form the foundation of web development.
- HTML: Learn the structure of web pages.
- CSS: Understand how to style web pages and make them visually appealing.
- JavaScript: Get comfortable with JavaScript fundamentals such as variables, data types, functions, and the DOM.
2. Grasp the Fundamentals of JavaScript ES6+
React heavily relies on modern JavaScript features introduced in ECMAScript 6 and beyond. Familiarize yourself with the following concepts:
- Arrow Functions: Concise syntax for writing functions.
- Destructuring: Extracting values from arrays and objects.
- Spread and Rest Operators: Working with arrays and objects more efficiently.
- Modules: Importing and exporting code between files.
- Promises and Async/Await: Handling asynchronous operations.
3. Learn the Basics of React
Start with the core concepts of React. Understanding these basics is essential:
- JSX: Syntax extension that allows mixing HTML with JavaScript.
- Components: Building blocks of a React application. Learn the difference between functional and class components.
- Props and State: Mechanisms for passing data and managing component data.
- Lifecycle Methods: Understanding component lifecycle events (if using class components).
4. Advanced React Concepts
Once you have a good grasp of the basics, move on to more advanced topics:
- Hooks: Modern way to use state and other React features in functional components.
- useState: Managing state in functional components.
- useEffect: Handling side effects in functional components.
- Custom Hooks: Creating reusable hooks.
- Context API: Managing global state without prop drilling.
- React Router: Handling navigation and routing in a React application.
- Error Boundaries: Handling errors gracefully in React components.
5. State Management
Learn state management techniques for handling more complex state:
- Redux: A popular state management library for React applications.
- MobX: Another state management library with a different approach.
- Recoil: A state management library that works seamlessly with React’s Suspense.
6. Styling React Applications
Explore various ways to style your React components:
- CSS Modules: Scoping CSS to specific components.
- Styled-Components: Writing CSS in JavaScript.
- Emotion: Library for writing CSS styles with JavaScript.
- Sass/SCSS: CSS preprocessor with additional features like variables and nesting.
7. Testing React Applications
Ensure the reliability of your applications by learning to test them:
- Jest: A testing framework for JavaScript.
- React Testing Library: Testing utilities for React components.
- End-to-End Testing: Tools like Cypress for testing the entire application.
8. Build Projects and Portfolio
Put your knowledge into practice by building projects. This will help you reinforce what you’ve learned and create a portfolio to showcase your skills.
- Personal Projects: Create small projects like to-do lists, weather apps, or blogs.
- Contribute to Open Source: Get involved in open-source projects to gain real-world experience.
9. Keep Up with React Ecosystem
React is constantly evolving. Stay updated with the latest trends and best practices:
- Follow React Blog: Official blog for updates and new features.
- Join React Community: Participate in forums, attend meetups, and join social media groups.
- Take Advanced Courses: Enroll in courses that cover new and advanced topics.
10. Apply for Jobs and Internships
With your skills and portfolio ready, start applying for React developer positions:
- Create a Strong Resume: Highlight your projects and skills.
- Prepare for Interviews: Practice common React interview questions and coding challenges.
- Network: Connect with professionals on LinkedIn and attend industry events.
Building a Strong Foundation in React
React, a popular JavaScript library for building user interfaces, is a vital tool in modern web development. Mastering React involves understanding its core concepts, architecture, and best practices. Here’s an in-depth guide to building a strong foundation in React, complete with detailed explanations and practical examples.
1. Understanding React’s Core Concepts
Components
Components are the building blocks of a React application. They allow you to split the UI into independent, reusable pieces.
Example:
import React from ‘react’;
const Greeting = () => {
return <h1>Hello, world!</h1>;
};
export default Greeting;
JSX (JavaScript XML)
JSX is a syntax extension that allows you to write HTML-like code inside JavaScript.
Example:
import React from ‘react’;
const JSXExample = () => {
const name = ‘React’;
return <h1>Hello, {name}!</h1>;
};
export default JSXExample;
Props (Properties)
Props are used to pass data from parent components to child components.
Example:
import React from ‘react’;
const Greeting = (props) => {
return <h1>Hello, {props.name}!</h1>;
};
const App = () => {
return <Greeting name=”React” />;
};
export default App;
State
State is used to manage data that changes over time within a component.
Example:
import React, { useState } from ‘react’;
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
Lifecycle Methods
Lifecycle methods are hooks that allow you to run code at specific points in a component’s lifecycle.
Example:
import React, { Component } from ‘react’;
class LifecycleExample extends Component {
componentDidMount() {
console.log(‘Component did mount’);
}
componentWillUnmount() {
console.log(‘Component will unmount’);
}
render() {
return <div>Check the console for lifecycle messages.</div>;
}
}
export default LifecycleExample;
2. React Hooks
Hooks allow you to use state and other React features without writing a class. The most commonly used hooks are useState
, useEffect
, and useContext
.
useState
useState
is a hook that lets you add React state to function components.
Example:
import React, { useState } from ‘react’;
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
useEffect
useEffect
is a hook that lets you perform side effects in function components. It serves the same purpose as componentDidMount
, componentDidUpdate
, and componentWillUnmount
in React classes.
Example:
import React, { useState, useEffect } from ‘react’;
const DataFetcher = () => {
const [data, setData] = useState([]);
useEffect(() => {
fetch(‘https://jsonplaceholder.typicode.com/posts’)
.then(response => response.json())
.then(data => setData(data));
}, []);
return (
<ul>
{data.map(post => (
<li key={post.id}>{post.title}</li>
))}
</ul>
);
};
export default DataFetcher;
useContext
useContext
is a hook that lets you subscribe to React context without introducing nesting.
Example:
import React, { useContext } from ‘react’;
const ThemeContext = React.createContext(‘light’);
const ThemeDisplay = () => {
const theme = useContext(ThemeContext);
return <div>The current theme is {theme}</div>;
};
const App = () => {
return (
<ThemeContext.Provider value=”dark”>
<ThemeDisplay />
</ThemeContext.Provider>
);
};
export default App;
3. Component Communication
Understanding how to manage component communication is crucial for building complex React applications.
Parent to Child
Data is passed from parent to child components via props.
Example:
import React from ‘react’;
const Child = ({ message }) => {
return <p>{message}</p>;
};
const Parent = () => {
return <Child message=”Hello from parent!” />;
};
export default Parent;
Child to Parent
Data is passed from child to parent components via callback functions.
Example:
import React, { useState } from ‘react’;
const Child = ({ onButtonClick }) => {
return <button onClick={() => onButtonClick(‘Hello from child!’)}>Click me</button>;
};
const Parent = () => {
const [message, setMessage] = useState(”);
return (
<div>
<Child onButtonClick={setMessage} />
<p>{message}</p>
</div>
);
};
export default Parent;
Sibling Communication
Sibling components communicate by lifting the state up to their common parent.
Example:
import React, { useState } from ‘react’;
const Sibling1 = ({ onInputChange }) => {
return <input type=”text” onChange={(e) => onInputChange(e.target.value)} />;
};
const Sibling2 = ({ value }) => {
return <p>{value}</p>;
};
const Parent = () => {
const [inputValue, setInputValue] = useState(”);
return (
<div>
<Sibling1 onInputChange={setInputValue} />
<Sibling2 value={inputValue} />
</div>
);
};
export default Parent;
4. State Management
Managing state is one of the key challenges in React applications. Beyond the built-in state management, several libraries help manage complex state logic.
Redux
Redux is a predictable state container for JavaScript applications. It helps you manage the state of your application in a single place and keep your logic predictable and traceable.
Example:
// src/store.js
import { createStore } from ‘redux’;
const initialState = { count: 0 };
const reducer = (state = initialState, action) => {
switch (action.type) {
case ‘INCREMENT’:
return { …state, count: state.count + 1 };
default:
return state;
}
};
const store = createStore(reducer);
export default store;
// src/Counter.js
import React from ‘react’;
import { useSelector, useDispatch } from ‘react-redux’;
const Counter = () => {
const count = useSelector(state => state.count);
const dispatch = useDispatch();
return (
<div>
<p>Count: {count}</p>
<button onClick={() => dispatch({ type: ‘INCREMENT’ })}>Increment</button>
</div>
);
};
export default Counter;
// src/App.js
import React from ‘react’;
import { Provider } from ‘react-redux’;
import store from ‘./store’;
import Counter from ‘./Counter’;
const App = () => (
<Provider store={store}>
<Counter />
</Provider>
);
export default App;
Context API
React’s Context API is useful for sharing state between components without passing props through every level of the tree.
Example:
import React, { useState, useContext, createContext } from ‘react’;
const CountContext = createContext();
const Counter = () => {
const { count, increment } = useContext(CountContext);
return (
<div>
<p>Count: {count}</p>
<button onClick={increment}>Increment</button>
</div>
);
};
const App = () => {
const [count, setCount] = useState(0);
const increment = () => setCount(count + 1);
return (
<CountContext.Provider value={{ count, increment }}>
<Counter />
</CountContext.Provider>
);
};
export default App;
5. React Router
React Router is a standard library for routing in React. It enables the navigation among views of various components in a React Application, allows changing the browser URL, and keeps the UI in sync with the URL.
Example:
import React from ‘react’;
import { BrowserRouter as Router, Route, Switch, Link } from ‘react-router-dom’;
const Home = () => <h1>Home</h1>;
const About = () => <h1>About</h1>;
const App = () => {
return (
<Router>
<nav>
<ul>
<li>
<Link to=”/”>Home</Link>
</li>
<li>
<Link to=”/about”>About</Link>
</li>
</ul>
</nav>
<Switch>
<Route exact path=”/”>
<Home />
</Route>
<Route path=”/about”>
<About />
</Route>
</Switch>
</Router>
);
};
export default App;
6. Testing
Testing is crucial to ensure your React application works correctly. The most popular tools for testing React applications are Jest and React Testing Library.
Jest
Jest is a JavaScript testing framework designed to ensure the correctness of any JavaScript codebase.
Example:
// src/Greeting.js
import React from ‘react’;
const Greeting = ({ name }) => {
return <h1>Hello, {name}!</h1>;
};
export default Greeting;
// src/Greeting.test.js
import React from ‘react’;
import { render } from ‘@testing-library/react’;
import Greeting from ‘./Greeting’;
test(‘renders greeting message’, () => {
const { getByText } = render(<Greeting name=”React” />);
expect(getByText(‘Hello, React!’)).toBeInTheDocument();
});
React Testing Library
React Testing Library is a lightweight solution for testing React components by querying and interacting with the DOM.
Example:
// src/Counter.js
import React, { useState } from ‘react’;
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
// src/Counter.test.js
import React from ‘react’;
import { render, fireEvent } from ‘@testing-library/react’;
import Counter from ‘./Counter’;
test(‘increments count’, () => {
const { getByText } = render(<Counter />);
const button = getByText(/increment/i);
fireEvent.click(button);
expect(getByText(/count: 1/i)).toBeInTheDocument();
});
Conclusion
Building a strong foundation in React involves mastering its core concepts, hooks, component communication, state management, routing, and testing. By understanding and practicing these fundamental principles, you can develop robust and efficient React applications. Remember to keep your code modular, reusable, and maintainable, and stay updated with the latest React features and best practices.
Joining Ignisys IT for training means gaining access to cutting-edge curriculum, industry-leading instructors, and hands-on learning experiences. Whether you’re looking to kickstart your career in web development, data science, artificial intelligence, cybersecurity, or any other tech field, we have tailored programs to suit your needs.
Whether you’re a beginner looking to break into the tech industry or a seasoned professional seeking to upskill, Ignisys IT is your partner in success. Join us today and embark on a journey toward a brighter future in technology.
Leave a Reply