The Role of JSON in Frontend and Backend Data Exchange
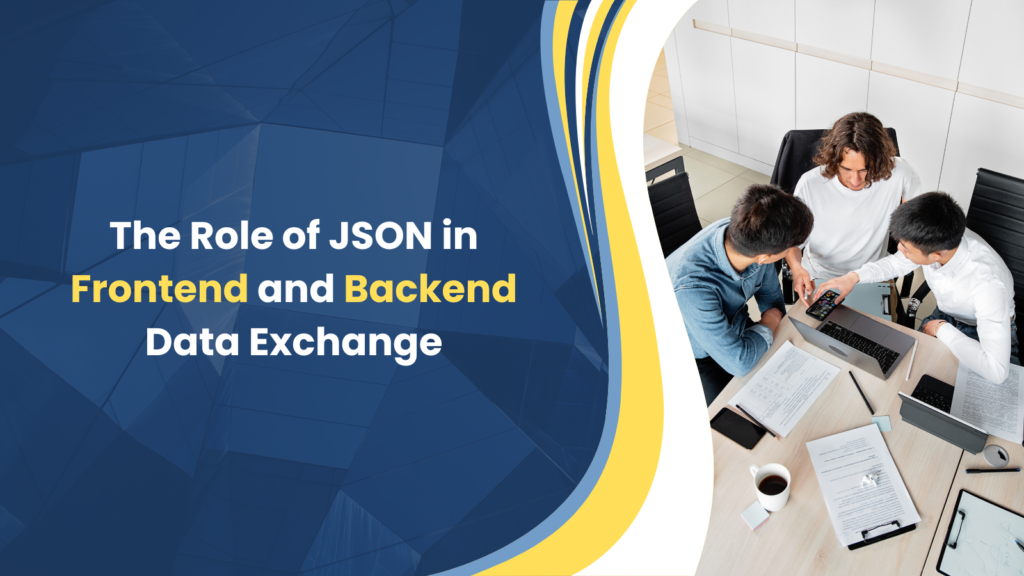
In today’s fast-paced digital landscape, web applications are expected to be responsive, dynamic, and capable of exchanging vast amounts of data seamlessly between the frontend (what users interact with) and the backend (where the business logic resides). At the core of this interaction is JSON (JavaScript Object Notation), a standardized data format that enables the smooth exchange of information between different parts of an application.
Whether it’s sending user input from a form to the server or receiving data from an external API to render on the user’s screen, JSON acts as the bridge that allows different systems to communicate in a language they both understand. Its efficiency and simplicity have cemented JSON as the dominant format for web-based data communication, playing a crucial role in the overall architecture of modern web applications.
What is JSON and Why is It So Popular?
JSON (JavaScript Object Notation) is a lightweight data-interchange format designed to be easy for humans to read and write while still being easy for machines to parse and generate. It was created as a subset of the JavaScript language, but its universal nature means that it’s now used in virtually every programming language.
Key Characteristics of JSON
- Lightweight and Efficient JSON is simple, making it easier to transfer data between systems without adding unnecessary complexity. Its lightweight nature also ensures faster data transmission compared to older formats like XML. By reducing the size of the data sent across the network, JSON minimizes bandwidth consumption and speeds up the data exchange process, which is crucial for modern web applications that require real-time updates and responsiveness.
- Human-Readable JSON’s clean and straightforward syntax is one of its greatest strengths. Even developers who are unfamiliar with JSON can quickly grasp its structure because it is highly intuitive. This readability reduces the time spent debugging and makes it easier to collaborate on code between teams that work on the frontend, backend, and data systems. For example, a simple JSON object like this:
{
“name”: “Alice”,
“age”: 28,
“city”: “London”
}
is easy to understand and process.
· Language-Agnostic While JSON originates from JavaScript, it is not limited to JavaScript-based environments. Virtually every modern programming language, from Python to Ruby, Java, C#, and Go, provides built-in support for parsing and generating JSON data. This cross-language compatibility ensures that developers working in different programming ecosystems can rely on a consistent format for data exchange, fostering integration across diverse technologies.
· Supports Complex Data Structures JSON is flexible enough to represent simple key-value pairs as well as more complex data structures such as arrays, nested objects, and hierarchies. This means it can handle a wide variety of use cases, from representing a list of products in an e-commerce app to modeling complex hierarchical relationships in a content management system. For instance, a JSON structure can model both individual entities and their nested relationships, such as a user profile with multiple associated addresses:
{
“name”: “John Doe”,
“age”: 30,
“addresses”: [
{
“street”: “123 Main St”,
“city”: “New York”
},
{
“street”: “456 Oak St”,
“city”: “Los Angeles”
}
]
}
This ability to represent both flat and nested data makes JSON highly versatile for a wide range of applications.
The Evolution from XML to JSON
Before JSON became the gold standard for web data exchange, XML (Extensible Markup Language) was widely used. While XML is still used in specific scenarios, JSON’s rise has largely been attributed to its comparative simplicity and efficiency. Let’s explore some of the differences between the two:
1. Readability and Simplicity
XML is much more verbose compared to JSON. Take this simple XML structure:
<person>
<name>John</name>
<age>30</age>
<city>New York</city>
</person>
In contrast, the JSON version of this data is shorter and simpler:
{
“name”: “John”,
“age”: 30,
“city”: “New York”
}
The lack of closing tags and extraneous markup makes JSON not only more readable but also more efficient in terms of data size.
2. Ease of Parsing
JSON is easier and faster to parse compared to XML, which typically requires more complex parsing rules due to its nested and sometimes hierarchical structure. In most programming environments, working with JSON data is as simple as calling a function like JSON.parse(), whereas parsing XML often involves more steps to handle its tree-like structure.
3. Smaller Payloads
A JSON object generally results in a smaller payload compared to XML. This size difference is critical when transferring large amounts of data over networks, particularly for applications that need to run in low-bandwidth or high-latency environments. Smaller payloads mean faster transmission and lower data costs, which can be significant in mobile or cloud-based applications where bandwidth consumption matters.
For these reasons, JSON has largely replaced XML in most web applications, and it continues to dominate as the preferred data format for exchanging information.
How JSON Powers Frontend Data Exchange
JSON plays a vital role in frontend development by enabling applications to communicate with servers, fetch data, and update the UI dynamically without requiring a full page reload. Modern web applications, especially single-page applications (SPAs) built using frameworks like React, Angular, or Vue.js, rely heavily on JSON to drive real-time interactivity.
1. Fetching Data from APIs: Fueling Dynamic Web Content
APIs (Application Programming Interfaces) serve as the backbone of modern web applications, providing the mechanism through which frontend applications fetch data from servers or third-party services. JSON is the most commonly used format for these API responses, allowing frontend applications to receive data asynchronously and update the user interface in real-time without the need for a page refresh.
For instance, in a weather app, JSON is used to receive data from a third-party API:
fetch(‘https://api.weatherapi.com/current’)
.then(response => response.json())
.then(data => {
// Process and display the data
console.log(data);
});
In this example, the fetch
API makes an HTTP request to a weather service. The server responds with data in JSON format, which is then parsed and used to update the UI. Without JSON, this type of real-time, asynchronous data interaction would be more complex and less efficient.
2. Rendering Dynamic Content with JSON in JavaScript Frameworks
Frameworks like React, Angular, and Vue.js thrive on the real-time, dynamic exchange of JSON data. These frameworks enable developers to build responsive user interfaces that can update as soon as new data is received. Here’s how JSON might be used in a React application to dynamically display a list of products:
const ProductList = ({ products }) => (
<div>
{products.map(product => (
<Product key={product.id} name={product.name} price={product.price} />
))}
</div>
);
In this example, the products
array, fetched in JSON format, is used to dynamically render each product as a component. This real-time data rendering enhances user experience by making the interface responsive and engaging.
3. Frontend State Management and JSON
State management is an integral part of frontend development, especially in applications that require data consistency and complex user interactions. JSON is commonly used to represent the application’s state, making it easy to structure and manipulate data across components.
Consider a React app managing user data:
const [userData, setUserData] = useState({});
useEffect(() => {
fetch(‘/api/user/123’)
.then(response => response.json())
.then(data => setUserData(data));
}, []);
Here, JSON data fetched from the API is stored in the component’s state (userData
). As the state updates, the UI automatically re-renders to reflect any changes. This data-driven approach is what powers the interactivity and real-time nature of most modern web applications.
4. Storing JSON Data in LocalStorage
Modern browsers provide a convenient way to store data on the client side using LocalStorage and SessionStorage. These storage mechanisms allow developers to persist data across browser sessions, such as user preferences or authentication tokens. JSON is often used to serialize this data before storing it.
For example:
const settings = { theme: ‘dark’, language: ‘en’ };
localStorage.setItem(‘userSettings’, JSON.stringify(settings));
const savedSettings = JSON.parse(localStorage.getItem(‘userSettings’));
console.log(savedSettings.theme); // Output: ‘dark’
By converting the settings object into a JSON string with JSON.stringify(), the data can be safely stored in LocalStorage and easily retrieved later. This use of JSON allows for a simple and effective way to manage persistent client-side data.
JSON in Backend Data Exchange: Handling API Requests and Responses
On the backend, JSON serves as the de facto standard for transferring data between web servers and clients, as well as between various backend services.
1. The Role of JSON in RESTful APIs
In RESTful APIs, which are widely used for web services, JSON is the most common format for sending and receiving data. When the frontend makes an HTTP request to a server, the server processes the request, generates a response (often in JSON format), and sends it back to the client. The frontend then processes the JSON data to update the UI accordingly.
Consider a RESTful API for creating a new user. The client sends a POST request with JSON data in the body:
{
“name”: “Jane Doe”,
“email”: “jane.doe@example.com”
}
On the server side, this data is parsed, processed, and stored. Here’s how an Express.js server might handle this request:
app.post(‘/users’, (req, res) => {
const newUser = req.body; // JSON data from the request body
// Logic to save the user data to the database
res.status(201).json({ message: ‘User created successfully’ });
});
The server responds with a JSON object containing a success message. This straightforward request-response cycle is powered by JSON’s ability to represent and transfer data efficiently between the client and server.
2. Microservices and JSON
In microservices-based architectures, where different services communicate with each other over the network, JSON is often the chosen data format for inter-service communication. Each service can run independently and expose APIs that return data in JSON format, enabling seamless integration across various microservices, even when they are written in different programming languages or running on different platforms.
JSON and NoSQL Databases: Perfect Match
The relationship between JSON and NoSQL databases like MongoDB, CouchDB, and Firestore is another reason for its popularity in the backend. These databases store data in a flexible, document-oriented format, often using BSON (Binary JSON) as their underlying data structure. This compatibility allows developers to store, query, and retrieve data without needing to transform it between formats.
Here’s an example of inserting JSON data into a MongoDB collection:
const product = {
name: ‘Laptop’,
price: 999,
stock: 10
};
db.collection(‘products’).insertOne(product, (err, result) => {
if (err) throw err;
console.log(‘Product inserted:’, result);
});
With this JSON-like data structure, MongoDB can efficiently store and manage the document, making it a natural choice for applications that heavily rely on JSON data.
Advantages of JSON in NoSQL Databases:
- Schema Flexibility: NoSQL databases allow for flexible, schema-less data models, which means JSON objects can evolve over time without the need for strict table structures.
- Scalability: As NoSQL databases are designed to scale horizontally, JSON fits well into this model because of its lightweight and compact nature, reducing overhead in database communication.
JSON Web Tokens (JWT): Securing Data Exchange
One of the most common uses of JSON in the backend is for authentication through JSON Web Tokens (JWT). JWTs are JSON-based tokens used to verify the identity of users or services and provide a stateless, scalable way to manage user sessions.
A typical JWT consists of three parts: Header, Payload, and Signature. The payload, often containing user information or claims, is a JSON object that looks something like this:
{
“sub”: “1234567890”,
“name”: “John Doe”,
“admin”: true
}
This token is signed on the backend and sent to the client, where it can be stored (in cookies or localStorage). The client includes this token in subsequent requests to the server to prove their identity. Here’s an example of verifying a JWT on the backend:
const token = req.headers.authorization.split(‘ ‘)[1];
jwt.verify(token, secretKey, (err, decoded) => {
if (err) return res.status(401).send(‘Unauthorized’);
// Proceed with the request using the decoded token data
});
JWTs enable stateless authentication, meaning that the server does not need to store session data, reducing memory overhead and improving scalability.
Optimizing JSON for Efficient Data Exchange
While JSON is highly efficient, it can still lead to performance bottlenecks in scenarios with large datasets or high-frequency exchanges. As such, optimizing JSON data transfer is essential for creating efficient, high-performance applications.
1. Minification
Minifying JSON before sending it across the network removes whitespace and other unnecessary characters to reduce the size of the payload. For example:
{
“name”: “John”,
“age”: 30,
“city”: “New York”
}
becomes:
{“name”:”John”,”age”:30,”city”:”New York”}
This small change can add up to significant savings in data-heavy applications.
2. Pagination and Lazy Loading
Large datasets can slow down both the client and server when transferred all at once. By implementing pagination and lazy loading, only a subset of data is transferred at a time, reducing the size of each API response and improving overall performance. For instance, instead of sending an entire product catalog, the server could send only 10 products per page, and the client could request additional pages as needed.
3. Compression
Another important optimization is applying gzip or Brotli compression to JSON responses. This can significantly reduce the size of the payload without losing data integrity, leading to faster transmission times and better user experience in low-bandwidth environments.
Conclusion
JSON has become the cornerstone of modern web development, facilitating the seamless exchange of data between frontend and backend systems. Its simplicity, flexibility, and compatibility with various programming languages and technologies make it an ideal choice for web applications of all sizes.
Whether you’re dealing with dynamic content rendering, handling user input in a web form, authenticating users via JWTs, or optimizing API performance, JSON plays a critical role in ensuring the smooth functioning of the application. Understanding how to effectively leverage and optimize JSON is key to building scalable, fast, and efficient web applications in the digital age.
As you continue to work with JSON, remember to focus not only on how it facilitates data exchange but also on how to optimize it for performance and security. With these techniques in hand, you can build applications that are not only functional but also high-performing and user-friendly.
Are you looking to advance your skills and stay competitive in today’s fast-evolving tech landscape? Look no further than IgnisysIT! We provide top-tier training programs designed to empower professionals with the knowledge and hands-on expertise needed to excel in their careers.
Join IgnisysIT and Take the First Step Towards Success
Our mission at IgnisysIT is to empower you with the tools and knowledge you need to succeed in the fast-paced world of technology. Whether you’re aiming for career growth, a new job opportunity, or simply want to sharpen your skills, IgnisysIT is the partner you need on your journey.
Contact us today to learn more about our upcoming training sessions and how we can help you achieve your career goals!
Leave a Reply