Unlocking the Power of C#: A Modern, Object-Oriented Programming Journey
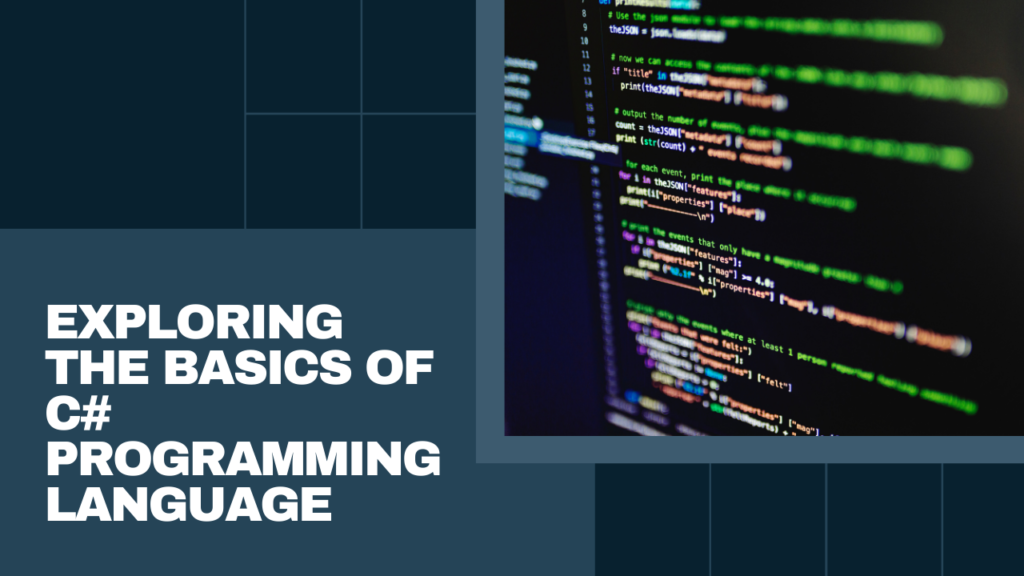
What is C#?
C# (pronounced “C Sharp”) is a modern, object-oriented programming language developed by Microsoft. It is part of the .NET platform and is designed for developing applications for both hosted and embedded systems.
Why Choose C#?
C# offers several advantages for developers:
- Object-Oriented: C# is a fully object-oriented programming language, making it easier to structure and organize software.
- Type-Safe: The C# language is type-safe, which means that the compiler validates types while compiling, minimizing bugs and errors in the code.
- Interoperability: C# can interact with many other languages and platforms, thanks to the .NET framework.
Getting Started with C#
To start coding in C#, you’ll need an Integrated Development Environment (IDE). Visual Studio, a popular IDE from Microsoft, is a great choice for C# development.
Here’s a simple “Hello, World!” program in C#:
using System;
class Program
{
static void Main()
{
Console.WriteLine(“Hello, World!”);
}
}
Key Concepts in C#
Variables and Data Types
C# includes several built-in data types. Here are a few examples:
int myInt = 5;
double myDouble = 5.5;
bool myBool = true;
string myString = “Hello, World!”;
Control Structures
C# includes several control structures including if
, switch
, for
, while
, and do-while
. Here’s an example of an if
statement:
int x = 10;
if (x > 5)
{
Console.WriteLine(“x is greater than 5”);
}
Exploring Classes, Inheritance, and Polymorphism
Classes and Objects
In C#, a class is a blueprint for creating objects (a particular data structure). A class defines what an object will consist of and what operations can be performed on it. Here’s an example of a class in C#:
public class Car
{
public string color;
public int year;
public void Drive()
{
Console.WriteLine(“The car is driving”);
}
}
Inheritance
Inheritance is a fundamental principle of object-oriented programming where a class (child class) inherits the members (fields, methods, etc.) of another class (parent class). Here’s an example:
public class Vehicle
{
public string brand = “Ford”;
public void Honk()
{
Console.WriteLine(“Tuut, tuut!”);
}
}
public class Car : Vehicle
{
public string modelName = “Mustang”;
}
In this example, Car
is the derived class and Vehicle
is the base class. The Car
class inherits the members of Vehicle
.
Polymorphism
Polymorphism is another fundamental principle of object-oriented programming. It allows methods to act differently based on the object that is calling it. Here’s an example:
public class Animal
{
public virtual void animalSound()
{
Console.WriteLine(“The animal makes a sound”);
}
}
public class Pig : Animal
{
public override void animalSound()
{
Console.WriteLine(“The pig says: wee wee”);
}
}
public class Dog : Animal
{
public override void animalSound()
{
Console.WriteLine(“The dog says: bow wow”);
}
}
In this example, animalSound
is a polymorphic method. The output depends on whether it’s called by an object of the Pig
class or of the Dog
class.
C# Advanced: Exploring Interfaces, Delegates, and Events
Interfaces
An interface in C# is a blueprint of a class. It’s a completely ‘abstract class’, which can have only abstract methods and properties etc.
Here’s an example of an interface:
public interface IAnimal
{
void animalSound(); // interface method (does not have a body)
}
A class or struct that implements the interface must implement the members of the interface that are declared in the interface definition. Here’s an example:
public class Pig : IAnimal
{
public void animalSound()
{
Console.WriteLine(“The pig says: wee wee”);
}
}
Delegates
A delegate in C# is similar to a function pointer in C or C++. Using a delegate allows the programmer to encapsulate a reference to a method inside a delegate object. The delegate object can then be passed to code which can call the referenced method, without having to know at compile time which method will be invoked.
Here’s an example of a delegate:
public delegate void MyDelegate(string msg); // declare a delegate
// set target method
public void ShowMessage(string message)
{
Console.WriteLine(“ShowMessage: ” + message);
}
// target method
MyDelegate del = ShowMessage;
del(“Hello World!”);
Events
Events enable a class or object to notify other classes or objects when something of interest occurs. The class that sends (or raises) the event is called the publisher and the classes that receive (or handle) the event are called subscribers.
Here’s an example of an event:
public class EventExample
{
public event Action<string> OnChange = delegate { };
public void RaiseEvent()
{
OnChange(“Event raised”);
}
}
public class Program
{
static void Main(string[] args)
{
EventExample obj = new EventExample();
obj.OnChange += (message) => Console.WriteLine(message);
obj.RaiseEvent();
}
}
These are some of the advanced features of C#. As you continue to explore C#, you’ll find that it’s a robust and versatile language for all kinds of applications.
Common commands (statements) used in C# programming along with examples:
1. Declaration and Initialization
In C#, you declare a variable with a specific data type and use the assignment operator =
to assign a value to the variable.
int num = 10;
string text = “Hello, World!”;
2. Conditional Statements
if-else
statements are used to perform different actions based on different conditions.
int num = 10;
if (num > 5)
{
Console.WriteLine(“Number is greater than 5”);
}
else
{
Console.WriteLine(“Number is less than or equal to 5”);
}
3. Loops
for
loop is used when you know how many times you want to loop.
for (int i = 0; i < 5; i++)
{
Console.WriteLine(i);
}
while
loop is used when you want to loop until a specific condition is met.
int i = 0;
while (i < 5)
{
Console.WriteLine(i);
i++;
}
4. Arrays
An array is used to store multiple values in a single variable.
int[] numbers = {1, 2, 3, 4, 5};
5. Functions
Functions are blocks of code that perform a specific task. A function is defined with the void
keyword for functions that don’t return a value. For functions that do return a value, you replace void
with the return type.
void SayHello()
{
Console.WriteLine(“Hello, World!”);
}
int AddNumbers(int a, int b)
{
return a + b;
}
These are just a few examples of the commands used in C#. There are many more commands and concepts to explore as you continue learning C#.
Conclusion: As we wrap up our exploration of C#, it’s evident that this modern, object-oriented programming language is more than just a tool—it’s a gateway to innovation and creativity. With its intuitive syntax and powerful features, C# empowers developers to bring their ideas to life, whether they’re crafting simple applications or architecting complex systems.
From mastering the fundamentals to delving into advanced topics like inheritance, polymorphism, and event handling, the journey of learning C# is as rewarding as it is enlightening. As you continue to hone your skills and expand your knowledge, you’ll discover new ways to leverage C# to solve real-world problems and drive positive change.
So, whether you’re a seasoned developer looking to stay ahead of the curve or a newcomer eager to dive into the exciting world of programming, C# offers endless opportunities for growth and exploration. Embrace the challenge, embrace the possibilities, and let C# be your guide on the path to success in the ever-evolving landscape of technology. The adventure awaits—let’s code! 🌟
At IgnisysIT, we’re dedicated to nurturing the next generation of tech talent through our comprehensive C# course. Our expert instructors bring years of industry experience to the classroom, ensuring that you receive top-notch training that’s both practical and relevant.
Join IgnisysIT today and embark on a transformative journey to becoming a C# expert. Your future in tech starts here!
Leave a Reply