Understanding Loops in Programming: Types, Importance, and Usage in Java
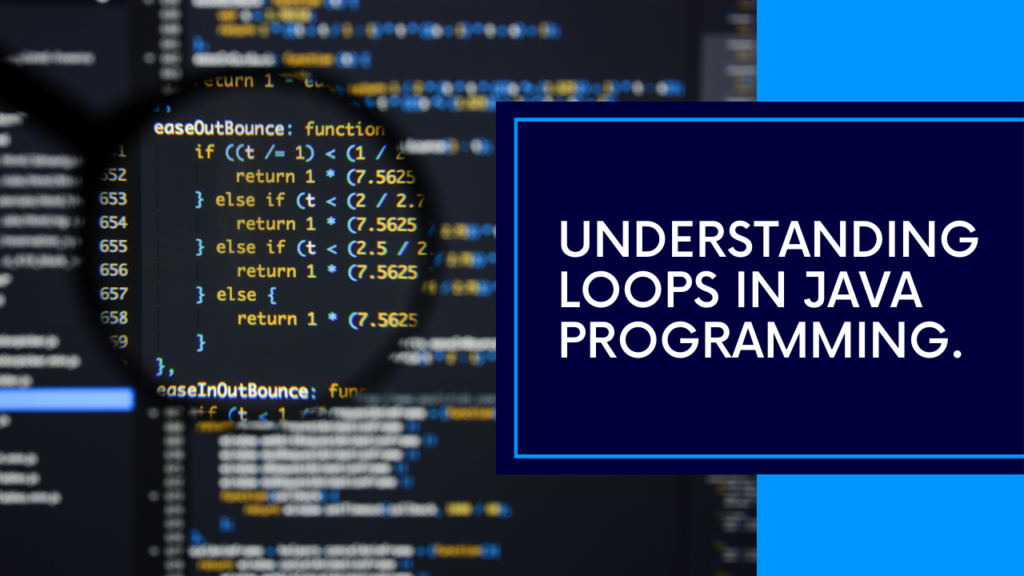
What is a Loop?
In programming, loops are iterative structures that enable the execution of a block of code repeatedly until a certain condition is met. They offer an efficient way to automate repetitive tasks within a program, making code execution more streamlined and manageable.
Importance of Loops
Loops are essential in programming due to several key reasons:
- Efficiency: They allow tasks to be performed repeatedly without the need for redundant code.
- Readability: By compactly expressing repetitive actions, loops enhance the readability and maintainability of code.
- Flexibility: Loops offer adaptability to dynamic data and conditions, enabling iteration based on changing variables.
Types of Loops in Java
Java provides three primary types of loops:
- For Loop
- Structure: for(initialization; condition; update) { }
- Explanation: The for loop allows initializing a variable, specifying a condition for execution, and defining an increment/decrement operation.
- Usage: Ideal for iterating over sequences for a fixed number of times.
Example:
for (int i = 0; i < 5; i++) {
System.out.println(“Iteration: ” + i);
}
While Loop
- Structure: while(condition) { }
- Explanation: While loops execute a block of code as long as a specified condition evaluates to true.
- Usage: Effective for condition-based iteration until the condition becomes false.
Example:
int count = 0;
while (count < 5) {
System.out.println(“Count: ” + count);
count++;
}
Do-While Loop
- Structure: do { } while(condition);
- Explanation: This loop executes the code block once before checking the condition. It guarantees at least one execution.
- Usage: Ensures execution at least once regardless of the condition.
Example:
int x = 5;
do {
System.out.println(“Value of x: ” + x);
x–;
} while (x > 0);
Difference Between For Loop, While Loop, and Do-While Loop
Loop Type | Structure | Execution | Usage |
For Loop | for(init; cond; update) { } | Checks condition before execution. | Ideal for fixed iterations. |
While Loop | while(condition) { } | Checks condition before execution. | Suitable for condition-based iteration. |
Do-While Loop | do { } while(condition); | Executes code once before checking condition. | Ensures execution at least once. |
Types of For Loops in Java
- Traditional For Loop
- Syntax: for(initialization; condition; update) { }
- Example:
for (int i = 0; i < 5; i++) {
System.out.println(“Value of i: ” + i);
}
Enhanced For Loop (For-Each)
- Syntax: for(datatype variable : collection) { }
- Example:
int[] numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
System.out.println(“Number: ” + num);
}
For Loop with a Labeled Break
- Syntax: labelName: for(initialization; condition; update) { }
- Example:
outerLoop: for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (i == 1 && j == 1) {
break outerLoop;
}
System.out.println(“i: ” + i + “, j: ” + j);
}
}
Conclusion
Understanding loops in Java is fundamental for efficient and concise programming. By utilizing the appropriate loop types and mastering their syntax, programmers can significantly enhance code readability, efficiency, and flexibility. Loops are powerful tools that simplify complex tasks and contribute to well-structured and optimized programs.