Exploring Functions in C: The Backbone of C Programming
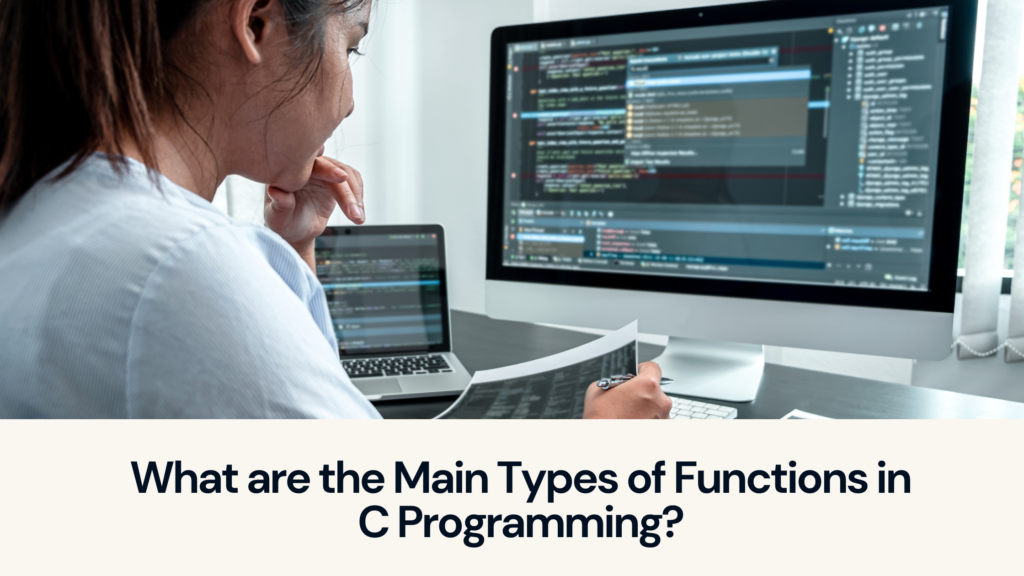
In C programming, functions serve as the foundational elements of any C program. Essentially, a function comprises a set of statements enclosed within curly brackets ({}) that accept inputs, perform computations, and yield outputs. The beauty of functions lies in their ability to be invoked multiple times, fostering reusability and modularity in C programming. Instead of duplicating code for different arguments, functions allow us to encapsulate the code and call it repeatedly by simply passing various arguments.
Why are Functions Essential in C Programming?
Functions are indispensable in C programming, as well as in other programming languages, owing to the manifold advantages they offer to developers. Some key benefits of leveraging functions include:
- Enabling Reusability and Reducing Redundancy: Functions facilitate the reuse of code across different parts of a program, thereby minimizing redundancy and enhancing efficiency.
- Promoting Modularity: By breaking down a program into smaller, self-contained functions, code becomes modular, facilitating easier debugging, testing, and maintenance.
- Providing Abstraction Functionality: Functions abstract away complex operations, allowing developers to focus on high-level functionality without being burdened by implementation details.
- Enhancing Program Understandability and Manageability: Organizing code into functions makes programs more readable, understandable, and manageable, even as complexity increases.
- Facilitating Program Decomposition: Functions empower developers to decompose extensive programs into smaller, more manageable pieces, thereby simplifying development and enhancing scalability.
In essence, functions not only enhance code organization and efficiency but also contribute significantly to the overall clarity and maintainability of C programs, making them an indispensable tool in the developer’s toolkit.
Understanding the Basic Syntax of Functions
In C programming, the syntax for defining functions follows a standard structure:
return_type function_name(arg1, arg2, … argn) {
// Body of the function – Statements to be processed
}
Breaking down the syntax:
- return_type: Specifies the data type of the value returned by the function. However, if a function does not return any value, the keyword
void
indicates to the compiler that no value will be returned. - function_name: This is the unique identifier for the function, aiding the compiler in identifying and executing it whenever it’s called within the program.
- arg1, arg2, …argn: Represents the list of arguments or parameters to be passed into the function. These parameters define the data type, sequence, and number of inputs expected by the function. The parameter list is optional, as a function may not necessarily require parameters.
- Body: Contains the actual statements to be executed whenever the function is invoked. This constitutes the core functionality of the function, performing computations, and generating outputs as needed.
It’s worth noting that the combination of the function’s name and its parameter list is collectively referred to as the signature of the function in C programming. This signature uniquely identifies the function and its expected inputs, facilitating its usage and integration within the program.
In C programming, functions serve as the foundational elements upon which a C program is constructed. Each function encapsulates a series of statements enclosed within curly brackets ({}) that are responsible for receiving inputs, performing computations, and producing output. One of the key advantages of functions is their ability to be invoked multiple times, facilitating reusability and modularity within C programming. This means that instead of duplicating identical code for different arguments, developers can consolidate the code into a function and then invoke it as needed with various arguments. In essence, functions streamline the development process by promoting code efficiency and enhancing program organization.
What is the significance of functions in C programming?
Functions are indispensable in C programming, as well as in other programming languages, owing to the multitude of advantages they offer to developers. Some of the primary benefits of incorporating functions into code include:
- Facilitating reusability and minimizing redundancy
- Promoting modularity in code structure
- Providing abstraction, thus simplifying complex operations
- Enhancing program comprehension and management
- Breaking down large programs into more manageable components
Now, let’s delve into the fundamental syntax of functions in C programming.
Certainly! Here are examples demonstrating the basic syntax and use of functions in C programming:
- Example of a Function with Return Value and Parameters:
#include <stdio.h>
// Function declaration
int add(int a, int b) {
return a + b;
}
int main() {
int result;
// Function call
result = add(5, 3);
printf(“The sum is: %d\n”, result);
return 0;
}
Output:
The sum is: 8
2. Example of a Function with No Return Value and Parameters:
#include <stdio.h>
// Function declaration
void greet(char name[]) {
printf(“Hello, %s!\n”, name);
}
int main() {
// Function call
greet(“Alice”);
greet(“Bob”);
return 0;
}
Output:
Hello, Alice!
Hello, Bob!
- Example of a Function with Return Value and No Parameters:
#include <stdio.h>
// Function declaration
int getRandomNumber() {
return rand() % 100; // Generate a random number between 0 and 99
}
int main() {
int randomNumber;
// Function call
randomNumber = getRandomNumber();
printf(“Random number: %d\n”, randomNumber);
return 0;
}
Output (may vary):
Random number: 42
These examples demonstrate how functions can be defined, called, and utilized within a C program to perform various tasks, showcasing the versatility and efficiency they bring to software development.
Understanding the Aspects of Functions in C Programming
Functions in C programming encompass three key aspects: declaration, definition, and calling. Let’s explore each aspect in detail:
- Function Declaration: Function declaration informs the compiler about the function’s name, the number and data types of its parameters, and its return type. While specifying parameter names during declaration is optional, it’s permissible to do so while defining the function.
Example:
// Function declaration
int add(int, int);
// Function definition
int add(int num1, int num2) {
return num1 + num2;
}
- Function Call: A function call involves invoking a function to be executed by the compiler. Functions can be called at any point within the program. It’s essential to pass arguments of the same data type as specified during declaration. If the function’s parameters match, the compiler executes the program and provides the return value.
Example:
#include <stdio.h>
// Function declaration
int add(int, int);
int main() {
int result;
// Function call
result = add(5, 3);
printf(“Result: %d\n”, result); // Output: Result: 8
return 0;
}
// Function definition
int add(int num1, int num2) {
return num1 + num2;
}
- Function Definition: Function definition entails specifying the actual statements that the compiler will execute upon calling the function. It essentially constitutes the body of the function. A function definition must return a single value at the conclusion of its execution.
Example:
#include <stdio.h>
// Function declaration
void greet();
int main() {
// Function call
greet(); // Output: Hello, World!
return 0;
}
// Function definition
void greet() {
printf(“Hello, World!\n”);
}
These examples illustrate the essential aspects of functions in C programming, showcasing their declaration, definition, and calling mechanisms.
Top of Form
Types of Functions in C Programming
Functions in C programming are typically categorized into two main types:
- Library Functions: Library functions, also known as predefined functions, are already implemented in the C standard libraries. These functions cover a wide range of functionalities, including input/output operations, mathematical computations, string manipulations, and more. Developers can readily use these functions without the need to define or implement them explicitly. However, it’s crucial to include the appropriate header files at the beginning of the program to access these functions. Examples of library functions include printf(), scanf(), ceil(), and floor().
- User-Defined Functions: User-defined functions are created by developers to fulfill specific requirements within a program. These functions are declared, defined, and invoked by the user as needed. They offer flexibility, extendibility, and enhanced reusability to C programming, allowing developers to tailor functions to suit their unique needs. A significant advantage of C programming is the ability to add user-defined functions to any library for use in other programs.
Header Files for Library Functions in C Programming
Library functions are organized into different header files, each serving a specific purpose. Including the appropriate header files at the beginning of the program is essential for accessing the corresponding library functions. Here are some commonly used header files in C programming along with their descriptions:
- stdio.h: Input/output operations
- conio.h: Console input/output operations
- string.h: String manipulation functions
- stdlib.h: General-purpose functions
- math.h: Mathematical functions
- time.h: Time-related functions
- ctype.h: Character handling functions
- stdarg.h: Variable argument functions
- signal.h: Signal handling functions
- setjmp.h: Jump functions
- locale.h: Locale-related functions
- errno.h: Error handling functions
- assert.h: Diagnostic functions
These header files provide access to a wide array of functions, simplifying the development process and enhancing the functionality of C programs.
Diverse Approaches to Function Invocation in C Programming
In C programming, the manner in which functions are called varies based on whether they accept arguments or return values. Let’s explore the four distinct scenarios:
- Functions Without Arguments and Return Value: These functions neither accept any arguments nor return any values. Below are examples demonstrating such functions along with their respective outputs.
- Functions With No Arguments But Returning Values: These functions return values without accepting any arguments. Here’s an example showcasing the computation and return of the area of a rectangle without requiring any arguments.
- Functions With Arguments But No Return Value: These functions accept arguments but do not return any values. The provided example illustrates such a function along with its output.
- Functions Accepting Arguments and Returning Values: Most functions in C accept arguments and return values. A sample program below demonstrates a C function that takes arguments and returns a value, along with its output.
Further Insights into Functions in C Programming:
- Every C program includes at least one function, typically the main function, serving as the program’s entry point where execution commences.
- Even if a function doesn’t return a value, it possesses a return type. If a value is returned, the return type corresponds to the data type of the value; otherwise, it defaults to void.
- C functions are incapable of returning array and function types, though this limitation can be circumvented using pointers.
- In C programming, void func() and void func(void) exhibit different behavior. Unlike in C++, where they are equivalent, in C, a function declared without any parameter list can be called with any number of parameters. Therefore, it’s advisable to declare a function as void func(void) if no parameters are intended.
- Calling a function prior to its declaration leads the C compiler to assume the return type to be int by default. Any discrepancies in the return type result in compilation errors, except for int.
These insights illuminate various nuances and considerations pertaining to function usage in C programming, ensuring effective utilization and robust program design.
Key Functions in C Programming
One fundamental function in C programming that you’ve likely encountered in every example is the main()
function. Indeed, in virtually all C programs, the main()
function plays a pivotal role as the entry point where program execution commences. By default, the return type of main()
is int. Notably, main()
functions can exist in two forms: with or without parameters.
Recursive Functions in C Programming
Recursive functions in C programming possess the ability to call themselves until a specified exit condition is met. This recursive behavior enables iterative processing within a function, allowing for elegant solutions to certain problems. For instance, consider calculating the factorial of a number using recursion.
Inline Functions in C Programming
In C programming, the execution flow typically jumps to the function definition after a function call, necessitating additional processing overhead. However, this overhead can be minimized through the use of inline functions. Inline functions replace the function call with the actual program code, eliminating the need for the compiler to jump back and forth between function calls and definitions. By prefixing a function with the inline
keyword, you can declare it as an inline function. These functions are particularly useful for optimizing small computations.
In summary, functions serve as the cornerstone of C programming, offering reusability, modularity, and simplicity to program design. Understanding how to create and leverage functions is essential for optimizing C programs.
However, in today’s competitive landscape, proficiency in C programming alone may not suffice. To stay ahead, developers are continually enhancing their skills by mastering multiple programming languages and tools. Consider enrolling in our Full-Stack Web Development Course at IgnisysIT, where you’ll gain comprehensive knowledge of various programming languages and practical experience with popular tools. Upon completion, you’ll be well-equipped to secure lucrative positions in multinational companies.