Git Tutorial for Beginners
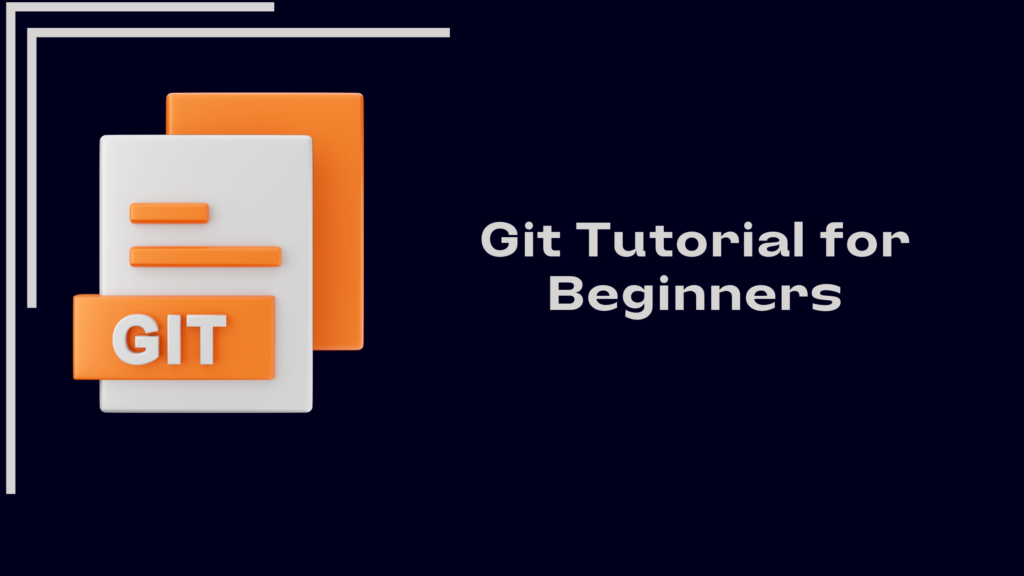
What is Git?
Git is a distributed version control system (DVCS) designed to handle everything from small to very large projects quickly and efficiently. It tracks changes in the source code during software development, allowing multiple developers to work on the same project simultaneously without conflicts. Git’s distributed architecture means that every developer has a full history of the project locally, making it a robust and reliable tool for managing code.
Key Characteristics of Git
- Distributed Version Control:
- Every developer has a full copy of the project repository, including the entire history of changes. This means that the repository is not reliant on a central server, and developers can work offline and sync changes once they reconnect.
- Branching and Merging:
- Git makes it easy to create, manage, and merge branches. Branching allows you to work on different features or fixes independently. Merging integrates these branches back into the main codebase, preserving the history and facilitating collaborative development.
- Efficiency and Speed:
- Git is optimized for performance, allowing it to handle large projects and repositories with ease. Operations such as commits, branching, and merging are designed to be fast, even for large codebases.
- Data Integrity:
- Git uses a cryptographic method (SHA-1 hashing) to ensure the integrity of the data. Every file and commit is checksummed, and Git uses these checksums to ensure that your data remains unchanged and secure.
- Snapshots, Not Differences:
- Unlike other version control systems that store changes as file differences (deltas), Git takes snapshots of the project files. When you commit changes, Git creates a snapshot of the current state of the project and stores a reference to that snapshot.
- Collaboration and Workflow:
- Git supports collaborative workflows, allowing multiple developers to work on the same project simultaneously. Changes can be shared, reviewed, and integrated efficiently, enhancing team productivity.
Benefits of Using Git
- Version Control:
- Git keeps a detailed history of changes, making it easy to track modifications, revert to previous versions, and understand the evolution of the project.
- Collaboration:
- Multiple developers can work on the same project without overwriting each other’s work. Git facilitates code reviews, branch management, and merging, making teamwork more efficient.
- Backup and Recovery:
- With every developer having a full copy of the repository, Git provides built-in redundancy. This ensures that data is not lost and can be recovered easily in case of failures.
- Flexibility:
- Git supports various workflows and branching strategies, allowing teams to choose the approach that best suits their development style.
- Integration:
- Git integrates with many tools and services, such as GitHub, GitLab, Bitbucket, and continuous integration/continuous deployment (CI/CD) pipelines, enhancing its capabilities and making it a central part of the development ecosystem.
Basic Git Terminology
- Repository (Repo): A storage location for your project’s files and their history. It can be local (on your computer) or remote (hosted on a platform like GitHub).
- Commit: A snapshot of changes made to the files in the repository. Each commit has a unique identifier and message describing the changes.
- Branch: A separate line of development. Branches allow you to work on different features or fixes without affecting the main codebase.
- Merge: The process of integrating changes from one branch into another.
- Clone: A copy of an existing Git repository. When you clone a repository, you get the full history and all branches of the project.
- Pull: Fetching changes from a remote repository and merging them into your local branch.
- Push: Sending your local commits to a remote repository.
Git Workflow
A typical Git workflow involves a series of steps to manage changes to the source code:
- Clone the Repository:
git clone <repository-url>
This command copies a remote repository to your local machine.
- Create a New Branch:
git checkout -b <branch-name>
Creating a branch allows you to work on new features or fixes without affecting the main codebase.
- Make Changes and Stage Them:
git add <file>
This command stages your changes, preparing them for a commit.
- Commit the Changes:
git commit -m “commit message”
Commits your changes to the local repository with a descriptive message.
- Push the Changes:
git push origin <branch-name>
Pushes your changes to the remote repository.
- Create a Pull Request:
Once your changes are pushed, create a pull request to merge your branch into the main branch. This step usually involves code review and testing.
Step-by-Step Guide to Install Git on Windows
1. Download Git:
- Visit the official Git website: Git for Windows
- Click “Download” to get the latest version.
2. Run the Installer:
- Open the downloaded
.exe
file. - Follow the installation wizard:
- Choose the default settings unless you have specific requirements.
- Select your preferred editor for Git (e.g., Vim, Notepad++, etc.).
- Adjust your PATH environment (default option recommended).
- Choose HTTPS transport backend (OpenSSL recommended).
- Configure the line ending conversions (default option recommended).
3. Verify Installation:
- Open Command Prompt or Git Bash.
- Type
git --version
and press Enter. You should see the installed Git version.
What are Git Commands?
Git commands are the core of interacting with the Git system. Here are some essential Git commands with examples:
· Initialize a Repository
git init
This command creates a new Git repository in your current directory.
· Clone a Repository
git clone https://github.com/user/repo.git
This command copies an existing Git repository to your local machine.
· Add Files to Staging Area
This command stages a specific file. You can use git add .
to stage all changed files.
· Commit Changes
This command commits the staged changes to the repository with a message describing what was changed.
· Push Changes to Remote Repository
git push origin main
This command uploads your local commits to the remote repository.
· Pull Changes from Remote Repository
git pull origin main
This command fetches and merges changes from the remote repository to your local repository.
· Create a New Branch
git checkout -b feature-branch
This command creates a new branch and switches to it.
· Merge Branches
git checkout main
git merge feature-branch
This command merges changes from feature-branch
into the main
branch.
Difference Between Git and GitHub
While Git is a version control system, GitHub is a platform for hosting Git repositories. Here are the main differences:
Feature | Git | GitHub |
Definition | A version control system to manage source code history. | A web-based platform to host Git repositories. |
Usage | Local version control on a developer’s machine. | Remote repositories for collaborative development. |
Functionality | Tracks changes, manages branches, and merges code. | Provides a web interface, issue tracking, CI/CD. |
Collaboration | Command-line tool for local operations. | Web-based interface for team collaboration. |
Integration | Standalone tool. | Integrates with various development tools and services. |
What is a Git Repository? How to Create It?
A Git repository is a storage location for your project’s files and the entire history of their changes. It can be local or remote.
How to Create a Git Repository
1. Initialize a Repository:
git init
This command initializes a new Git repository in the current directory.
2. Add Files:
git add .
This command stages all files in the current directory for the initial commit.
3. Commit Files:
git commit -m “Initial commit”
This command links your local repository to a remote GitHub repository.
5. Push to the Remote Repository:
git push -u origin main
This command pushes your commits to the remote repository and sets the remote branch as the default upstream branch.
Conclusion
Git is an indispensable tool for modern software development, offering a robust and efficient system for version control. Its distributed nature allows developers to work offline and sync changes later, ensuring that the project history is always preserved and available to every team member. Git’s branching and merging capabilities make it easy to develop new features and fix bugs without disrupting the main codebase, and its speed and efficiency ensure smooth operations even with large projects.
This detailed guide provides a comprehensive overview of Git, making it accessible for beginners and useful for more experienced developers looking to refresh their knowledge.
🌟 Join IgnisysIT for Top-Notch Training Programs! 🌟
🚀 Are you looking to enhance your skills and stay ahead in the competitive tech industry? Look no further! IgnisysIT offers comprehensive training programs designed to help you achieve your career goals.