Mastering DevOps and Git: Top Interview Questions and Expert Answers
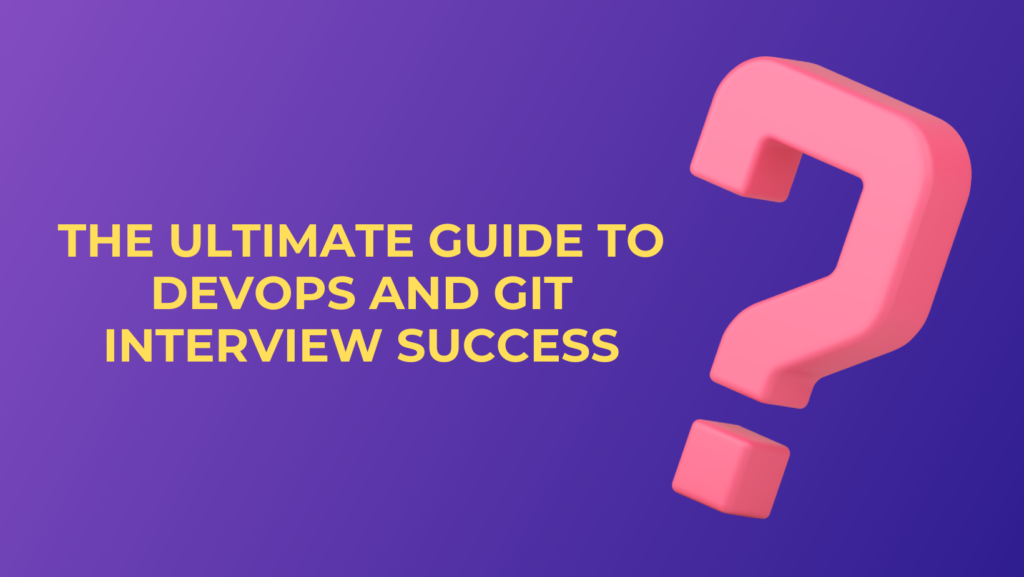
Preparing for a DevOps interview requires a strong understanding of the fundamental concepts, tools, and processes that drive DevOps practices. Below is a list of commonly asked DevOps interview questions, along with detailed answers and examples to help you succeed.
1. What is DevOps? How does it benefit an organization?
Answer:
DevOps is a culture and set of practices that promote collaboration between development and operations teams to automate and streamline the software development and deployment process.
Benefits:
- Faster Delivery: Enables rapid deployment of applications.
- Improved Collaboration: Breaks down silos between teams.
- Enhanced Quality: Automates testing and continuous integration, reducing errors.
Example:
A company using traditional methods takes weeks to release a software update. By adopting DevOps practices like CI/CD pipelines with tools like Jenkins, the same company can deploy updates within hours.
2. What is CI/CD? Why is it important in DevOps?
Answer:
CI/CD stands for Continuous Integration and Continuous Deployment/Delivery. It is a process where code changes are automatically built, tested, and deployed to production.
Importance:
- Reduces manual errors.
- Speeds up software release cycles.
- Ensures continuous feedback and improvement.
Example:
A developer pushes code changes to a Git repository. The CI/CD pipeline automatically triggers:
- Build: Compiles the code using Maven.
- Test: Runs automated tests via Selenium.
- Deploy: If successful, deploys the application to a staging environment using Kubernetes.
3. What are some popular DevOps tools you have used?
Answer:
Here are categories of tools with examples:
- Version Control: Git (GitHub, GitLab, Bitbucket).
- CI/CD: Jenkins, GitLab CI, CircleCI.
- Configuration Management: Ansible, Puppet, Chef.
- Containerization: Docker.
- Orchestration: Kubernetes.
- Monitoring: Prometheus, Nagios, Splunk.
Example Scenario:
For a microservices-based application, Docker is used for containerizing services, Kubernetes manages the orchestration, and Jenkins handles the CI/CD pipeline.
4. What is Infrastructure as Code (IaC)? Which tools are used for IaC?
Answer:
IaC is the practice of managing and provisioning infrastructure through machine-readable configuration files, rather than physical hardware configuration.
Tools:
- Terraform
- AWS CloudFormation
- Ansible
Example:
Using Terraform, you can write a configuration file to provision an AWS EC2 instance:
resource “aws_instance” “example” {
ami = “ami-12345678”
instance_type = “t2.micro”
}
Running terraform apply sets up the infrastructure automatically.
5. How do you monitor and ensure the reliability of applications in a DevOps environment?
Answer:
Monitoring involves tracking application performance, uptime, and errors using tools.
Best Practices:
- Use tools like Prometheus and Grafana for real-time monitoring.
- Set up alerting systems with tools like PagerDuty.
- Implement logging with ELK Stack (Elasticsearch, Logstash, Kibana).
Example:
A monitoring dashboard with Grafana shows CPU usage and memory consumption for a Kubernetes cluster. If CPU usage exceeds 80%, an alert is sent to the operations team.
6. Explain the concept of Blue-Green Deployment.
Answer:
Blue-Green Deployment is a technique to reduce downtime and risk during application updates by maintaining two environments:
- Blue: The current live environment.
- Green: The new version of the application.
Process:
- Deploy the new application version to the Green environment.
- Test the Green environment.
- Switch traffic from Blue to Green if tests are successful.
Example:
An e-commerce site updates its payment module. The Green environment runs the new module. Once validated, DNS routing is switched from Blue to Green.
7. What are some common challenges faced in DevOps implementation? How can they be mitigated?
Answer:
Challenges:
- Resistance to cultural change.
- Lack of standardization in tools.
- Inefficient CI/CD pipelines.
Mitigation Strategies:
- Promote collaboration and communication through regular meetings and shared goals.
- Use standardized tools across teams.
- Regularly optimize CI/CD pipelines for performance.
Example:
To address tool standardization, a company standardizes its CI/CD process using Jenkins and Ansible, ensuring all teams follow the same workflow.
8. How do you handle security in a DevOps workflow (DevSecOps)?
Answer:
DevSecOps integrates security into every stage of the DevOps lifecycle.
Practices:
- Use tools like SonarQube for static code analysis.
- Implement secret management tools like HashiCorp Vault.
- Perform regular vulnerability scanning with tools like OWASP ZAP.
Example:
Before deploying an application, the CI/CD pipeline runs a security scan using SonarQube and alerts the team if any vulnerabilities are found.
9. How does Docker differ from virtual machines (VMs)?
Answer:
Docker is a containerization platform that allows applications to run in lightweight, isolated environments.
Key Differences:
- Docker: Shares the host OS kernel; faster and uses fewer resources.
- VMs: Include a full OS, making them heavier and slower.
Example:
Running 10 Docker containers on a server takes less memory compared to running 10 VMs because containers share the OS kernel.
10. What is the role of Kubernetes in DevOps?
Answer:
Kubernetes is a container orchestration tool that automates the deployment, scaling, and management of containerized applications.
Features:
- Automated scaling.
- Self-healing capabilities.
- Load balancing.
Example:
For a web application with fluctuating traffic, Kubernetes automatically scales the number of pods up during high demand and down during low demand, ensuring efficient resource utilization.
11. What are Microservices, and how do they relate to DevOps?
Answer:
Microservices are an architectural style where applications are built as a collection of small, independent services that communicate through APIs.
Relation to DevOps:
- Encourages continuous deployment by isolating service updates.
- Enhances scalability and fault tolerance.
- Works well with DevOps tools like Kubernetes and Docker.
Example:
An e-commerce app might have separate microservices for user authentication, product catalog, and payment processing. DevOps practices ensure that each microservice is tested and deployed independently.
12. What are the differences between Continuous Deployment and Continuous Delivery?
Answer:
- Continuous Delivery: Ensures that code changes are automatically tested and ready for manual deployment.
- Continuous Deployment: Automates the entire process, deploying code changes to production without manual intervention.
Example:
In Continuous Deployment, every successful build in Jenkins gets deployed directly to the live environment. In Continuous Delivery, the deployment step is manual.
13. How do you handle rollbacks in a CI/CD pipeline?
Answer:
Rollbacks are crucial to mitigate issues caused by faulty deployments.
Methods:
- Use version control tools like Git to revert to a previous commit.
- Leverage containerization to deploy the previous stable image.
- Implement feature toggles to disable new features.
Example:
If a new deployment fails, Kubernetes can roll back to the last stable deployment automatically using kubectl rollout undo.
14. How do you implement security in containerized environments?
Answer:
Securing containers is essential for protecting applications.
Best Practices:
- Use minimal base images to reduce vulnerabilities.
- Regularly scan images with tools like Aqua Security or Trivy.
- Implement Role-Based Access Control (RBAC) in Kubernetes.
Example:
A Docker image is scanned with Trivy before being deployed to a Kubernetes cluster. Any vulnerabilities are fixed before deployment proceeds.
15. What is Git, and why is it essential in DevOps?
Answer:
Git is a distributed version control system that tracks changes in code and facilitates collaboration among developers.
Importance in DevOps:
- Enables version control of code.
- Facilitates collaboration through branching and merging.
- Integrates with CI/CD tools for automated workflows.
Example:
Multiple developers work on different features using separate branches in Git. Changes are merged into the main branch after successful testing.
16. What are Git branches, and why are they used?
Answer:
A branch in Git is an independent line of development.
Usage:
- To work on features, bug fixes, or experiments without affecting the main codebase.
- To isolate development until changes are tested and ready to merge.
Example:
A developer creates a feature branch:
git checkout -b feature-login
Once the feature is complete, it is merged into the main branch.
17. How do you resolve a merge conflict in Git?
Answer:
A merge conflict occurs when changes in two branches conflict with each other.
Steps to Resolve:
- Identify conflicting files during the merge.
- Open the files and manually resolve conflicts by editing the conflicting sections.
- Mark the file as resolved using git add.
- Commit the changes.
Example:
git merge feature-branch
# Resolve conflicts in file.txt
git add file.txt
git commit -m “Resolved merge conflict in file.txt”
18. What is the difference between git pull and git fetch?
Answer:
- git fetch: Downloads changes from the remote repository but does not merge them into your local branch.
- git pull: Combines git fetch and git merge, downloading changes and merging them into the current branch.
Example:
To inspect remote changes before merging:
git fetch
git diff origin/main
19. How do you revert a commit in Git?
Answer:
You can undo changes made by a specific commit without deleting the commit itself using git revert
.
Example:
git revert <commit-hash>
20. How do you tag a specific commit in Git? Why is it useful?
Answer:
Tagging: A way to mark a specific commit with a meaningful name, often used for release versions.
Example:
git tag -a v1.0 -m “Version 1.0 release”
git push origin v1.0
Tags make it easy to identify and retrieve specific versions of the code.
21. Explain the Gitflow Workflow.
Answer:
Gitflow is a branching strategy used for collaborative development.
Key Branches:
- Main: The production-ready code.
- Develop: The integration branch.
- Feature: For individual feature development.
- Release: For preparing a release.
- Hotfix: For critical bug fixes in production.
Example:
A developer creates a feature branch from develop, completes the feature, and merges it back into develop.
By mastering these Git-related questions and other DevOps concepts, you’ll be well-prepared to demonstrate your expertise during interviews.
🚀 Elevate Your Career with Ignisys IT Training Programs!
Are you ready to boost your skills and achieve your career goals? Join Ignisys IT for industry-leading training programs designed to empower you with the latest tools and technologies.
📩 Take the first step towards success!
Enroll today by reaching out to us
🔗 Let’s shape your future together at Ignisys IT!
Leave a Reply