Demystifying Database Cursors: Benefits, Overview, and Examples
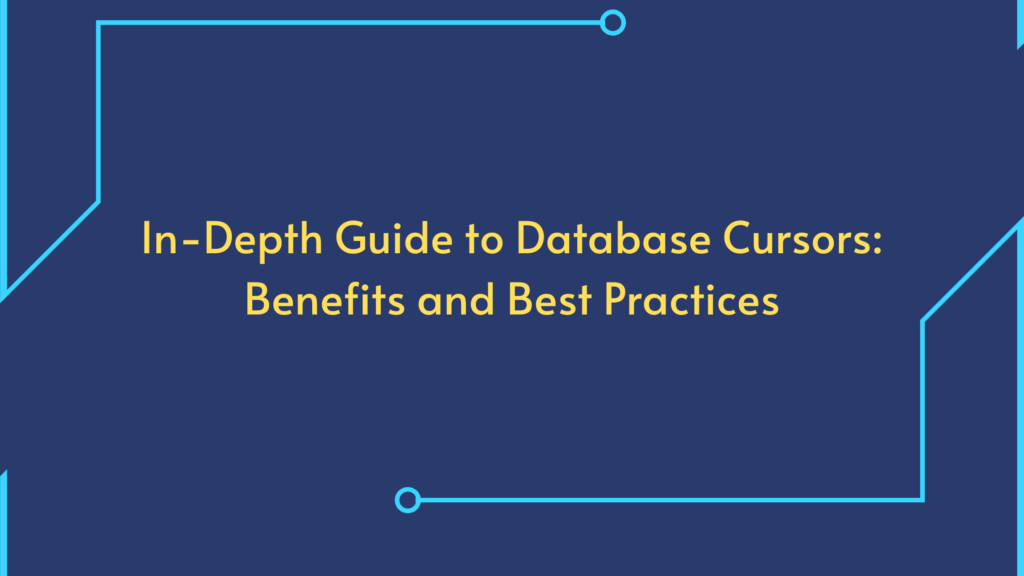
Introduction
In the world of databases, cursors are a powerful tool that allows for fine-grained control and manipulation of data. Cursors provide a mechanism to traverse through query result sets one row at a time, enabling developers to perform complex data operations. In this comprehensive blog post, we’ll dive deep into what cursors are, explore their benefits, discuss the types of cursors, and provide practical examples to illustrate their usage.
What is a Cursor?
In the context of databases, a cursor is a database object or a programming construct that provides a way to work with individual rows of a result set generated by a SQL query. Unlike fetching the entire result set in one go, cursors allow you to retrieve, process, and manipulate data row by row.
Benefits of Using Cursors
Cursors offer several advantages when working with databases:
1. Precise Data Manipulation:
Cursors enable developers to process and manipulate individual rows within a result set, offering fine-grained control over data operations. This level of precision is invaluable for complex data transformations.
2. Reduced Memory Consumption:
When dealing with large result sets, cursors are memory-efficient. They fetch and process data one row at a time, which minimizes memory usage compared to loading the entire result set into memory.
3. Custom Data Filtering and Sorting:
Cursors allow you to filter and sort data as it is fetched, providing the flexibility to customize data retrieval according to specific criteria. This is particularly useful when working with dynamic or evolving datasets.
4. Real-time Data Processing:
Cursors are well-suited for real-time data processing scenarios where data is constantly changing. You can process data as soon as it becomes available without waiting for the entire dataset to be loaded.
5. Transaction Management:
Cursors can be used within database transactions, ensuring data integrity while performing complex operations that involve multiple rows. This is essential for maintaining data consistency.
Types of Cursors
There are two main types of cursors:
1. Implicit Cursors:
Implicit cursors are automatically created and managed by the database management system (DBMS) to handle SQL operations. They are typically used for executing queries, fetching data, and returning results without developer intervention.
2. Explicit Cursors:
Explicit cursors are created and controlled by developers through procedural programming languages such as PL/SQL, T-SQL, or other database-specific languages. Developers have greater control over explicit cursors, allowing for more complex data operations and customizations.
Practical Examples of Using Cursors
Let’s explore two practical examples of using cursors in SQL and PL/SQL:
SQL Example:
Suppose we have a “Products” table with columns “ProductID” and “ProductName.” We want to retrieve and display the names of all products using a cursor.
DECLARE @ProductName NVARCHAR(255)
DECLARE ProductCursor CURSOR FOR
SELECT ProductName FROM Products
OPEN ProductCursor
FETCH NEXT FROM ProductCursor INTO @ProductName
WHILE @@FETCH_STATUS = 0
BEGIN
PRINT @ProductName
FETCH NEXT FROM ProductCursor INTO @ProductName
END
CLOSE ProductCursor
DEALLOCATE ProductCursor
PL/SQL Example:
In Oracle PL/SQL, you can use explicit cursors to achieve similar functionality:
DECLARE
v_product_name Products.ProductName%TYPE;
CURSOR product_cursor IS
SELECT ProductName FROM Products;
BEGIN
OPEN product_cursor;
LOOP
FETCH product_cursor INTO v_product_name;
EXIT WHEN product_cursor%NOTFOUND;
DBMS_OUTPUT.PUT_LINE(v_product_name);
END LOOP;
CLOSE product_cursor;
END;
/
In both examples, we declare a cursor, open it, fetch rows one by one, and process the data accordingly.
Conclusion
Cursors are valuable assets in the database developer’s toolkit, offering precise data manipulation, memory efficiency, custom data filtering, and real-time processing capabilities. However, it’s essential to use cursors judiciously, as improper usage can lead to performance issues. Understanding how and when to leverage cursors empowers developers to work with data effectively and efficiently in a database environment, making it a crucial skill in the realm of database management.
Leave a Reply