Exploring the Effective Use of While Loop in Oracle Integration Cloud (OIC)
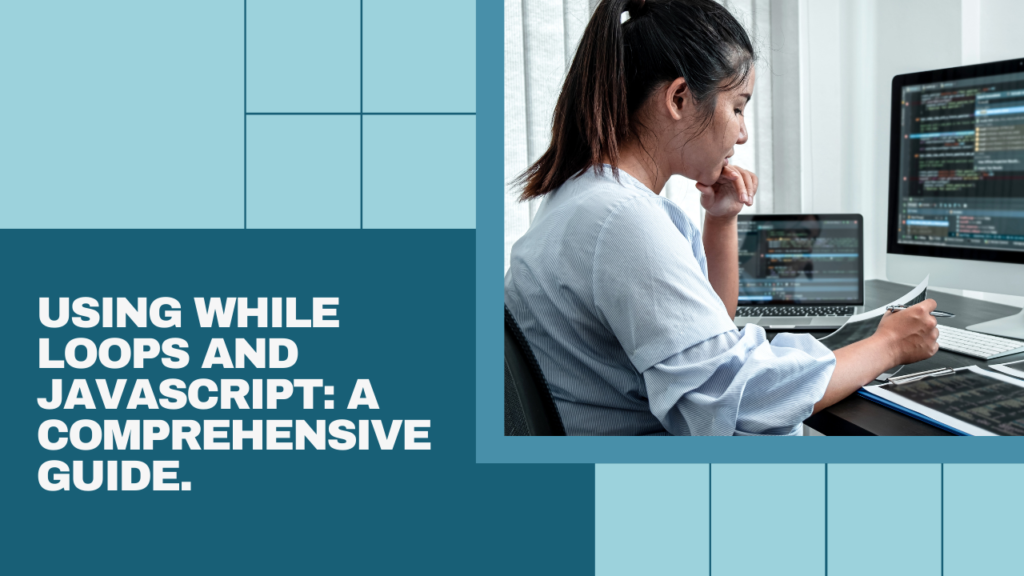
Introduction:
While Oracle Integration Cloud (OIC) offers robust functionalities for integration tasks, incorporating custom JavaScript code or libraries can further enhance its capabilities. Leveraging while loops, a fundamental programming construct, within OIC can efficiently handle iterative processes. Here’s a comprehensive guide on utilizing while loops within OIC and integrating JavaScript code to optimize your integration workflows.
Utilizing While Loop in OIC:
- Understanding While Loops:
- While loops are iterative structures that repeatedly execute a block of code while a specified condition holds true.
- Within OIC, while loops can be employed to perform repetitive tasks, iterate over data sets, or execute logic until a particular condition is met.
- Integrating JavaScript Code:
a. External Service Integration: – Create a RESTful web service or an external HTTP endpoint hosted externally to execute JavaScript logic. – Use “Invoke REST Service” or “Invoke HTTP Service” activities within OIC to make requests to this external service, passing necessary data and receiving results.
b. Custom Code Activities in OIC: – Utilize OIC’s capability to create custom code activities and write JavaScript code directly within them. – JavaScript code in these activities can handle data transformations, calculations, or other specific logic required for your integration.
c. JavaScript Cloud Functions: – Explore serverless computing platforms like Oracle Functions, AWS Lambda, or Azure Functions supporting JavaScript execution. – Develop serverless functions using JavaScript and expose them as APIs, invoking these functions from OIC integrations through HTTP calls.
d. JavaScript within Adapter Transformations: – Certain OIC adapters might support JavaScript or scripting languages for transformation logic within adapter mappings. – Review the adapter documentation to check if it allows scripting within transformations for enhanced integration capabilities.
Conclusion:
By leveraging while loops and integrating JavaScript code into Oracle Integration Cloud, developers can enhance the functionality and flexibility of their integrations. Whether through custom code activities, external services, or serverless functions, utilizing JavaScript within OIC empowers users to efficiently handle iterative tasks and implement tailored logic within their integration workflows.
Remember to refer to Oracle’s documentation and specific adapter capabilities for optimal utilization of while loops and JavaScript integration within OIC.
Certainly! Here are some hypothetical examples demonstrating the use of while loops and integration of JavaScript code within Oracle Integration Cloud (OIC):
Example 1: Custom Code Activity with While Loop
Scenario: You have a requirement to process a list of orders until a specific condition is met. Utilize a while loop within a custom code activity to handle this iterative task.
// Custom Code Activity in OIC
var orders = getOrders(); // Function to retrieve orders
while (orders.length > 0) {
var currentOrder = orders.pop(); // Process orders in reverse order
// Perform operations on the current order
processOrder(currentOrder);
// Check condition or perform other actions
// For instance, break loop if specific criteria are met
if (criteriaMet(currentOrder)) {
break;
}
}
Explanation:
- getOrders() retrieves a list of orders to be processed.
- The while loop iterates through the orders until the condition (criteriaMet) is fulfilled, then breaks the loop.
- processOrder() represents the logic for handling each order.
Example 2: Using External Service Integration
Scenario: Calculate statistics from external data using JavaScript and integrate it into OIC through an external service.
External JavaScript code (hosted on a server):
// External JavaScript Code
function calculateStatistics(data) {
// Perform statistical calculations
let total = 0;
for (let i = 0; i < data.length; i++) {
total += data[i];
}
return {
count: data.length,
sum: total,
average: total / data.length
};
}
Integration within OIC:
- Create a RESTful endpoint (e.g., /calculate-statistics) hosting the above JavaScript code.
- Use OIC’s “Invoke REST Service” activity to make an HTTP request to this endpoint, passing data.
- Receive the calculated statistics as the service response and handle it within the OIC integration.
Example 3: Utilizing JavaScript Cloud Functions
Scenario: Develop a serverless function using JavaScript (e.g., Oracle Functions) to manipulate incoming data and integrate it into an OIC workflow.
JavaScript Cloud Function:
// Oracle Functions – JavaScript Cloud Function
function processData(data) {
// Perform data processing or transformation
let processedData = data.map(item => {
return {
id: item.id,
modifiedValue: item.value * 2 // Manipulate incoming data
};
});
return processedData;
}
Integrating with OIC:
- Expose the Oracle Functions endpoint as an API.
- Within OIC, invoke this function by making an HTTP call to the exposed endpoint.
- Process the returned data from the function within the OIC integration flow.
These examples illustrate how while loops and JavaScript integration can be applied within OIC to handle iterative tasks, perform data processing, and interact with external services or functions.
Leave a Reply