Exploring the Power of PyTorch: A Comprehensive Guide to its Basics and Real-World Applications
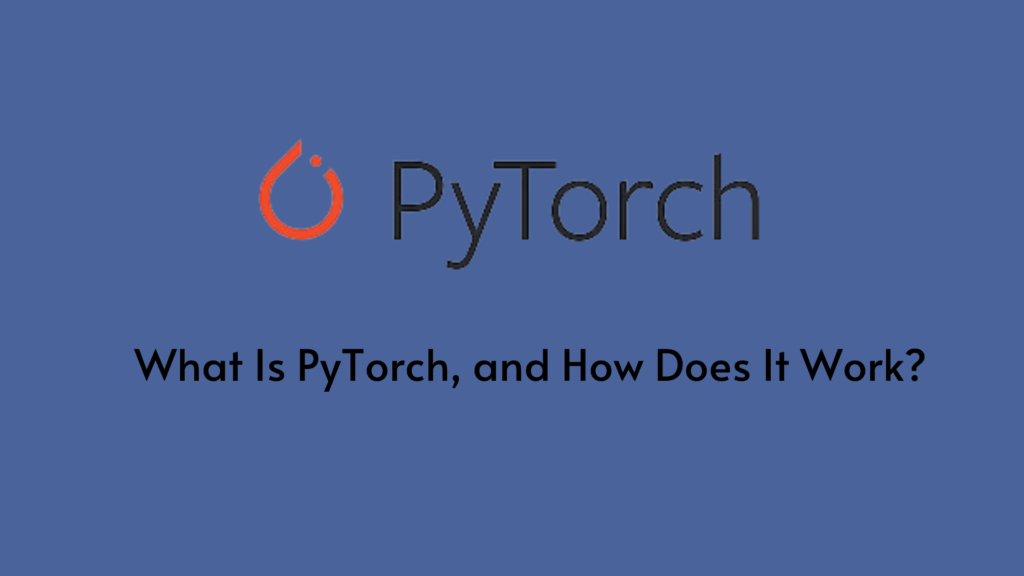
Intro: In the ever-evolving world of deep learning and artificial intelligence, PyTorch has established itself as a formidable framework for both researchers and developers. Its flexibility and dynamic computation graph make it a popular choice for building cutting-edge neural networks. In this blog, we’ll take a deep dive into the world of PyTorch, understanding its basics, key modules, dynamic computation graph, data loaders, and even apply its prowess to solve a real-world problem – image classification.
What Is PyTorch?
PyTorch is an open-source machine learning library developed by Facebook’s AI Research lab (FAIR). It is known for its flexibility, dynamic computation graph, and ease of use. Unlike other deep learning frameworks, PyTorch adopts an imperative programming style, which makes it more intuitive and easier to debug.
Basics of PyTorch
- Tensors: At the core of PyTorch are tensors, which are multi-dimensional arrays similar to NumPy arrays. PyTorch tensors can be used for various mathematical operations and are essential for building neural networks.
- Example: Creating Tensors
- One of the fundamental elements of PyTorch is tensors. These multi-dimensional arrays are similar to NumPy arrays but come with the added advantage of GPU acceleration for faster computation. Let’s create a simple tensor:
import torch
# Create a 2×3 tensor
x = torch.tensor([[1, 2, 3], [4, 5, 6]])
print(x)
2. Autograd: PyTorch’s automatic differentiation library, Autograd, allows you to compute gradients of tensors, which is crucial for training neural networks using gradient-based optimization algorithms.
- Example: Automatic Differentiation with Autograd
import torch
x = torch.tensor([2.0], requires_grad=True)
y = x**2
y.backward()
print(x.grad)
- Autograd is PyTorch’s automatic differentiation library, which is vital for training neural networks. Here’s a quick example of how to use Autograd to calculate gradients:
- Example: Automatic Differentiation with Autograd
- Autograd is PyTorch’s automatic differentiation library, which is vital for training neural networks. Here’s a quick example of how to use Autograd to calculate gradients:
import torch
x = torch.tensor([2.0], requires_grad=True)
y = x**2
y.backward()
print(x.grad)
- pythonCo
3. Neural Networks: PyTorch provides a high-level neural network module that simplifies the creation and training of neural networks. You can define layers, loss functions, and optimizers with ease.
- Example: Building a Simple Neural Network
- PyTorch provides a high-level neural network module for creating and training neural networks. Here’s an example of a simple feedforward neural network:
import torch
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init()
self.fc1 = nn.Linear(2, 3)
self.fc2 = nn.Linear(3, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# Initialize and use the network
net = Net()
output = net(torch.tensor([1.0, 2.0]))
Common PyTorch Modules
PyTorch offers several modules for different aspects of deep learning, such as:
- nn.Module: This module provides a base class for defining custom neural network architectures.
- nn.functional: It contains various functions that can be used within neural network architectures, including activation functions, loss functions, and more.
- Example: Using
nn.Module
andnn.functional
- PyTorch provides the
nn.Module
class for defining custom neural network architectures and thenn.functional
module for functions used within network layers. In this example, we’ll create a simple convolutional neural network (CNN):
import torch
import torch.nn as nn
class SimpleCNN(nn.Module):
def __init__(self):
super(SimpleCNN, self).__init__()
self.conv1 = nn.Conv2d(1, 16, kernel_size=3, stride=1, padding=1)
self.fc1 = nn.Linear(16*14*14, 10)
def forward(self, x):
x = torch.relu(self.conv1(x))
x = x.view(x.size(0), -1)
x = self.fc1(x)
return x
# Instantiate the CNN
cnn = SimpleCNN()
- optim: PyTorch’s optim module provides various optimization algorithms like SGD, Adam, and RMSprop, which are crucial for training models.
- Example: Optimizing with the
optim
Module - PyTorch’s
optim
module provides a wide range of optimization algorithms. Here, we’ll use the Adam optimizer to train a neural network:
import torch
import torch.optim as optim
# Define a simple neural network
net = Net()
# Define the optimizer
optimizer = optim.Adam(net.parameters(), lr=0.001)
Dynamic Computation Graph
One of PyTorch’s distinguishing features is its dynamic computation graph. Unlike static computation graphs found in some other deep learning frameworks, PyTorch’s graph is built on-the-fly, which allows for dynamic, on-the-fly changes to the network structure. This is particularly beneficial when dealing with sequences or variable-length inputs.
Example: Dynamic Computation Graph in Action
PyTorch’s dynamic computation graph is incredibly powerful when dealing with variable-length sequences. Here’s an example of how it works:
import torch
# Dynamic computation graph
x = torch.tensor([1.0], requires_grad=True)
y = x**2
z = 2*y + 3
z.backward()
print(x.grad)
Data Loader
For efficient data handling, PyTorch offers the DataLoader class. This allows you to load and preprocess data in parallel, making it easier to work with large datasets. It’s an essential component for training deep learning models.
Example: Using Data Loaders for Image Classification
Data loaders are essential for efficient data handling. Let’s see how to use a data loader to train an image classification model:
import torch
from torchvision import datasets, transforms
from torch.utils.data import DataLoader
# Define data transformations
transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5, 0.5, 0.5), (0.5, 0.5, 0.5))])
# Load the CIFAR-10 dataset
train_dataset = datasets.CIFAR10(root=’./data’, train=True, transform=transform, download=True)
train_loader = DataLoader(train_dataset, batch_size=32, shuffle=True)
Solving an Image Classification Problem Using PyTorch
To demonstrate PyTorch in action, we’ll walk you through the process of solving an image classification problem. We’ll cover data preprocessing, defining a neural network, training the model, and evaluating its performance. You’ll see how PyTorch simplifies the development of complex machine learning tasks.
Example: Image Classification with a Convolutional Neural Network (CNN)
Let’s put everything together by creating a complete example of solving an image classification problem using PyTorch and a CNN:
import torch
import torch.nn as nn
import torch.optim as optim
from torchvision import datasets, transforms
from torch.utils.data import DataLoader
# Define and train a CNN for image classification
# …
In conclusion, PyTorch’s versatility and dynamic capabilities make it an invaluable tool for deep learning enthusiasts. With this comprehensive guide and practical examples, you now have a strong foundation to explore the vast possibilities that PyTorch offers in the world of artificial intelligence and machine learning. Whether you’re a seasoned practitioner or just starting out, PyTorch’s power is at your fingertips. Happy exploring!
Leave a Reply