Master SQL with Conditional Statements: A Comprehensive Guide
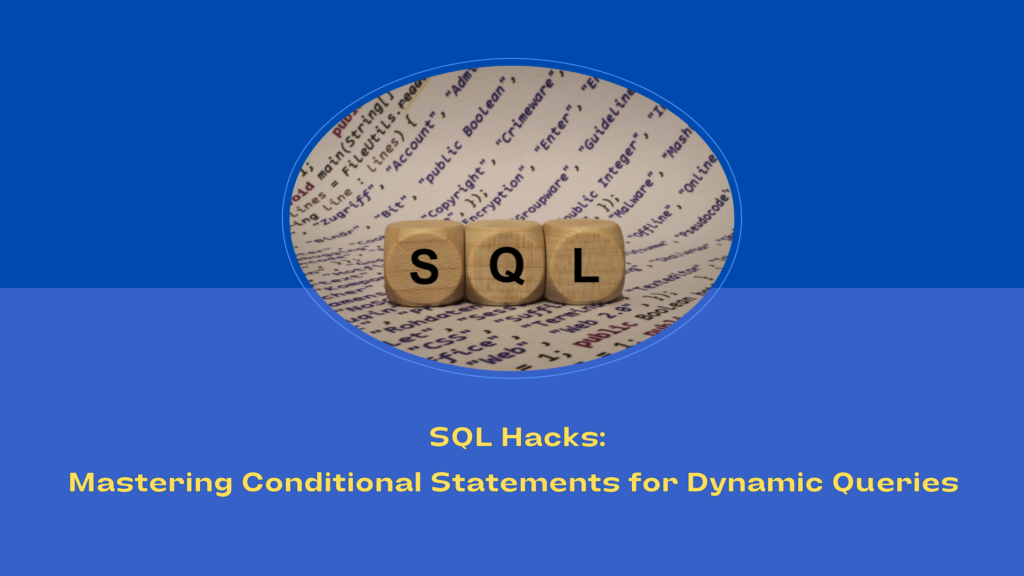
SQL (Structured Query Language) is the backbone of database management and plays a crucial role in retrieving, manipulating, and analyzing data. One of the most powerful aspects of SQL is conditional logic, which allows users to control the flow of queries and execute different actions based on specific conditions.
Conditional statements make SQL more flexible and dynamic, enabling developers to handle real-world business scenarios efficiently. This blog will explore SQL’s conditional statements, their syntax, and how to use them with real-world examples to improve query performance and decision-making.
Understanding Conditional Statements in SQL
Conditional statements in SQL help control query logic by executing different actions based on conditions. The most commonly used conditional statements include:
- CASE Statement – Executes different results based on multiple conditions.
- IF Statement (in procedural SQL like PL/SQL, T-SQL) – Evaluates conditions and acts.
- IF-ELSE Logic (via CASE or procedural SQL) – Determines alternative actions.
- COALESCE & NULLIF Functions – Handle null values efficiently.
1. The CASE Statement in SQL
The CASE statement is one of the most versatile conditional expressions in SQL. It works like an IF-ELSE statement in programming languages and helps return different values based on conditions.
Syntax of CASE Statement
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
ELSE default_result
END
Example 1: Categorizing Employees by Salary Range
Suppose we have an employees
table and we want to classify employees based on their salaries.
SELECT employee_id, name, salary,
CASE
WHEN salary >= 80000 THEN ‘High Earner’
WHEN salary BETWEEN 50000 AND 79999 THEN ‘Mid Earner’
ELSE ‘Low Earner’
END AS salary_category
FROM employees;
Output:
Employee_ID | Name | Salary | Salary_Category |
101 | Alice | 90000 | High Earner |
102 | Bob | 65000 | Mid Earner |
103 | Charlie | 40000 | Low Earner |
✅ Use Case: This approach is helpful for financial reports, HR analytics, and decision-making processes.
2. Using CASE in the WHERE Clause
The CASE
statement can also be used in the WHERE
clause to dynamically filter results based on conditions.
Example 2: Filtering Employees Based on Dynamic Criteria
Suppose we want to retrieve employees earning more than $60,000 only if they belong to the IT department; otherwise, we retrieve employees earning more than $50,000.
SELECT employee_id, name, department, salary
FROM employees
WHERE salary >
CASE
WHEN department = ‘IT’ THEN 60000
ELSE 50000
END;
✅ Use Case: This is useful for department-specific salary analysis without writing separate queries for each department.
3. Using CASE in ORDER BY for Custom Sorting
SQL allows dynamic sorting using the CASE
statement in the ORDER BY
clause.
Example 3: Sorting Employees Based on Custom Priority
Let’s say we want to sort employees first by salary category (High → Low) and then by salary (descending).
✅ Use Case: Sorting employees based on business-defined categories improves data presentation and reporting.
4. IF and IF-ELSE in Procedural SQL (PL/SQL & T-SQL)
In procedural SQL (e.g., PL/SQL for Oracle, T-SQL for SQL Server), IF
and IF-ELSE
statements are used in stored procedures, triggers, and functions for decision-making.
Example 4: Using IF-ELSE in a Stored Procedure (PL/SQL)
CREATE PROCEDURE update_salary(employee_id INT, new_salary DECIMAL)
AS
BEGIN
IF new_salary < 40000 THEN
UPDATE employees SET salary = salary * 1.05 WHERE employee_id = employee_id;
ELSE
UPDATE employees SET salary = new_salary WHERE employee_id = employee_id;
END IF;
END;
✅ Use Case: Helps automate salary adjustments based on predefined rules.
5. Handling NULL Values with COALESCE and NULLIF
COALESCE: Replacing NULL with a Default Value
COALESCE()
returns the first non-null value from a list of columns.
SELECT employee_id, name, COALESCE(phone_number, ‘Not Provided’) AS contact_info
FROM employees;
✅ Use Case: Useful for data cleaning and ensuring NULL values do not impact reports.
NULLIF: Avoiding Division by Zero Errors
NULLIF(a, b)
returns NULL
if a = b
; otherwise, it returns a
.
SELECT employee_id, salary / NULLIF(bonus, 0) AS salary_to_bonus_ratio
FROM employees;
✅ Use Case: Prevents division by zero errors, which can cause runtime failures.
6. Using CASE with Aggregate Functions
Conditional logic can be applied within SUM(), COUNT(), AVG(), etc., for advanced analytics.
Example 5: Conditional Aggregation – Counting Employees by Category
SELECT
COUNT(CASE WHEN salary >= 80000 THEN 1 END) AS high_earners,
COUNT(CASE WHEN salary BETWEEN 50000 AND 79999 THEN 1 END) AS mid_earners,
COUNT(CASE WHEN salary < 50000 THEN 1 END) AS low_earners
FROM employees;
✅ Use Case: Quickly categorize data for business insights without complex queries.
Key Takeaways: Why Master Conditional Statements in SQL?
🔹 Improved Query Flexibility: Conditional logic allows dynamic data filtering, sorting, and categorization.
🔹 Enhanced Decision Making: Businesses can analyze and process data based on complex conditions.
🔹 Efficient Data Handling: NULL values, error handling, and aggregation become more streamlined.
🔹 Better Performance & Readability: Using CASE instead of multiple queries simplifies SQL scripts.
Final Thought:
Mastering conditional statements in SQL elevates your ability to analyze and manipulate data efficiently. Whether you’re building reports, processing business logic, or optimizing queries, these techniques are indispensable.
🚀 Next Steps:
✅ Practice CASE
, IF
, COALESCE
, and NULLIF
on real-world datasets.
✅ Apply conditional aggregation for insightful reports.
✅ Use conditional sorting to improve query readability.
Boost Your Career with SQL Training at Ignisys IT!
Master the power of SQL and take your data management and analytics skills to the next level with Ignisys IT’s expert-led training! Whether you’re a beginner or looking to refine your SQL expertise, our training covers everything from fundamentals to advanced techniques like:
✅ SQL Queries & Data Manipulation
✅ Joins, Subqueries & Indexing
✅ Stored Procedures & Triggers
✅ Conditional Statements & Advanced SQL Functions
✅ Real-world Projects & Hands-on Practice
💡 Future-proof your career! Learn SQL and unlock opportunities in Data Science, Business Intelligence, and Database Administration.
📩 Enroll today! Contact us to kickstart your SQL journey with Ignisys IT.
Leave a Reply