PL/SQL Tutorials: A Comprehensive Guide to Mastering Oracle’s Procedural Language
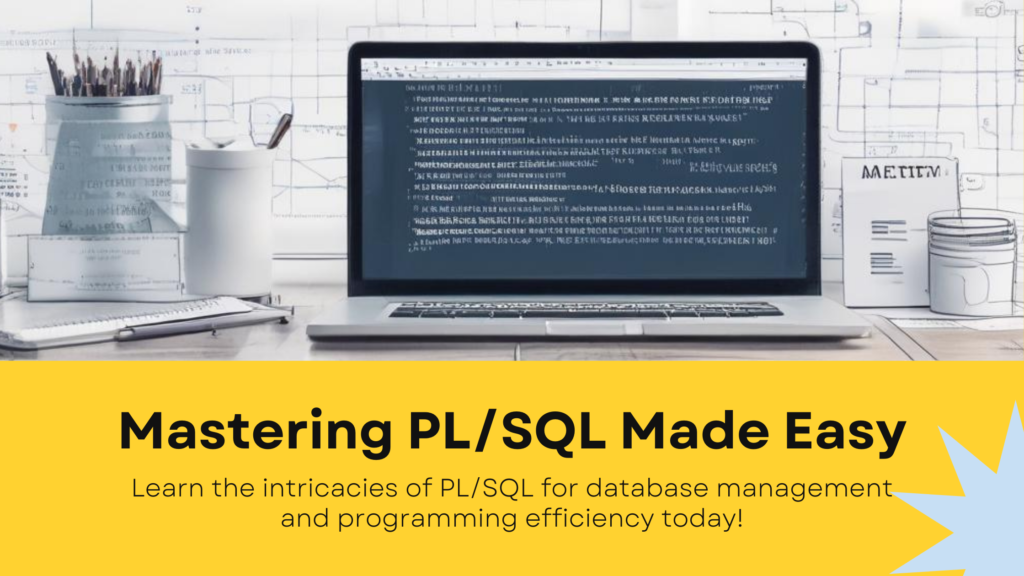
PL/SQL (Procedural Language/SQL) is Oracle’s extension to SQL, designed to allow developers to combine the power of SQL with procedural programming constructs. It adds programming capabilities such as loops, conditions, variables, and error handling, making it an indispensable tool for developers working with Oracle databases.
In this comprehensive guide, we will explore the basics and advanced features of PL/SQL and demonstrate how to use it effectively to improve your database programming skills.
What is PL/SQL?
PL/SQL is Oracle’s procedural language extension to SQL that allows users to write complex queries, execute multiple SQL statements, and perform logic-based tasks within the database itself. Unlike SQL, which is declarative and designed primarily for querying and updating data, PL/SQL enables developers to embed procedural logic, allowing them to define functions, procedures, and triggers that can run directly within the database.
PL/SQL is designed for use with Oracle databases, but many of its principles can be applied across other RDBMS (Relational Database Management Systems) that support procedural SQL extensions.
Getting Started with PL/SQL
Before diving into the more advanced aspects of PL/SQL, it’s important to start with the basic syntax and structure of a PL/SQL block. A basic PL/SQL block consists of three sections:
- Declaration Section: This is where variables, constants, cursors, and exceptions are defined.
- Execution Section: The core part of the block, where SQL queries and PL/SQL logic are executed.
- Exception Handling Section: This section captures and handles errors that occur during execution.
Here’s a simple example of a PL/SQL block: DECLARE
v_employee_id NUMBER := 100;
v_employee_name VARCHAR2(50);
BEGIN
— Fetch employee details
SELECT first_name INTO v_employee_name
FROM employees
WHERE employee_id = v_employee_id;
DBMS_OUTPUT.PUT_LINE(‘Employee Name: ‘ || v_employee_name);
EXCEPTION
WHEN NO_DATA_FOUND THEN
DBMS_OUTPUT.PUT_LINE(‘Employee not found.’);
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE(‘Error occurred: ‘ || SQLERRM);
END;
In the above example:
- We declare a variable v_employee_id to hold the employee’s ID and v_employee_name to hold the employee’s name.
- The SELECT statement fetches the employee’s first name and stores it in v_employee_name.
- The exception block catches any errors that may occur, such as if no employee is found for the given ID.
Understanding Key PL/SQL Constructs: A Detailed Overview
PL/SQL (Procedural Language/SQL) is a powerful extension of SQL, providing users with the ability to write complex queries, control flow logic, and handle errors directly within the Oracle database. Understanding the key constructs of PL/SQL is essential to master this language and harness its full potential. In this section, we will delve into some of the key features and concepts that make PL/SQL a unique and effective tool for database developers.
1. Variables and Data Types in PL/SQL
In PL/SQL, variables are used to store data that can be manipulated during the execution of a program. These variables are declared in the declaration section of a PL/SQL block and can store values of different data types such as numbers, strings, dates, and even booleans.
Common Data Types:
- NUMBER: Stores numeric values, including integers and decimals.
- VARCHAR2: Stores variable-length character strings.
- CHAR: Stores fixed-length character strings.
- DATE: Stores date and time information.
- BOOLEAN: Stores logical values
TRUE
orFALSE
.
Example:
DECLARE
v_salary NUMBER(8,2); — variable for salary
v_employee_name VARCHAR2(50); — variable for employee name
BEGIN
v_salary := 5000.50;
v_employee_name := ‘John Doe’;
DBMS_OUTPUT.PUT_LINE(‘Employee Name: ‘ || v_employee_name || ‘, Salary: ‘ || v_salary);
END;
In this example, two variables are declared: v_salary
(for storing a numeric value) and v_employee_name
(for storing a string). These variables are then assigned values and used within the program.
2. Control Structures in PL/SQL
PL/SQL includes several control structures that allow you to implement logic and decision-making processes in your code. The most commonly used control structures are:
a. IF-ELSE Statement
The IF-ELSE
statement enables branching based on a condition. You can check conditions and perform specific actions depending on the outcome.
DECLARE
v_salary NUMBER := 7000;
BEGIN
IF v_salary > 5000 THEN
DBMS_OUTPUT.PUT_LINE(‘Salary is above average’);
ELSE
DBMS_OUTPUT.PUT_LINE(‘Salary is below average’);
END IF;
END;
b. CASE Statement
The CASE
statement is a more flexible way of handling multiple conditions. It’s similar to the IF-ELSE
, but you can compare a value against several possible outcomes.
DECLARE
v_grade CHAR(1) := ‘A’;
BEGIN
CASE v_grade
WHEN ‘A’ THEN
DBMS_OUTPUT.PUT_LINE(‘Excellent’);
WHEN ‘B’ THEN
DBMS_OUTPUT.PUT_LINE(‘Good’);
ELSE
DBMS_OUTPUT.PUT_LINE(‘Needs Improvement’);
END CASE;
END;
c. Loops (FOR, WHILE, and LOOP)
PL/SQL offers several types of loops to handle repetitive tasks.
- FOR Loop: Ideal when you know the number of iterations in advance.
- WHILE Loop: Useful when the number of iterations is unknown, and the loop continues as long as a condition is true.
- LOOP: A general-purpose loop that runs until an explicit exit condition is met.
Example of a FOR
loop:
DECLARE
v_counter NUMBER := 1;
BEGIN
FOR v_counter IN 1..5 LOOP
DBMS_OUTPUT.PUT_LINE(‘Iteration: ‘ || v_counter);
END LOOP;
END;
3. Cursors in PL/SQL
Cursors are a fundamental concept in PL/SQL. They are pointers to SQL query results that allow you to fetch rows one by one and process them. There are two types of cursors:
a. Implicit Cursors
Implicit cursors are automatically created by Oracle for single SQL queries (such as SELECT
, INSERT
, UPDATE
, or DELETE
). These cursors do not require explicit declaration in PL/SQL code.
Example:
BEGIN
UPDATE employees
SET salary = 6000
WHERE employee_id = 100;
COMMIT;
END;
b. Explicit Cursors
Explicit cursors provide greater control over the result set and are especially useful when you need to perform more complex processing of query results.
- Declaring a Cursor: First, you declare a cursor based on a SQL query.
- Opening the Cursor: You open the cursor to execute the query and retrieve the result set.
- Fetching Data: You fetch data row by row using the
FETCH
statement. - Closing the Cursor: After processing, you close the cursor to release resources.
Example of an explicit cursor:
DECLARE
CURSOR emp_cursor IS
SELECT employee_id, first_name FROM employees;
v_emp_id employees.employee_id%TYPE;
v_emp_name employees.first_name%TYPE;
BEGIN
OPEN emp_cursor;
LOOP
FETCH emp_cursor INTO v_emp_id, v_emp_name;
EXIT WHEN emp_cursor%NOTFOUND;
DBMS_OUTPUT.PUT_LINE(‘Employee ID: ‘ || v_emp_id || ‘, Name: ‘ || v_emp_name);
END LOOP;
CLOSE emp_cursor;
END;
4. Stored Procedures and Functions
PL/SQL allows you to write stored procedures and functions that can be executed on demand. These reusable blocks of code simplify complex operations and reduce redundancy in your SQL scripts.
Stored Procedure
A stored procedure is a named PL/SQL block that performs an action, such as modifying data. Procedures do not return values.
CREATE OR REPLACE PROCEDURE update_salary (p_emp_id IN NUMBER, p_salary IN NUMBER) IS
BEGIN
UPDATE employees
SET salary = p_salary
WHERE employee_id = p_emp_id;
COMMIT;
END;
Function
A function is similar to a procedure but returns a value. Functions are useful for encapsulating reusable business logic that can return values for use in SQL queries.
CREATE OR REPLACE FUNCTION get_employee_name (p_emp_id IN NUMBER) RETURN VARCHAR2 IS
v_name employees.first_name%TYPE;
BEGIN
SELECT first_name INTO v_name FROM employees WHERE employee_id = p_emp_id;
RETURN v_name;
END;
Functions can be called directly in SQL statements:
SELECT get_employee_name(100) FROM dual;
5. Exception Handling in PL/SQL
PL/SQL provides robust exception handling to manage errors that occur during program execution. Exceptions are predefined (like NO_DATA_FOUND
) or can be user-defined.
The exception handling section allows you to catch and manage runtime errors, ensuring that your program behaves predictably even in the face of unexpected issues.
BEGIN
— Code that might raise an exception
NULL; — Placeholder for actual code
EXCEPTION
WHEN NO_DATA_FOUND THEN
DBMS_OUTPUT.PUT_LINE(‘No data found.’);
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE(‘Error occurred: ‘ || SQLERRM);
END;
In this example:
- The NO_DATA_FOUND exception is handled when no rows are returned by a query.
- The OTHERS exception captures all other errors and outputs the error message using the SQLERRM function.
6. Triggers in PL/SQL
Triggers are special types of stored procedures that automatically fire in response to certain events on a table or view (like INSERT
, UPDATE
, DELETE
). They are useful for enforcing business rules, maintaining audit logs, or preventing invalid data changes.
Example of a trigger that logs every update to the employees
table:
CREATE OR REPLACE TRIGGER audit_employee_changes
AFTER UPDATE ON employees
FOR EACH ROW
BEGIN
INSERT INTO employee_audit_log (employee_id, old_salary, new_salary, change_date)
VALUES (:OLD.employee_id, :OLD.salary, :NEW.salary, SYSDATE);
END;
In this example:
- The trigger fires after any update to the employees table.
- It logs the employee ID, old salary, new salary, and the date of the change into an employee_audit_log table.
Advanced PL/SQL Concepts: Unlocking the Full Potential of Oracle’s Procedural Language
PL/SQL (Procedural Language for SQL) is a powerful extension of SQL that allows for procedural programming within Oracle databases. While mastering the basics of PL/SQL is crucial for any database developer, understanding advanced concepts can significantly improve the flexibility, performance, and maintainability of your database applications. In this blog, we will explore some of the advanced PL/SQL concepts that can help you unlock the full potential of Oracle’s procedural language.
1. Complex Cursors and Cursor Variables
a. Implicit vs. Explicit Cursors
PL/SQL offers two types of cursors: implicit cursors (automatically created by Oracle for single SQL queries) and explicit cursors (defined by the user to handle complex queries).
In advanced PL/SQL programming, cursor variables provide greater flexibility. A cursor variable is a reference to a cursor, and it allows for dynamic management of query results at runtime.
Cursor Variables:
Cursor variables (also known as REF CURSORs) allow for the dynamic execution of SQL queries at runtime and can be passed between programs. These cursor variables enable the retrieval of result sets and can be used to pass result sets back from stored procedures and functions.
Example:
sql
Copy code
DECLARE
TYPE ref_cursor IS REF CURSOR;
v_cursor ref_cursor;
v_employee_name employees.first_name%TYPE;
BEGIN
OPEN v_cursor FOR
SELECT first_name FROM employees WHERE department_id = 10;
FETCH v_cursor INTO v_employee_name;
DBMS_OUTPUT.PUT_LINE(v_employee_name);
CLOSE v_cursor;
END;
In this example, the ref_cursor
type is used to declare a cursor variable that can be opened and passed dynamically. It allows for flexible querying based on conditions at runtime, which is useful in complex applications where queries are not fixed.
2. Dynamic SQL (EXECUTE IMMEDIATE)
Dynamic SQL is an advanced feature of PL/SQL that allows you to build SQL statements dynamically at runtime and execute them. The EXECUTE IMMEDIATE
command is used to execute these dynamic SQL statements.
Dynamic SQL is particularly useful when you need to execute SQL statements with variable table names, column names, or conditions that cannot be determined in advance. This feature gives you the ability to construct SQL statements on-the-fly, making your applications more adaptable.
Use Cases of Dynamic SQL:
- Executing a SQL statement where table names or column names are unknown at compile time.
- Constructing complex queries dynamically based on user input.
- Executing DDL statements like
CREATE
,ALTER
, orDROP
.
Example:
DECLARE
v_table_name VARCHAR2(30) := ’employees’;
v_sql VARCHAR2(1000);
BEGIN
v_sql := ‘SELECT * FROM ‘ || v_table_name;
EXECUTE IMMEDIATE v_sql;
END;
In this example, the EXECUTE IMMEDIATE
statement constructs a dynamic SQL query by concatenating the table name and then executes the query. The table name (employees
) is passed as a variable, making the code adaptable to different tables.
3. PL/SQL Collections
PL/SQL Collections are powerful data structures that allow you to store multiple values in a single variable. Collections in PL/SQL are similar to arrays in other programming languages, and they come in three primary types:
- Associative Arrays: Key-value pairs, similar to hash maps or dictionaries.
- Nested Tables: A collection of elements that are similar to one-dimensional arrays, where each element is a row of data.
- Varrays (Variable-Size Arrays): Fixed-size arrays that can store a predefined number of elements.
Using collections allows you to efficiently handle large sets of data in memory and process them in PL/SQL programs.
Example of a Nested Table:
DECLARE
TYPE number_table IS TABLE OF NUMBER;
v_numbers number_table;
BEGIN
v_numbers := number_table(1, 2, 3, 4, 5);
FOR i IN 1..v_numbers.COUNT LOOP
DBMS_OUTPUT.PUT_LINE(‘Number: ‘ || v_numbers(i));
END LOOP;
END;
In this example, a nested table is used to store a series of numbers. We then loop through the collection and print each number using the DBMS_OUTPUT.PUT_LINE
function.
Example of an Associative Array:
DECLARE
TYPE emp_table IS TABLE OF VARCHAR2(100) INDEX BY BINARY_INTEGER;
v_employees emp_table;
BEGIN
v_employees(1) := ‘John Doe’;
v_employees(2) := ‘Jane Smith’;
DBMS_OUTPUT.PUT_LINE(‘Employee 1: ‘ || v_employees(1));
DBMS_OUTPUT.PUT_LINE(‘Employee 2: ‘ || v_employees(2));
END;
Associative arrays are useful when you need to store and access data using non-contiguous integer indexes or string keys.
4. Error Handling with Advanced Exception Management
PL/SQL provides robust exception handling to catch and manage runtime errors that may occur during program execution. Beyond basic error handling, advanced exception management includes user-defined exceptions, handling specific error codes, and using the PRAGMA EXCEPTION_INIT
directive to map custom exceptions to Oracle error numbers.
User-Defined Exceptions:
A user-defined exception allows you to define your own error conditions and handle them as needed. You can raise a user-defined exception using the RAISE
statement and catch it in the EXCEPTION
block.
Example:
DECLARE
e_overdraft EXCEPTION;
balance NUMBER := 1000;
withdrawal NUMBER := 1500;
BEGIN
IF withdrawal > balance THEN
RAISE e_overdraft;
END IF;
EXCEPTION
WHEN e_overdraft THEN
DBMS_OUTPUT.PUT_LINE(‘Insufficient funds for withdrawal.’);
END;
In this example, a custom exception (e_overdraft
) is raised when the withdrawal amount exceeds the balance. The exception is then caught in the EXCEPTION
block and handled accordingly.
5. Advanced Triggers
Triggers in PL/SQL are used to automatically perform actions in response to changes in a database, such as INSERT
, UPDATE
, or DELETE
. Advanced triggers allow for more sophisticated use cases, such as managing cascading changes, auditing, and ensuring data integrity.
Compound Triggers:
A compound trigger is a new type of trigger introduced in Oracle 11g. It allows you to group multiple actions (such as BEFORE
and AFTER
events) into a single trigger. This eliminates issues with conflicting triggers and improves maintainability.
Example:
CREATE OR REPLACE TRIGGER employee_salary_update
FOR INSERT OR UPDATE ON employees
COMPOUND TRIGGER
BEFORE STATEMENT IS
BEGIN
— Pre-statement logic
DBMS_OUTPUT.PUT_LINE(‘Trigger started…’);
END BEFORE STATEMENT;
AFTER STATEMENT IS
BEGIN
— Post-statement logic
DBMS_OUTPUT.PUT_LINE(‘Trigger completed.’);
END AFTER STATEMENT;
END;
Compound triggers allow for more organized and controlled event handling in complex database systems.
6. Optimizing PL/SQL Performance
Optimizing PL/SQL code is essential for improving the performance of database applications, especially when dealing with large datasets and complex operations. Some of the key techniques for optimizing PL/SQL performance include:
- Bulk Operations: PL/SQL provides mechanisms such as BULK COLLECT and FORALL to fetch and update large volumes of data in a single operation. These techniques reduce context switching between PL/SQL and SQL engines and improve performance.
Example of BULK COLLECT:
sql
Copy code
- Limit Context Switching: Reduce the number of context switches between SQL and PL/SQL engines by processing as much data as possible in the SQL layer.
- Use Efficient Data Types: Always choose the appropriate data types for variables and columns. For example, use NUMBER(10, 2) for currency instead of NUMBER, as it ensures better precision and performance.
Why PL/SQL Is Important
PL/SQL (Procedural Language for SQL) is an extension of SQL developed by Oracle, blending the simplicity of SQL with the power of procedural programming. It plays a pivotal role in database management and application development, making it indispensable for professionals in IT, database management, and software development. Here’s why PL/SQL is so important:
1. Enhanced SQL Capabilities
While SQL is powerful for querying data, it lacks procedural features like loops and conditional statements. PL/SQL fills this gap by allowing programmers to write complex scripts to manipulate data, execute transactions, and manage workflows efficiently.
Example:
A business can automate monthly payroll processing using PL/SQL blocks that calculate salaries, apply deductions, and generate pay slips, all within the database.
2. High Performance
PL/SQL minimizes the interaction between applications and the database by bundling multiple SQL operations into a single block of code. This reduces network latency and enhances performance, especially in applications with high transaction volumes.
Example:
A banking application can use PL/SQL to batch process thousands of financial transactions without frequent database calls, ensuring speed and reliability.
3. Improved Maintainability
PL/SQL allows you to create reusable program units like procedures, functions, and packages. This modular approach makes it easier to update, debug, and maintain code.
Example:
A retail system can use PL/SQL packages for inventory management, enabling consistent updates across all applications linked to the database.
4. Advanced Error Handling
With robust exception-handling features, PL/SQL ensures that errors are detected and managed gracefully, preventing system crashes and data corruption.
Example:
An e-commerce platform can use PL/SQL to handle issues like payment gateway failures, ensuring orders remain in a pending state for retry instead of being lost.
5. Tight Integration with Oracle Databases
PL/SQL is natively supported by Oracle databases, providing seamless integration and access to advanced features like triggers and dynamic SQL.
Example:
A healthcare application can use database triggers written in PL/SQL to log changes in patient records automatically for auditing and compliance.
6. Secure Data Operations
PL/SQL provides a secure way to execute database operations. By embedding business logic within the database, it reduces the risk of data breaches caused by unauthorized external access.
Example:
In financial systems, sensitive operations like loan approval workflows can be securely executed using PL/SQL procedures with restricted access.
7. Scalability and Flexibility
PL/SQL is highly scalable, making it suitable for applications ranging from small databases to enterprise-level systems handling massive datasets.
Example:
Telecommunication companies use PL/SQL to manage customer billing systems that process millions of records daily.
8. Cost Efficiency
By leveraging PL/SQL, organizations can optimize database operations, reduce application server load, and lower overall operational costs.
Example:
A logistics company can automate route optimization for shipments using PL/SQL, reducing manual effort and operational costs.
Conclusion
PL/SQL is an essential skill for anyone working with Oracle databases. By combining SQL with procedural logic, it empowers developers to create efficient, reliable, and scalable database applications. From basic SQL queries to advanced features like triggers, stored procedures, and complex error handling, PL/SQL offers a comprehensive toolset for database programming.
Learning PL/SQL not only boosts your development capabilities but also enhances your understanding of how to work more effectively with Oracle databases. Whether you’re just starting or looking to deepen your knowledge, mastering PL/SQL will significantly enhance your career as a database professional.
Start with the basics and gradually move on to more complex topics to unlock the full potential of Oracle’s powerful PL/SQL language.
Mastering the key PL/SQL constructs such as variables, control structures, cursors, exception handling, and stored procedures is essential for any developer working with Oracle databases. PL/SQL’s procedural capabilities offer enhanced flexibility, efficiency, and scalability for building powerful database applications.
By understanding these core concepts and their practical applications, you will be able to write efficient and maintainable code, automate complex tasks, and optimize database performance. As you advance in your PL/SQL journey, the ability to handle complex queries, business logic, and error management within Oracle databases will give you a significant edge as a database professional.
Mastering advanced PL/SQL concepts such as dynamic SQL, cursor variables, collections, advanced triggers, and efficient error handling can significantly enhance your ability to write efficient, scalable, and maintainable database applications. By leveraging these advanced features, you can optimize your database operations, handle complex data structures, and build sophisticated applications that take full advantage of Oracle’s procedural capabilities.
As you deepen your understanding of PL/SQL, you will be able to handle larger, more complex datasets, improve your application’s performance, and ensure data integrity, making you a more effective and efficient Oracle developer.
💻 Join Ignisys IT for PL/SQL Training! 📊
Are you ready to master PL/SQL and elevate your database management skills? Whether you’re a beginner or looking to enhance your expertise, Ignisys IT is the perfect place to start your journey into the world of PL/SQL programming.
Start your journey to becoming a PL/SQL expert with Ignisys IT and gain the skills that industry leaders value most!
Ignisys IT – Empowering Careers with Knowledge and Practical Expertise.
Leave a Reply