React JS vs JavaScript: Understanding Their Roles in Web Development
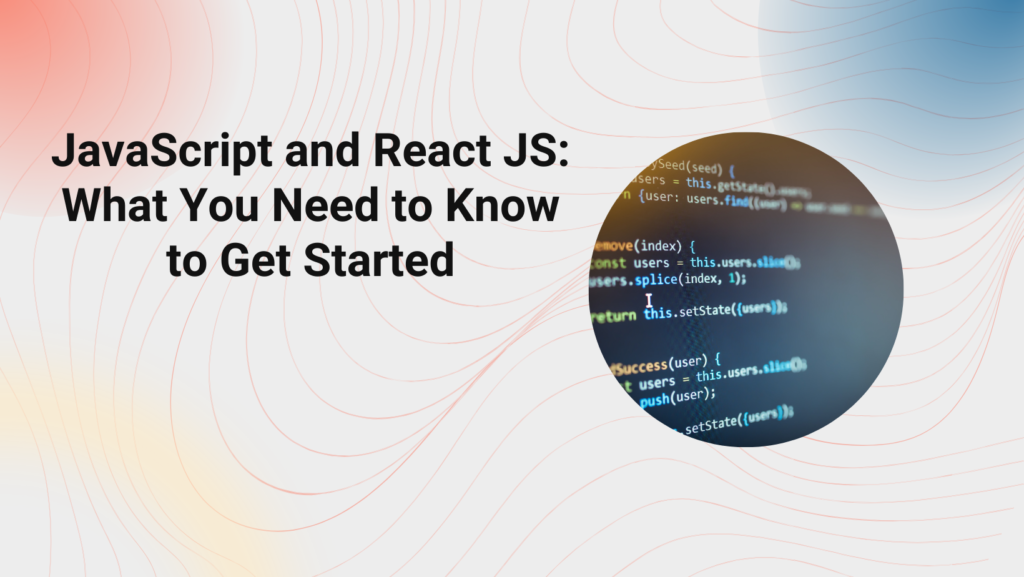
What is JavaScript?
JavaScript is one of the most widely used programming languages in the world, and it plays a crucial role in modern web development. If you’ve ever interacted with a website that had dynamic content, like animations, interactive forms, or even just a button that changes color when you hover over it, chances are JavaScript was behind the scenes making it all possible. But what exactly is JavaScript, and why is it so important? Let’s dive in.
The Basics: What is JavaScript?
JavaScript is a high-level, interpreted programming language that is primarily used to create and control dynamic website content. It was developed by Brendan Eich in 1995 while he was working at Netscape Communications Corporation, and it quickly became an essential part of the web, enabling developers to create interactive, user-friendly websites.
Unlike HTML, which defines the structure of web pages, and CSS, which controls their appearance, JavaScript adds interactivity to websites, allowing them to respond to user actions. Whether it’s displaying real-time data, animating graphics, or validating user input in forms, JavaScript makes the web a more dynamic and engaging place.
JavaScript in the Browser: The Client-Side Powerhouse
JavaScript is a client-side scripting language, which means it runs directly in the user’s web browser. When you visit a website, the HTML and CSS code are typically loaded first, setting up the structure and design of the page. Then, the JavaScript code is executed, enabling the page to react to user actions like clicks, keyboard input, and mouse movements.
This client-side execution is what allows JavaScript to create smooth, real-time interactions without needing to constantly communicate with the web server. For example, when you submit a form on a website, JavaScript can validate the input fields instantly, alerting you to any errors before the form is sent to the server.
Key Features and Capabilities of JavaScript
JavaScript is a versatile language that has grown far beyond its original scope. Some of its key features and capabilities include:
- Dynamic Typing: JavaScript is a dynamically typed language, meaning variables do not have fixed types. You can assign different types of values to the same variable during runtime, making JavaScript flexible but also requiring careful management to avoid type-related errors.
- Event-Driven Programming: JavaScript is inherently event-driven, meaning it can listen for and respond to various events triggered by user interactions, like clicks, key presses, or form submissions. This makes it ideal for creating interactive and responsive web applications.
- Asynchronous Programming: JavaScript supports asynchronous operations, allowing developers to perform tasks like fetching data from an API or reading a file without blocking the execution of other code. This is achieved through features like callbacks, promises, and async/await syntax.
- Object-Oriented Programming: JavaScript is also an object-oriented language, allowing developers to create reusable code components (objects) that can encapsulate data and behavior. JavaScript objects can be created using classes or directly as literal objects, providing a flexible approach to organizing and reusing code.
- Rich Ecosystem and Libraries: JavaScript boasts a vast ecosystem of libraries and frameworks that simplify and enhance web development. Tools like React, Angular, and Vue.js are built on top of JavaScript and enable developers to build complex, high-performance web applications with ease.
JavaScript Beyond the Browser: Server-Side and Full-Stack Development
While JavaScript is most commonly associated with client-side scripting, its role has expanded far beyond the browser. With the advent of Node.js, JavaScript can also be used for server-side development. Node.js allows developers to build scalable, high-performance web servers using JavaScript, making it possible to use a single language for both front-end and back-end development.
This capability has led to the rise of full-stack JavaScript development, where a single developer or team can build an entire web application using JavaScript across the stack. This unification simplifies development workflows and reduces the need for context-switching between different programming languages.
The Evolution and Standardization of JavaScript
JavaScript has undergone significant evolution since its creation. To ensure consistency and compatibility across different browsers, JavaScript is standardized through the ECMAScript (ES) specification. New features and improvements are introduced in periodic ECMAScript releases, with ES6 (also known as ECMAScript 2015) being one of the most significant updates, introducing features like classes, modules, and arrow functions.
Modern JavaScript continues to evolve, with each new ECMAScript version adding powerful features and syntax enhancements that make the language more efficient, readable, and capable of handling complex development tasks.
Why Learn JavaScript?
JavaScript is an essential skill for anyone looking to pursue a career in web development. Here are a few reasons why learning JavaScript is so valuable:
- Ubiquity: JavaScript is everywhere—virtually every website uses it in some form, making it an indispensable tool for web developers.
- Career Opportunities: JavaScript is one of the most in-demand programming languages in the job market, with opportunities ranging from front-end and back-end development to full-stack roles.
- Community and Resources: The JavaScript community is vast and active, with countless resources, tutorials, and libraries available to help developers of all skill levels.
- Flexibility: JavaScript’s ability to run on both the client and server-side, along with its use in mobile app development (via frameworks like React Native), means that learning JavaScript opens doors to a wide range of development opportunities.
- Constantly Evolving: JavaScript is a living language that continues to grow and adapt to new technologies, ensuring that it remains relevant in the ever-changing landscape of web development.
What is React JS?
React JS, commonly referred to simply as React, is a powerful and popular JavaScript library used for building user interfaces, particularly single-page applications where the user interacts with a web page without having to reload the page. Developed and maintained by Facebook, React was first released in 2013 and has since become one of the most widely adopted libraries for front-end web development.
Core Concepts of React JS
- Component-Based Architecture:
React is built around the idea of components—reusable, self-contained blocks of code that define how a portion of the user interface (UI) should appear and behave. Each component in React can manage its own state and render its own UI, making it easier to build complex applications by breaking them down into smaller, manageable pieces. - JSX (JavaScript XML):
JSX is a syntax extension for JavaScript that allows developers to write HTML-like code within JavaScript. It’s a syntactic sugar for React’s createElement() function, making the code more readable and easier to write. Although JSX is not required to use React, it is widely adopted because it allows for more intuitive and clean code. - Virtual DOM:
React introduces the concept of a Virtual DOM, an in-memory representation of the real DOM elements generated by React components. When the state of a component changes, React updates the Virtual DOM, compares it with the previous version, and then efficiently updates the real DOM only where changes have occurred. This process, known as reconciliation, ensures that updates to the UI are fast and efficient. - State and Props:
- State: State is an object that represents the dynamic data of a component. It is managed within the component (or in more advanced cases, using state management libraries) and determines how the component renders and behaves. When the state of a component changes, React re-renders the component to reflect the updated state.
- Props (Properties): Props are the data passed from a parent component to a child component. They are read-only, meaning that a child component cannot modify its own props but can use them to render its content dynamically. Props are crucial for making components reusable and for establishing relationships between components.
- Unidirectional Data Flow:
React follows a unidirectional data flow, meaning that data always flows from parent to child components through props. This makes the app easier to debug and understand, as the flow of data is predictable and consistent. Any changes to the application’s state trigger re-renders from the top-level component down to the lower-level components.
Advantages of React JS
- Performance:
The Virtual DOM and efficient diffing algorithms make React applications perform well even with complex UIs and large amounts of dynamic content. - Reusable Components:
React’s component-based structure encourages code reusability, which can significantly reduce development time and improve maintainability. - Strong Community and Ecosystem:
React has a vast and active community of developers, which means there are plenty of resources, libraries, tools, and extensions available to help with development. - SEO-Friendly:
React can be rendered on the server side, which means that the content can be indexed by search engines more effectively, improving the SEO performance of web applications. - Flexibility:
React can be used for various purposes, not just for web applications but also for mobile app development (with React Native), desktop applications, and even VR experiences.
Disadvantages of React JS
- Learning Curve:
While React is relatively easy to get started with, mastering it requires a good understanding of JavaScript, ES6+ features, and the various concepts introduced by React, such as hooks, context, and more. - Rapid Changes:
React’s ecosystem is constantly evolving, with new libraries, tools, and best practices emerging frequently. Keeping up with these changes can be challenging for developers. - JSX Complexity:
While JSX can make the code more readable, it also introduces an additional layer of complexity, as developers must be comfortable with both HTML and JavaScript.
Use Cases of React JS
- Single Page Applications (SPAs):
React is ideal for SPAs where the user experience needs to be fast and dynamic without frequent page reloads. - Interactive User Interfaces:
Applications that require rich interactivity, such as dashboards, data visualization tools, and form-based applications, benefit from React’s component-based architecture. - Mobile Applications:
React Native, a framework derived from React, allows developers to build mobile applications using the same principles, making it easier to share code between web and mobile platforms. - Progressive Web Apps (PWAs):
React is often used to build PWAs that offer a native app-like experience in the browser, with features like offline access,
JavaScript and React JS: What Are the Differences?
JavaScript and React JS are often discussed together, but they serve different purposes and have distinct characteristics. Understanding the differences between JavaScript, a fundamental programming language, and React JS, a popular JavaScript library, is essential for web developers. This blog explores the key differences between JavaScript and React JS, highlighting their roles, features, and how they complement each other in modern web development.
1. Definition and Purpose
- JavaScript: JavaScript is a high-level, interpreted programming language primarily used for adding interactivity and dynamic behavior to websites. It allows developers to manipulate the Document Object Model (DOM), handle events, perform asynchronous operations, and interact with web APIs. JavaScript is a core technology of the web, alongside HTML and CSS, and is used for both client-side and server-side programming.
- React JS: React JS is a JavaScript library developed by Facebook for building user interfaces (UIs), particularly single-page applications (SPAs). It focuses on creating reusable UI components and efficiently updating the user interface through its Virtual DOM. React helps in managing the view layer of web applications, making it easier to build complex and interactive UIs.
2. Core Technology vs. Library
- JavaScript: JavaScript is a core technology of the web and serves as a foundational language for developing web applications. It is versatile and can be used independently or in conjunction with various frameworks and libraries.
- React JS: React JS is a library built on top of JavaScript. It leverages JavaScript to offer a structured approach to building UIs with reusable components and state management. React provides a specific set of tools and conventions for developing user interfaces.
3. Learning Curve
- JavaScript: Learning JavaScript involves understanding basic syntax, data types, functions, control flow, and object-oriented concepts. While JavaScript itself is relatively straightforward, mastering advanced features like asynchronous programming, closures, and ES6+ syntax can be challenging.
- React JS: React has a steeper learning curve due to its component-based architecture, JSX syntax, and state management concepts. Developers need to grasp JavaScript fundamentals before diving into React to effectively use its features like components, props, state, and lifecycle methods.
4. DOM Manipulation
- JavaScript: JavaScript directly manipulates the DOM using methods like
getElementById
,querySelector
, andappendChild
. Developers handle DOM updates manually, which can become cumbersome and inefficient for large applications. - React JS: React uses a Virtual DOM to abstract and optimize the process of updating the real DOM. When a component’s state or props change, React calculates the difference between the current and previous Virtual DOMs and updates the real DOM efficiently. This approach improves performance and simplifies UI updates.
5. Component-Based Architecture
- JavaScript: JavaScript does not enforce a component-based architecture. Developers create functions and objects to manage different parts of the application, but there is no inherent structure for building reusable UI components.
- React JS: React promotes a component-based architecture where the UI is divided into reusable and self-contained components. Each component manages its own state and logic, making it easier to develop, maintain, and scale complex user interfaces.
6. State Management
- JavaScript: State management in JavaScript applications requires custom solutions or external libraries. Developers manually track and update the state of different parts of the application.
- React JS: React provides built-in state management within components through
useState
and class component state. For more complex state management, React can be integrated with libraries like Redux or Context API, offering a structured approach to managing global state.
7. JSX Syntax
- JavaScript: JavaScript does not have a built-in syntax for defining HTML structures. Developers use JavaScript to dynamically create and manipulate HTML elements.
- React JS: React introduces JSX (JavaScript XML), a syntax extension that allows developers to write HTML-like code within JavaScript. JSX makes it easier to define and manage UI components and their structure. JSX is compiled into JavaScript function calls that create React elements.
8. Event Handling
- JavaScript: JavaScript uses native event handling methods like
addEventListener
to attach and manage events such as clicks, form submissions, and key presses. - React JS: React uses a synthetic event system that wraps native browser events. This system provides a consistent event handling mechanism across different browsers and integrates seamlessly with React’s component model. Event handlers are passed as props to components and can be managed within the component’s logic.
9. Ecosystem and Libraries
- JavaScript: JavaScript has a vast ecosystem with numerous libraries and frameworks for different purposes, such as Angular, Vue.js, and Node.js. Developers choose from various tools depending on their project requirements.
- React JS: React has a rich ecosystem of tools and libraries specifically designed to work with React applications. Popular tools include React Router for routing, Redux for state management, and various component libraries like Material-UI and Ant Design.
10. Use Cases
- JavaScript: JavaScript is used for a wide range of tasks, from simple scripts and interactive web elements to complex server-side applications. It is versatile and applicable in various contexts.
- React JS: React is primarily used for building modern, dynamic user interfaces for web applications. It excels in scenarios requiring complex UI interactions, single-page applications, and reusable components.
How Much JavaScript is Needed to Learn React?
React has become a popular choice for building modern web applications, but before diving into React, a solid understanding of JavaScript is essential. React is built on JavaScript and leverages many of its features, so having a good grasp of JavaScript concepts will make learning React much smoother. We will explore how much JavaScript you need to know before you start learning React and why these skills are important.
Core JavaScript Concepts Needed for React
- Basic Syntax and Operators
- Variables: Understanding how to declare and use variables with let, const, and var.
- Data Types: Familiarity with primitive data types (e.g., strings, numbers, booleans) and complex data types (e.g., arrays, objects).
- Operators: Knowledge of arithmetic, comparison, logical, and assignment operators.
- Functions
- Function Declaration: Knowing how to define and invoke functions using both function declarations and function expressions.
- Arrow Functions: Understanding ES6 arrow functions for concise syntax and lexical this binding.
- Parameters and Return Values: Using function parameters and return values effectively.
- Control Flow
- Conditionals: Using if, else if, and else statements to control the flow of execution.
- Switch Statements: Employing switch statements for handling multiple conditions.
- Loops: Utilizing loops like for, while, and do…while to iterate over data.
- Objects and Arrays
- Object Manipulation: Understanding how to create, access, and modify objects and their properties.
- Array Methods: Using array methods such as map(), filter(), reduce(), and forEach() for handling collections of data.
- ES6+ Features
- Destructuring: Using object and array destructuring to extract values.
- Spread and Rest Operators: Applying … syntax for spreading and gathering values.
- Template Literals: Employing template literals for string interpolation.
- Asynchronous JavaScript
- Promises: Understanding promises for handling asynchronous operations.
- Async/Await: Using async and await for more readable asynchronous code.
- JavaScript Classes and Modules
- Classes: Familiarity with ES6 classes for creating objects and inheritance.
- Modules: Knowing how to use import and export statements to modularize code.
- Event Handling
- Events: Understanding how to handle events such as clicks, form submissions, and input changes.
Why These JavaScript Skills Are Important for React
- JSX Syntax: React uses JSX, a syntax extension that allows you to write HTML-like code within JavaScript. A good understanding of JavaScript syntax and concepts helps you understand and work with JSX effectively.
- State and Props: React components rely on state and props to manage and pass data. Knowledge of JavaScript objects and functions is crucial for managing these concepts.
- Component Lifecycle: React components have lifecycle methods that require a solid grasp of JavaScript functions and classes.
- Event Handling: React uses JavaScript events for user interactions. Understanding JavaScript event handling is necessary for implementing dynamic features in React.
- Data Manipulation: React often involves manipulating data and updating the UI based on state changes. Proficiency in JavaScript data handling methods is essential for effective React development.
How to Prepare
- Strengthen Your JavaScript Fundamentals: Ensure you are comfortable with core JavaScript concepts. Resources like MDN Web Docs, JavaScript.info, and interactive tutorials can help build a strong foundation.
- Practice Coding: Build small projects or solve coding challenges to apply JavaScript concepts in practical scenarios.
- Learn ES6+ Features: Familiarize yourself with modern JavaScript features such as arrow functions, destructuring, and async/await, which are commonly used in React.
- Explore Asynchronous Programming: Understanding how to handle asynchronous operations with promises and async/await will help you manage data fetching and other asynchronous tasks in React.
- Work with Classes and Modules: Practice using JavaScript classes and modules, as these concepts are integral to React’s component-based architecture.
Top 10 JavaScript Topics to Know Before Learning React JS
Before diving into React JS, having a solid grasp of key JavaScript concepts is crucial. React is built on JavaScript, and many of its features and functionalities rely on a good understanding of the language. Here’s an in-depth look at the top 10 JavaScript topics you should be familiar with before starting with React JS:
1. Variables and Data Types
- Variables: Understanding how to declare variables using var, let, and const is fundamental. let and const are part of ES6 and offer block scope, whereas var is function-scoped.
- Data Types: Familiarize yourself with primitive data types (strings, numbers, booleans, null, undefined, and symbols) and complex data types (objects, arrays, and functions). Knowing how to work with these types is essential for managing state and props in React.
2. Functions
- Function Declaration: Know how to define functions using function declarations and expressions. Understand the difference between them and how they affect hoisting.
- Arrow Functions: Learn about ES6 arrow functions, which provide a shorter syntax and lexical this binding. This is particularly useful for handling events and managing component methods in React.
- Higher-Order Functions: Functions that take other functions as arguments or return functions are crucial in React, especially when dealing with callbacks and rendering logic.
3. Control Flow
- Conditionals: Use if, else if, and else statements to control the flow of execution based on conditions. React often involves conditionally rendering components or elements based on certain criteria.
- Switch Statements: The switch statement can be useful for handling multiple conditions more cleanly than a series of if-else statements.
- Loops: Learn how to use loops like for, while, and do…while for iterating over data structures, which is often needed when rendering lists of items in React.
4. Objects and Arrays
- Object Manipulation: Understand how to create, access, and modify objects, including nested objects. This knowledge is vital for managing complex data structures in React.
- Array Methods: Familiarize yourself with array methods such as map(), filter(), reduce(), and forEach(). These methods are frequently used in React to transform and render data.
5. ES6+ Features
- Destructuring: Learn object and array destructuring to extract values more succinctly. This is commonly used in React to extract props and state.
- Spread and Rest Operators: Understand the … syntax for spreading elements and gathering them. This is useful in React for copying objects or arrays and handling function parameters.
- Template Literals: Use template literals for string interpolation, which is helpful for dynamically creating strings in React components.
6. Asynchronous JavaScript
- Promises: Know how to work with promises for handling asynchronous operations. React often involves data fetching from APIs, which relies on promises.
- Async/Await: Learn how to use async and await for writing asynchronous code in a more readable and synchronous-like manner.
7. JavaScript Classes and Prototypes
- Classes: Understand ES6 classes for creating objects and handling inheritance. React uses classes for components, especially in older versions.
- Prototypes: While less common in React, knowledge of prototypes and inheritance helps in understanding JavaScript’s object-oriented features.
8. Event Handling
- Event Listeners: Learn how to add and remove event listeners, handle events like clicks and form submissions, and use event objects. React’s event handling model builds upon these concepts, using a synthetic event system for consistency across browsers.
9. Closures and Scope
- Closures: Understand closures for managing variable scope and creating functions with private variables. Closures are useful in React for managing component state and encapsulating logic.
- Scope: Be familiar with lexical and dynamic scope to understand how variables and functions are accessed and managed in different contexts.
10. Modules and Import/Export
- Modules: Learn about ES6 modules and how to organize code using import and export. React applications are often built using modular code, making it crucial to understand how to structure and share code across different files.
Which One to Choose and When?
Choosing between using plain JavaScript and adopting a library like React depends on several factors, including the complexity of your project, your development goals, and your team’s familiarity with the tools. Here’s a detailed guide to help you decide which option is best for your needs:
When to Use Plain JavaScript
- Small Projects or Simple Tasks:
- Description: For small projects or tasks that involve basic interactivity, DOM manipulation, or simple web features, plain JavaScript is often sufficient.
- Example: Creating a simple form validation, handling basic user interactions, or adding dynamic effects to a static webpage.
- Learning and Experimentation:
- Description: If you’re new to web development or want to grasp fundamental concepts, working with plain JavaScript helps build a strong foundation.
- Example: Learning basic JavaScript syntax, functions, and event handling without additional abstractions.
- Performance Considerations:
- Description: For highly performance-sensitive applications where every millisecond counts, plain JavaScript might offer more control over optimization and fine-tuning.
- Example: Developing a real-time data visualization tool where minimizing overhead is crucial.
- No Need for Complex UI:
- Description: If your application does not require a complex user interface with dynamic state management or reusable components, plain JavaScript may be sufficient.
- Example: Building a simple static website or a small utility tool.
- Minimal Dependencies:
- Description: Projects with minimal dependencies or those aiming to avoid additional libraries can benefit from using plain JavaScript.
- Example: Integrating a few interactive features into an existing website without introducing a large framework.
When to Use React JS
- Complex User Interfaces:
- Description: For applications with complex, interactive user interfaces that require efficient state management and dynamic content updates, React is an ideal choice.
- Example: Building a single-page application (SPA) with multiple views, dynamic content, and interactive elements.
- Component-Based Architecture:
- Description: React’s component-based architecture allows you to create reusable and modular components, making it easier to manage and scale complex UIs.
- Example: Developing a large-scale application where components can be reused across different parts of the app, such as a dashboard with various widgets.
- State Management Needs:
- Description: If your application requires managing and synchronizing state across different parts of the UI, React provides built-in tools like useState and useReducer, as well as integration with state management libraries like Redux.
- Example: Implementing a shopping cart application where the state needs to be managed across multiple components.
- Efficient UI Updates:
- Description: React’s Virtual DOM optimizes the process of updating the real DOM, improving performance and ensuring that UI updates are handled efficiently.
- Example: Building a real-time chat application where frequent updates to the UI are necessary.
- Team Collaboration:
- Description: React’s component-based approach and ecosystem make it easier for teams to collaborate on large projects. Components can be developed and tested independently, facilitating modular development.
- Example: Working on a collaborative project where multiple developers are responsible for different parts of the UI.
- Ecosystem and Tooling:
- Description: React comes with a rich ecosystem of tools, libraries, and community support, which can significantly speed up development and provide solutions for common challenges.
- Example: Utilizing libraries like React Router for routing, or integrating with tools like Create React App for bootstrapping projects.
- Future Growth and Maintenance:
- Description: If you anticipate that your project will grow or require ongoing maintenance and updates, React’s modular architecture can make it easier to manage and scale over time.
- Example: Developing a web application with plans for future enhancements or expansions.
Conclusion
JavaScript is undeniably a cornerstone of modern web development, enabling the creation of interactive, dynamic web experiences that are integral to today’s digital landscape. From its early days as a simple scripting language to its current role in both client-side and server-side development, JavaScript has continually evolved to meet the needs of developers and users alike. Its flexibility and widespread adoption make it an essential skill for anyone looking to build web applications.
React JS, as a powerful library built on top of JavaScript, offers a structured approach to managing complex user interfaces. Its component-based architecture, efficient Virtual DOM, and strong ecosystem provide tools that streamline development, enhance performance, and facilitate collaboration. While React introduces additional concepts and a learning curve, its benefits for building scalable and interactive applications are substantial.
Choosing between plain JavaScript and React depends on the scope and requirements of your project. Plain JavaScript remains a robust choice for simpler tasks, learning purposes, and performance-critical applications where minimal dependencies are crucial. On the other hand, React shines in scenarios requiring sophisticated user interfaces, component reusability, and efficient state management.
In the end, understanding both JavaScript fundamentals and the advantages of libraries like React equips developers with the knowledge to select the right tool for their projects. Whether you’re just starting your journey or looking to enhance your skills, mastering JavaScript and exploring React opens doors to a wide array of development opportunities and prepares you for the evolving demands of web development.
Are you a student eager to start your tech career or an employee looking to upskill and stay ahead in the fast-paced IT industry? Ignisys IT is here to help you achieve your goals with our comprehensive training programs!
Leave a Reply