The Versatility of JavaScript: Unleashing the Power of Web Development and Reasons to Learn JavaScript
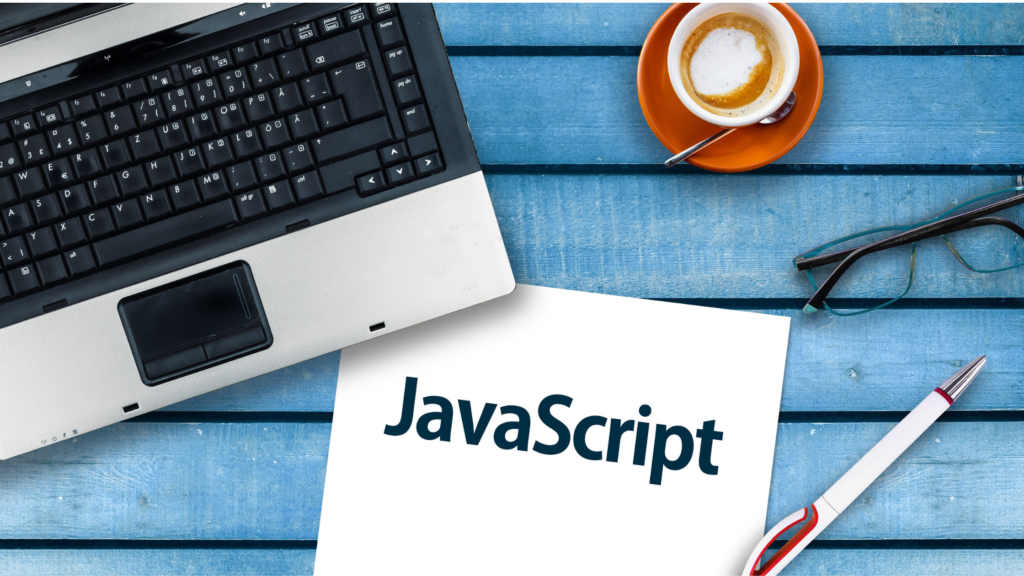
Introduction:
JavaScript is a versatile and ubiquitous programming language that powers websites’ interactive and dynamic elements. JavaScript plays a vital role in modern web development, from front-end development to server-side programming and beyond. In this blog post, we will explore the capabilities and advantages of JavaScript, highlighting its importance and versatility in creating engaging web applications.
Introduction to JavaScript
JavaScript is a versatile and widely used programming language that plays a fundamental role in web development. Initially developed by Netscape Communications in 1995, JavaScript was created to enable dynamic and interactive elements on web pages. Unlike Java, which is often confused with due to its name, JavaScript is a scripting language primarily used for client-side web development.
Role of JavaScript in Web Development
JavaScript is essential for creating dynamic, interactive, and responsive web applications. It operates directly within the web browser and is used to manipulate the Document Object Model (DOM), allowing developers to modify the content, structure, and style of web pages in real time based on user actions or other events.
Key Features of JavaScript
- Client-Side Scripting: JavaScript executes on the client’s browser, enabling dynamic changes to web pages without requiring server interaction.
- Event-Driven Programming: JavaScript is event-driven, responding to user actions like clicks, form submissions, or keyboard input to trigger specific behaviors.
- Versatile Syntax: JavaScript’s syntax is similar to other programming languages like Java and C, making it relatively easy to learn for developers familiar with structured programming.
- Extensive Ecosystem: JavaScript has a vast ecosystem of libraries and frameworks, such as React, Angular, and Vue.js, that simplify and streamline web development tasks.
- Support for Asynchronous Operations: JavaScript excels at handling asynchronous tasks, such as making HTTP requests to fetch data from servers, without blocking the user interface.
Basic Concepts of JavaScript
- Variables and Data Types: JavaScript supports various data types, including numbers, strings, booleans, arrays, objects, and more. Variables are used to store and manipulate data.
- Functions: JavaScript functions are blocks of reusable code designed to perform specific tasks. They can be invoked multiple times with different arguments.
- Control Flow: JavaScript uses conditional statements (if-else), loops (for, while), and switch-case statements to control the flow of program execution.
- DOM Manipulation: JavaScript interacts with the Document Object Model (DOM) of web pages to modify HTML elements, change styles, handle events, and update content dynamically.
Use Cases of JavaScript
- Enhancing User Interfaces: JavaScript is used to create interactive features like sliders, dropdown menus, form validations, and animations to enhance user experience.
- Form Validation: JavaScript validates user input on forms in real-time, providing immediate feedback to users and preventing invalid submissions.
- AJAX and Fetch: JavaScript enables Asynchronous JavaScript and XML (AJAX) requests to fetch data from servers without refreshing the entire web page. The newer Fetch API simplifies making HTTP requests and handling responses.
- Single-Page Applications (SPAs): JavaScript frameworks like React and Vue.js facilitate the development of SPAs, where content is dynamically loaded and updated without page refreshes.
Front-End Web Development with JavaScript
Front-end web development involves creating the visual and interactive components of a website that users interact with directly in their web browsers. JavaScript is a core technology used extensively in front-end development to enhance user experience, handle user interactions, and make web pages dynamic and responsive.
Role of JavaScript in Front-End Development
JavaScript is primarily responsible for manipulating the Document Object Model (DOM), which represents the structure of a web page as a hierarchical tree of objects. By interacting with the DOM, JavaScript can:
- Modify HTML Content: JavaScript can dynamically update HTML elements, change text content, update styles, and add or remove elements from the DOM based on user actions or other events.
- Handle User Interactions: JavaScript allows developers to respond to user interactions such as clicks, mouse movements, keyboard inputs, and form submissions. These interactions trigger specific actions or behaviors defined in JavaScript code.
- Implement Dynamic Features: JavaScript enables the creation of dynamic and interactive components like sliders, carousels, dropdown menus, accordions, modals, and interactive forms that enhance usability and engagement.
Key Concepts in Front-End Development with JavaScript
- Event Handling: JavaScript uses event listeners to detect and respond to user actions or events like clicks, hovers, and keystrokes. Event handlers execute specific functions in response to these events.
- DOM Manipulation: JavaScript interacts with the DOM to access and modify HTML elements, update their attributes, insert or remove elements, and dynamically change the structure and content of web pages.
- Asynchronous Operations: JavaScript supports asynchronous programming, allowing developers to perform non-blocking operations like fetching data from servers (AJAX requests) or executing time-consuming tasks without freezing the user interface.
- Cross-Browser Compatibility: Front-end developers must ensure that JavaScript code works consistently across different web browsers by adhering to web standards and using polyfills or libraries to address compatibility issues.
Tools and Libraries for Front-End Development
- JavaScript Libraries: Popular JavaScript libraries like jQuery simplify DOM manipulation, event handling, AJAX requests, and animation tasks, providing cross-browser compatibility and enhancing developer productivity.
- Front-End Frameworks: JavaScript frameworks such as React, Angular, and Vue.js facilitate the development of scalable, component-based user interfaces (UIs) for single-page applications (SPAs) and complex web applications.
- Development Tools: Front-end developers use integrated development environments (IDEs) like Visual Studio Code, code editors, and browser developer tools (e.g., Chrome DevTools) to write, debug, and test JavaScript code efficiently.
Best Practices in Front-End JavaScript Development
- Code Organization: Adopt modular JavaScript patterns (e.g., ES Modules) to encapsulate functionality, improve code reusability, and facilitate maintenance.
- Performance Optimization: Minimize JavaScript file sizes, bundle and compress scripts, and optimize DOM manipulation to improve page load times and overall performance.
- Accessibility: Ensure that JavaScript-driven interactions and components are accessible to users with disabilities by implementing keyboard navigation, focus management, and semantic HTML markup.
JavaScript Libraries and Frameworks
JavaScript libraries and frameworks play a crucial role in modern web development by providing pre-written code and reusable components that streamline development, enhance productivity, and facilitate the creation of robust web applications. These libraries and frameworks abstract complex tasks, simplify common development patterns, and promote best practices within the JavaScript ecosystem.
JavaScript Libraries
JavaScript libraries are collections of pre-written JavaScript code that provide ready-made functions and utilities for specific tasks. They are designed to simplify common web development tasks and address cross-browser compatibility issues. Some popular JavaScript libraries include:
- jQuery: jQuery is a lightweight and versatile JavaScript library that simplifies DOM manipulation, event handling, AJAX interactions, and animation tasks. It abstracts complex JavaScript code into simple, concise methods and offers cross-browser compatibility.
- Lodash: Lodash is a utility library that provides helper functions for common tasks like array manipulation, object iteration, functional programming, and data manipulation. It enhances JavaScript’s standard library and improves code readability and performance.
- Moment.js: Moment.js is a library for parsing, validating, manipulating, and formatting dates and times in JavaScript. It simplifies date-related operations and supports internationalization for working with dates in different locales.
- D3.js: D3.js (Data-Driven Documents) is a powerful library for creating dynamic and interactive data visualizations in web browsers. It enables developers to bind data to HTML elements, generate SVG-based charts and graphs, and implement complex data-driven visualizations.
JavaScript Frameworks
JavaScript frameworks provide comprehensive structures and guidelines for building scalable, maintainable, and feature-rich web applications. They enforce patterns like MVC (Model-View-Controller) or component-based architecture and often include built-in features for routing, state management, data fetching, and UI rendering. Some prominent JavaScript frameworks include:
- React: React is a declarative and component-based JavaScript library developed by Facebook for building interactive user interfaces. It uses a virtual DOM (Document Object Model) for efficient updates and supports server-side rendering for SEO-friendly web applications.
- Angular: Angular is a comprehensive framework maintained by Google for building large-scale, feature-rich web applications. It provides tools for dependency injection, component-based architecture, routing, forms handling, and state management.
- Vue.js: Vue.js is a progressive JavaScript framework that focuses on simplicity and flexibility. It allows developers to build interactive UI components using a combination of HTML templates and JavaScript logic. Vue.js supports reactivity, component composition, and seamless integration with existing projects.
- Express.js: While primarily a server-side framework for Node.js, Express.js simplifies the creation of RESTful APIs and web servers using JavaScript. It provides middleware for routing, request handling, and response processing, making it ideal for building back-end services in JavaScript.
Benefits of Using Libraries and Frameworks
- Productivity: Libraries and frameworks reduce development time by providing pre-built components and abstractions for common tasks, allowing developers to focus on application logic rather than low-level implementation details.
- Code Quality: By adhering to established patterns and best practices, libraries and frameworks promote code consistency, maintainability, and scalability across projects.
- Community Support: Popular libraries and frameworks have vibrant communities that contribute plugins, extensions, and documentation. This community support fosters learning, collaboration, and continuous improvement within the JavaScript ecosystem.
- Performance Optimization: Many frameworks employ efficient rendering techniques (e.g., virtual DOM) and offer built-in optimizations to enhance application performance and minimize resource consumption.
Asynchronous Programming with JavaScript
Asynchronous programming is a fundamental aspect of JavaScript that enables developers to execute multiple tasks concurrently without blocking the main execution thread. JavaScript’s asynchronous capabilities are essential for handling time-consuming operations like network requests, file I/O, and user interactions efficiently. Asynchronous programming in JavaScript is achieved through callback functions, promises, and the async/await syntax.
Callback Functions
Callback functions are a traditional approach to asynchronous programming in JavaScript. A callback is a function passed as an argument to another function, which is invoked once the asynchronous operation completes. Here’s an example of using callbacks for asynchronous file reading:
const fs = require(‘fs’);
fs.readFile(‘example.txt’, ‘utf8’, (err, data) => {
if (err) {
console.error(‘Error reading file:’, err);
} else {
console.log(‘File content:’, data);
}
});
In the above code, fs.readFile
reads the content of example.txt
asynchronously. Once the file is read, the callback function is invoked with an error (if any) and the file data.
Promises
Promises provide a cleaner and more structured way to handle asynchronous operations in JavaScript. A promise represents the eventual completion or failure of an asynchronous operation, allowing chaining of multiple operations and handling errors more effectively. Here’s how promises can be used:
const fs = require(‘fs’).promises;
fs.readFile(‘example.txt’, ‘utf8’)
.then(data => {
console.log(‘File content:’, data);
})
.catch(err => {
console.error(‘Error reading file:’, err);
});
In the above code, fs.promises.readFile
returns a promise that resolves with the file data or rejects with an error. The .then()
method handles the successful resolution, and the .catch()
method handles any errors that occur during the operation.
Async/Await
Async functions and the await
keyword provide a more intuitive and synchronous-like way to write asynchronous code in JavaScript. An async function returns a promise, and the await
keyword pauses the execution until the promise is resolved or rejected. Here’s an example using async/await:
const fs = require(‘fs’).promises;
async function readFileAsync() {
try {
const data = await fs.readFile(‘example.txt’, ‘utf8’);
console.log(‘File content:’, data);
} catch (err) {
console.error(‘Error reading file:’, err);
}
}
readFileAsync();
In the above code, readFileAsync
is an async function that uses await
to wait for the promise returned by fs.promises.readFile
to resolve. This approach makes the code more readable and easier to understand, especially for handling asynchronous operations sequentially.
Benefits of Asynchronous Programming
- Non-blocking: Asynchronous operations do not block the main execution thread, allowing other tasks to proceed concurrently.
- Improved Performance: By executing tasks concurrently, asynchronous programming enhances overall application performance and responsiveness.
- Error Handling: Asynchronous patterns like promises and async/await simplify error handling and propagation, making it easier to manage exceptions in asynchronous code.
- Scalability: Asynchronous programming is crucial for building scalable applications that can handle multiple requests and operations simultaneously.
Server-Side JavaScript: Node.js and Express.js
Node.js is a runtime environment that allows developers to run JavaScript server-side, outside the browser environment. It leverages Google’s V8 JavaScript engine to execute JavaScript code on the server, making it possible to build powerful and scalable backend applications using JavaScript.
Introduction to Node.js
Node.js enables developers to use JavaScript for both client-side (browser) and server-side (server) applications. It provides built-in modules and libraries that facilitate various server-side tasks like file system operations, networking, and HTTP handling.
Here’s a basic example of creating a simple HTTP server using Node.js:
const http = require(‘http’);
// Create an HTTP server that responds with “Hello, World!” to all requests
const server = http.createServer((req, res) => {
res.writeHead(200, { ‘Content-Type’: ‘text/plain’ });
res.end(‘Hello, World!\n’);
});
// Listen on port 3000
server.listen(3000, () => {
console.log(‘Server running at http://localhost:3000/’);
});
In the above code, we import the http
module, create an HTTP server using http.createServer()
, and listen on port 3000 for incoming requests. Node.js allows us to handle server-side logic using JavaScript, making it a popular choice for building web servers, APIs, and microservices.
Introduction to Express.js
Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features for building web and mobile applications. It simplifies the process of creating server-side applications by providing a layer of abstraction over Node.js’s built-in HTTP module.
Here’s an example of creating a basic web server using Express.js:
const express = require(‘express’);
const app = express();
// Define a route that responds with “Hello, Express!” when accessed
app.get(‘/’, (req, res) => {
res.send(‘Hello, Express!’);
});
// Start the server on port 3000
app.listen(3000, () => {
console.log(‘Express server running at http://localhost:3000/’);
});
In the above code, we import the express
module, create an Express application using express()
, define a route using app.get()
, and start the server using app.listen()
. Express.js simplifies routing, middleware handling, and request/response management, allowing developers to focus on building scalable and maintainable server-side applications.
Key Features of Node.js and Express.js
- Event-Driven Architecture: Node.js uses an event-driven, non-blocking I/O model that allows handling multiple concurrent connections efficiently.
- NPM (Node Package Manager): Node.js comes with NPM, a powerful package manager that provides access to thousands of reusable packages and modules.
- Middleware Support: Express.js allows developers to use middleware functions to process HTTP requests before reaching the route handler, enabling features like authentication, logging, and error handling.
- Routing: Express.js simplifies route handling and parameter extraction, making it easy to define RESTful APIs and web application routes.
- Template Engines: Express.js supports various template engines like EJS, Handlebars, and Pug (formerly Jade) for server-side rendering of dynamic content.
Benefits of Server-Side JavaScript
- Unified Language: Using JavaScript for both client-side and server-side development reduces context switching and makes it easier to share code between frontend and backend.
- Scalability: Node.js’s event-driven architecture and non-blocking I/O model allow building scalable and performant server applications that can handle concurrent requests efficiently.
- Large Ecosystem: The Node.js ecosystem, supported by NPM, offers a vast collection of open-source libraries and modules that simplify development tasks and accelerate project delivery.
- Community Support: Node.js and Express.js have active communities with abundant resources, tutorials, and plugins available, making it easier for developers to get started and troubleshoot issues.
Data Manipulation and Storage with JavaScript:
JavaScript offers powerful capabilities for manipulating data, working with arrays, objects, and JSON (JavaScript Object Notation). Let’s explore these techniques and discuss browser storage mechanisms using localStorage and sessionStorage for client-side data storage.
Working with Data in JavaScript
- Arrays and Objects:
- JavaScript arrays allow you to store multiple values in a single variable. You can manipulate arrays using methods like
push()
,pop()
,shift()
,unshift()
,slice()
,splice()
, andforEach()
for iteration. - Objects in JavaScript are collections of key-value pairs. You can access, modify, and iterate over object properties using dot notation (
object.property
) or bracket notation (object['property']
).
- JSON (JavaScript Object Notation):
- JSON is a lightweight data-interchange format widely used for data storage and communication between server and client. JavaScript provides built-in methods like
JSON.stringify()
andJSON.parse()
to serialize JavaScript objects into JSON strings and parse JSON strings back into JavaScript objects.
Client-Side Data Storage
- localStorage and sessionStorage:
localStorage
andsessionStorage
are client-side storage mechanisms available in modern browsers.localStorage
stores data with no expiration date, whilesessionStorage
stores data for the duration of the session (until the browser tab is closed).- You can use
setItem()
,getItem()
, andremoveItem()
methods to manage data inlocalStorage
andsessionStorage
.
// Example: Storing and retrieving data using localStorage
localStorage.setItem(‘username’, ‘john_doe’);
let username = localStorage.getItem(‘username’);
console.log(username); // Output: john_doe
localStorage.removeItem(‘username’);
JavaScript and Modern Web Technologies:
JavaScript plays a crucial role in various modern web technologies, empowering developers to build dynamic and interactive web applications.
- Single-Page Applications (SPAs):
- SPAs load a single HTML page and dynamically update the content using JavaScript. Frameworks like React, Angular, and Vue.js facilitate SPA development.
- Progressive Web Apps (PWAs):
- PWAs leverage modern web capabilities to deliver app-like experiences across devices. They use service workers and caching strategies for offline support and fast loading.
- Web Components:
- Web Components are reusable custom elements defined using HTML, CSS, and JavaScript. They enable encapsulation and reusability of UI components.
- APIs (Fetch API, Geolocation API):
- The Fetch API provides a modern interface for fetching resources (e.g., JSON data, images) asynchronously over the network.
- The Geolocation API retrieves geographical information (e.g., latitude, longitude) of the device running the web app.
Testing and Debugging JavaScript Code:
Writing reliable JavaScript code requires effective testing and debugging practices.
- Testing Frameworks (Jest, Jasmine):
- Jest and Jasmine are popular JavaScript testing frameworks that support unit testing, integration testing, and test-driven development (TDD).
- They provide APIs for writing and executing test cases, assertions, and mocks.
- Debugging Techniques:
- Browser developer tools (e.g., Chrome DevTools) offer debugging features like breakpoints, console logging, network inspection, and performance profiling.
- Understanding how to use these tools helps identify and resolve errors, optimize code performance, and ensure the quality of JavaScript applications.
By mastering data manipulation, modern web technologies, testing, and debugging techniques in JavaScript, developers can build robust, scalable, and performant web applications that meet user expectations and industry standards.
Top 10 Reasons to Learn JavaScript
When faced with a plethora of programming languages to choose from, it can be challenging to determine where to begin. However, acquiring knowledge in multiple languages is an asset that enhances a programmer’s marketability and demand. To embark on this journey, starting with a popular language experiencing significant growth is a strategic move. JavaScript, introduced by Netscape Communications in 1995, is distinct from Java and is hailed as a scripting language that enriches web pages, making them dynamic and responsive to user interactions. Originally named LiveScript, it underwent rebranding to capitalize on Java’s popularity, ultimately amassing a substantial following.
Let’s delve into 10 compelling reasons why mastering JavaScript is vital for your growth and development as a programmer:
- Most Popular Language: JavaScript reigns as the top programming language used by professional developers today, including a significant preference among back-end developers.
- In Your Browser: JavaScript is intrinsic to the internet, serving as the default language for web development within browsers.
- Beyond the Internet: JavaScript transcends conventional web applications, extending its utility to power smart devices, facilitate IoT interactions, develop native apps for iOS and Android, and construct cross-platform desktop applications.
- Ideal for Beginners: JavaScript’s ubiquitous presence across all web browsers eliminates the need for complex setup, enabling beginners to dive into coding promptly. It serves as an entry point for aspiring programmers, facilitating seamless progression into other languages like Python or Java.
- Easy to Learn: JavaScript boasts user-friendly syntax and intuitive constructs, abstracting complexities to facilitate swift comprehension by newcomers.
- Visual Effects and Interactivity: JavaScript empowers developers to craft visually captivating web pages adorned with animations, interactive maps, and engaging scrolling features, thereby enhancing user engagement and interaction.
- Versatility: JavaScript offers versatility across front-end (user-side) and back-end (server-side) development, bolstered by robust frameworks like Angular, React, and Node.js. This versatility underpins the creation of desktop, mobile, and web applications with ease.
- Influence in Big Data and Cloud: JavaScript’s JSON format serves as a prevalent data exchange standard on the internet, with Node.js emerging as a favored platform for constructing cloud-based applications.
- Valuable for Game Development: The adaptability and prowess of JavaScript in generating visual effects render it indispensable for game developers seeking to capitalize on its capabilities.
- Skyrocketing Career Potential: As businesses and organizations gravitate toward digital transformation, the demand for proficient JavaScript developers continues to surge. This burgeoning demand underscores JavaScript’s status as a coveted skill in today’s competitive job market.
Mastering JavaScript empowers developers with a multifaceted toolkit, enabling them to unlock diverse career prospects and cultivate indispensable skills pivotal for contemporary software development. Whether you are venturing into programming for the first time or expanding your technical repertoire, JavaScript offers a robust foundation for fostering growth, innovation, and professional success.
In conclusion, JavaScript represents more than just a programming language; it’s a gateway to the dynamic and ever-evolving world of web development. By delving into JavaScript’s capabilities, developers gain access to a versatile toolkit that spans front-end interactivity, server-side power, data manipulation, and integration with cutting-edge web technologies.
The journey through JavaScript begins with understanding its role in front-end development, leveraging the DOM for interactive web experiences. JavaScript libraries and frameworks like React and Angular streamline development, while Node.js opens doors to server-side programming, enabling full-stack solutions within a unified language ecosystem.
Data management in JavaScript, including array manipulation and JSON handling, equips developers with essential skills for handling data within applications. Additionally, JavaScript’s integration with modern web paradigms like SPAs, PWAs, and APIs showcases its adaptability to diverse development needs.
Ensuring code quality through testing frameworks like Jest and debugging tools enhances the reliability and maintainability of JavaScript applications, essential for delivering robust solutions in a rapidly evolving landscape.
For aspiring developers, JavaScript offers a compelling entry point into programming, supported by its popularity, accessibility, and extensive community. Beyond web development, JavaScript’s reach extends to IoT, mobile app development, game design, and cloud-based solutions, creating abundant career opportunities for those fluent in its language.
In summary, mastering JavaScript empowers developers to create engaging, responsive, and innovative web applications that address real-world challenges. Embracing JavaScript means embracing the future of web development and the endless possibilities it brings.
Leave a Reply