Understanding Loops in Java: Choosing the Right Loop for the Job
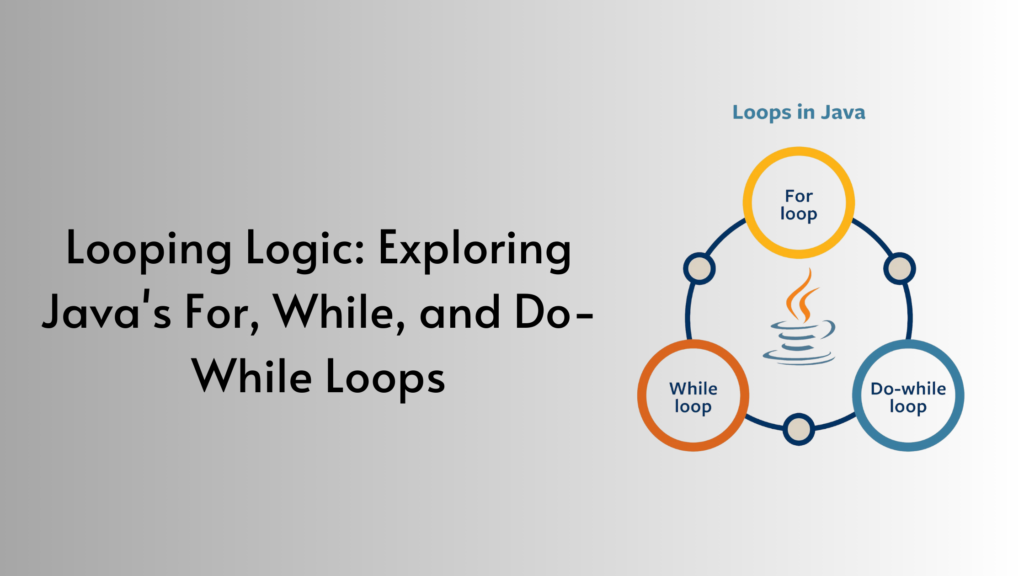
Loops are fundamental constructs in Java (and many other programming languages) that allow you to execute a block of code repeatedly. They play a crucial role in automating tasks, processing data, and controlling program flow. In this blog, we’ll explore the three primary types of loops in Java and understand when and how to use each one.
Types of Loops in Java
1. For Loop:
The for loop is ideal when you know the exact number of iterations required. It consists of three parts:
- Initialization: Initialize a control variable.
- Condition: Define a condition that, when false, exits the loop.
- Iteration: Update the control variable after each iteration.
Example: Calculating the Sum of Numbers
int sum = 0;
for (int i = 1; i <= 10; i++) {
sum += i;
}
System.out.println(“Sum of numbers from 1 to 10: ” + sum);
2. While Loop:
The while
loop is suitable when you don’t know the number of iterations in advance but have a specific condition for continuation.
Example: Rolling a Dice Until a Specific Number is Rolled (e.g., 6)
int roll;
int target = 6;
int attempts = 0;
while ((roll = (int)(Math.random() * 6) + 1) != target) {
attempts++;
System.out.println(“Attempt ” + attempts + “: Rolled ” + roll);
}
System.out.println(“It took ” + attempts + ” attempts to roll a ” + target);
3. Do-While Loop:
The do-while
loop is similar to the while
loop but guarantees that the loop block is executed at least once, even if the condition is initially false.
Example: User Input Validation
import java.util.Scanner;
int userGuess;
int secretNumber = 42;
Scanner scanner = new Scanner(System.in);
do {
System.out.print(“Guess the secret number: “);
userGuess = scanner.nextInt();
} while (userGuess != secretNumber);
System.out.println(“Congratulations! You guessed the secret number.”);
When to Use Each Type of Loop
- Use for loops when you know the number of iterations in advance or need to iterate through a known range of values.
- Use while loops when you want to loop based on a specific condition and are uncertain about the number of iterations required.
- Use do-while loops when you need to ensure that the loop block is executed at least once, even if the condition is initially false.
Conclusion
Loops are indispensable tools in Java programming, allowing you to automate repetitive tasks and efficiently process data. Choosing the right type of loop depends on your specific requirements, whether you know the number of iterations in advance or need to loop based on a condition. Mastery of loops is a fundamental skill for any Java developer.
Leave a Reply