A Comprehensive Guide to Docker: Empowering Modern Software Development
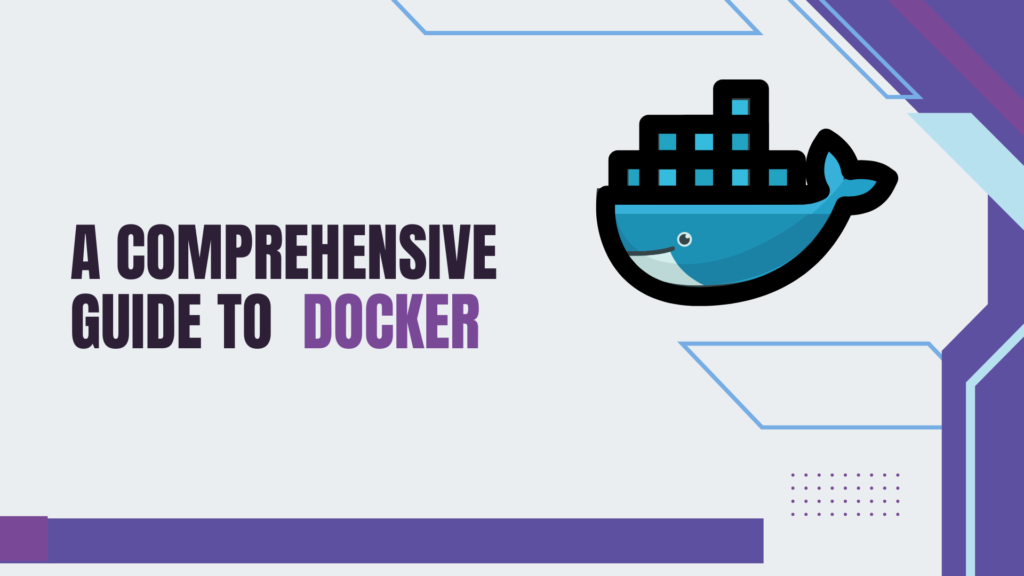
In today’s fast-paced digital landscape, efficiency and consistency are crucial for software development. Docker has emerged as one of the most powerful tools for developers, revolutionizing how applications are built, tested, and deployed. By leveraging containerization, Docker enables developers to streamline processes, reduce dependencies, and ensure their applications run reliably in different environments.
This blog takes a deep dive into Docker’s core concepts, practical use cases, and why it’s become a go-to solution for developers around the world.
What is Docker? A Brief Overview
Docker is an open-source platform designed to make it easier to create, deploy, and run applications using containers. A container is a lightweight, portable package that includes everything the software needs to run: code, libraries, dependencies, and configuration files. Containers are isolated but share the same OS kernel, making them more efficient than traditional virtual machines (VMs).
Example: Imagine you’re developing an application that requires a specific version of Python, along with a few external libraries. On your local machine, you install everything and the application works perfectly. However, when you move to another environment (e.g., a production server), the app may fail due to differences in system configurations or missing dependencies. Docker solves this issue by packaging everything your app needs into a container, ensuring it runs smoothly across different environments.
Key Components of Docker: How It Works
To understand how Docker operates, we need to break down its fundamental components:
1. Docker Images
A Docker image is a blueprint for containers. It contains everything needed to run an application—code, libraries, environment variables, and configuration files. Docker images are built from a set of instructions contained in a Dockerfile. Each step in the Dockerfile creates a new layer in the image, enabling modularity and efficiency, as unchanged layers are cached, speeding up subsequent builds.
For example, if you’re building a Python application, your Dockerfile might install Python, set the working directory, copy the source code, install dependencies, and run the application.
Here’s an example of a simple Dockerfile:
# Base image with Python 3
FROM python:3.8
# Set the working directory inside the container
WORKDIR /usr/src/app
# Copy the current directory contents into the container
COPY . .
# Install necessary dependencies
RUN pip install –no-cache-dir -r requirements.txt
# Command to run the application
CMD [“python”, “app.py”]
This file defines the environment in which your Python application will run, ensuring it works the same way on any system that supports Docker.
2. Docker Containers
A container is a runnable instance of a Docker image. Containers are isolated from each other and the host system, making them portable and secure. They have their own CPU, memory, file system, and network resources, allowing applications to run independently, even when they share the same host.
Containers are created, started, and stopped easily. When you run a container, Docker allocates the necessary resources and runs the application inside the container. Once stopped, the container can be removed or restarted, ensuring the same environment every time.
For example, to run a Python application built using the Dockerfile above, you could use the following command:
docker build -t mypythonapp .
docker run -d -p 5000:5000 mypythonapp
This creates a container from the image and runs it, exposing it on port 5000.
3. Docker Daemon and Client
Docker follows a client-server architecture. The Docker daemon (or engine) is responsible for managing containers, images, networks, and volumes. It listens for requests from the Docker client, which interacts with the daemon through the Docker API. The client is what you interact with directly through the Docker CLI (command-line interface) to execute commands like building images, running containers, and managing Docker services.
4. Docker Registry (Docker Hub)
Docker Hub is the default registry where Docker images are stored and shared. You can pull public images from Docker Hub (such as images for Node.js, MySQL, or Ubuntu) or push your own custom images. This makes it easier to share and collaborate on Docker images across teams or publicly with the community.
The Benefits of Using Docker
Docker offers several advantages over traditional development and deployment methods. Let’s break down some key benefits:
1. Portability
Docker containers can run anywhere: your local machine, a data center, or any cloud provider. This ensures that you don’t face environment-specific issues.
Example: If your development environment uses Ubuntu, and your production uses CentOS, Docker ensures that the application behaves the same across both environments by packaging everything into a consistent container.
2. Resource Efficiency
Unlike virtual machines, Docker containers don’t require a separate OS instance. They share the host’s OS kernel, making them lighter and faster to start, while using fewer system resources.
3. Rapid Deployment
Containers start up in seconds, making them ideal for continuous integration and deployment pipelines. This reduces downtime and accelerates development cycles.
4. Scalability
Docker makes it easy to scale applications. Using orchestration tools like Kubernetes or Docker Swarm, you can deploy thousands of containers to handle increased traffic or computing power.
Example: Suppose you’re running a web application that experiences a surge in traffic. Using Docker and Kubernetes, you can scale up by creating more containers to distribute the load, ensuring your application performs smoothly.
5. Consistency
Docker eliminates the “works on my machine” problem. By encapsulating all dependencies in a container, Docker ensures your application behaves consistently, whether in development, staging, or production.
Docker Use Cases: Real-World Applications
Docker’s flexibility makes it applicable across many different scenarios. Let’s explore a few practical examples of how Docker can be used:
1. Development and Testing Environments
One of Docker’s most popular use cases is setting up isolated development environments. Developers can spin up a container with specific configurations, test code, and shut it down without affecting their local environment.
Example: Suppose you’re working on a Python-based project that requires different versions of Python for testing compatibility. You can create separate Docker containers for Python 3.6, 3.7, and 3.8, and test your code in each one without any version conflicts.
2. Microservices Architecture
Docker is perfect for building microservices-based architectures, where each service runs in its own container. This separation of concerns enhances scalability, security, and maintainability.
Example: Consider an e-commerce application that has different services for handling payments, user authentication, and product management. With Docker, you can deploy each service in its own container, ensuring they run independently and can be updated without affecting other parts of the application.
3. Continuous Integration/Continuous Deployment (CI/CD)
Docker is widely used in CI/CD pipelines to automate the process of testing, building, and deploying applications. By running tests in Docker containers, developers ensure that the code is tested in a consistent environment, reducing the likelihood of bugs when moving from development to production.
Example: Using Jenkins and Docker, you can set up a pipeline that automatically pulls the latest code from GitHub, builds a Docker image, runs tests inside a container, and deploys the application if all tests pass.
4. Cloud Deployments
Docker makes it easy to deploy applications in the cloud. Many cloud providers offer services that integrate directly with Docker, such as AWS ECS (Elastic Container Service) and Azure Kubernetes Service (AKS).
Example: You can package your entire web application into a Docker container and deploy it to AWS using ECS, ensuring your app is scalable, resilient, and easily maintainable.
Docker vs. Virtual Machines: Understanding the Difference
While both Docker containers and virtual machines (VMs) offer application isolation, they operate quite differently. Let’s compare:
Virtual Machines
- Heavyweight: Each VM runs its own OS and requires a hypervisor to manage the underlying hardware.
- Slow Startup: VMs take minutes to start since they need to boot up a full operating system.
- Resource Intensive: VMs consume a lot of system resources (CPU, memory, and storage).
Docker Containers
- Lightweight: Containers share the host OS kernel and use less memory and storage.
- Fast Startup: Containers can start in seconds since they don’t require a full OS.
- Efficient: Containers allow for higher density, meaning you can run more containers on the same hardware compared to VMs.
Common Docker Commands: Getting Started with Docker
If you’re new to Docker, here are a few essential commands to get you started:
1. Build an image:
docker build -t myapp .
2. Run a container:
docker run -d -p 8080:80 myapp
3. List running containers:
docker ps
4. Stop a container:
docker stop container_id
5. Remove a container:
docker rm container_id
Docker in the Future: What’s Next?
As cloud-native technologies continue to evolve, Docker’s role in modern development is set to expand. Containers have become the foundation for distributed systems and microservices architectures. With the rise of Kubernetes as a leading orchestration platform and serverless computing, Docker will continue to play a critical role in building, deploying, and scaling applications.
In the future, we may see:
- Better integration with AI/ML workflows: Containers are already being used for training and deploying machine learning models. Docker could become even more integrated with AI frameworks.
- Enhanced security features: With growing concerns over container security, Docker will likely introduce more robust solutions to ensure containerized applications are more secure.
- Edge Computing: As edge computing grows, Docker containers will be key in deploying applications across distributed networks.
Practical Examples: Docker in Action
Docker’s versatility makes it a go-to tool for many industries and use cases. Let’s take a look at how organizations use Docker in real-world scenarios:
1. Modernizing Legacy Applications
Many organizations with legacy applications face the challenge of modernizing their infrastructure without entirely rewriting their code. Docker provides an easy solution by allowing these applications to be containerized. Once containerized, legacy applications can run on modern infrastructure without needing extensive refactoring.
For example, a financial institution might have an old Java-based application. By using Docker, they can package the entire application along with the necessary environment and dependencies, enabling the application to run on modern cloud platforms like AWS or Azure without code changes.
2. Streamlining Development Workflows
For software development teams, Docker simplifies development environments. Rather than configuring local development environments with specific versions of databases, languages, and libraries, developers can simply pull a Docker image that contains everything they need.
For instance, a team building a Node.js application with MongoDB can create separate Docker containers for Node.js and MongoDB. The developers can quickly spin up both services without manually installing them on their local machines. This ensures that all team members work in identical environments, reducing the risk of environment-related bugs.
3. Hybrid and Multi-Cloud Deployments
In today’s multi-cloud world, Docker’s portability makes it easier for companies to deploy their applications across different cloud providers. Whether you’re using AWS, Microsoft Azure, or Google Cloud, Docker containers can seamlessly run across any of these platforms, making it easier to adopt hybrid cloud strategies.
For example, a company might use AWS for their production environment but rely on Azure for development and testing. Docker ensures that the application can be deployed in both environments without compatibility issues.
Advanced Docker Features for Power Users
Once you’ve mastered the basics of Docker, you can explore advanced features to optimize your containerized applications:
1. Docker Compose
Docker Compose simplifies the management of multi-container applications. With Compose, you can define multiple services, networks, and volumes in a single YAML file, making it easy to manage complex applications.
For instance, let’s say you’re building an application that requires three services: a front-end, a back-end API, and a database. Using Docker Compose, you can define and start all three containers with a single command, ensuring they can communicate with each other seamlessly.
2. Docker Swarm
Docker Swarm provides native clustering and orchestration for Docker. It allows you to manage a cluster of Docker engines and schedule containers across different nodes. Swarm simplifies scaling applications and ensures high availability by distributing workloads across multiple containers.
3. Kubernetes Integration
While Docker handles containerization, Kubernetes has become the leading platform for container orchestration. Kubernetes automates the deployment, scaling, and management of containerized applications. Docker and Kubernetes are often used together to scale applications across distributed environments, with Kubernetes handling the complexities of managing large numbers of containers.
Conclusion: Why Docker is Essential for Modern Developers
Docker has revolutionized the software development process by providing a lightweight, efficient, and portable solution for containerizing applications. From its ability to provide consistent environments to its powerful support for microservices architectures, Docker is a must-have tool in every developer’s toolkit.
Whether you’re looking to modernize legacy applications, streamline development workflows, or scale applications in the cloud, Docker is the key to making your software development processes more efficient, scalable, and robust. As containerization continues to play a pivotal role in the future of technology, Docker is set to remain at the forefront of this transformation.
Are you looking to enhance your skills and advance your career in the tech industry? IgnisysIT offers a range of comprehensive training programs designed to equip you with the knowledge and hands-on experience needed to excel in today’s competitive job market.
Get Started Today!
Don’t miss out on the opportunity to elevate your career with IgnisysIT. Join our community of learners and unlock your potential.
For more information and to enroll in our training programs, please visit our website or contact us
Together, let’s build a brighter future in technology!
Leave a Reply