What is a Programming Language? Exploring Popular Languages and Their Uses
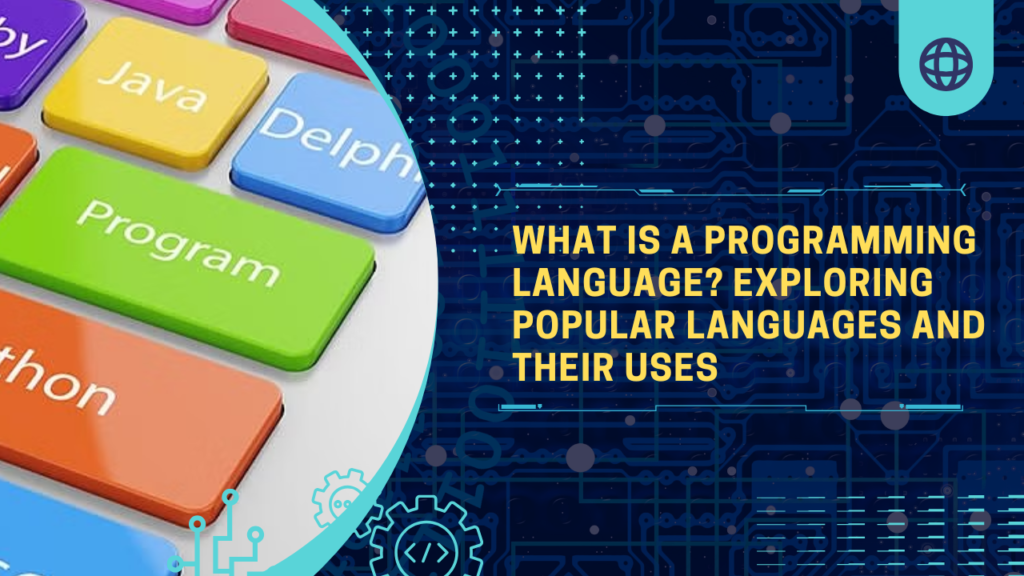
What is a Programming Language?
In the digital age, programming languages are essential tools that allow developers to communicate with computers. A programming language is a set of rules and syntax that enables programmers to write instructions that a computer can understand and execute. These languages vary in complexity and functionality, enabling the creation of everything from simple scripts to sophisticated software applications.
Programming languages are categorized into several types based on their levels of abstraction, paradigm (the style of programming), and purpose. Understanding these languages is crucial for anyone looking to enter the field of technology, as they form the foundation of software development.
How Programming Languages Work
Programming languages function through two main execution models:
- Compiled Languages: These languages require a compiler, which translates the entire source code into machine code before execution. This results in faster execution times, as the computer runs the pre-compiled code directly.
- Example: C++ is a compiled language that translates code into machine code, making it suitable for applications where performance is critical, such as video games and real-time simulations.
- Interpreted Languages: These languages are executed line by line by an interpreter. While this allows for greater flexibility and ease of debugging, it can lead to slower performance since the code is translated on-the-fly.
- Example: Python is an interpreted language known for its readability and simplicity, making it a popular choice for beginners and in data science.
Some languages, such as Java, utilize both compilation and interpretation, compiling code into an intermediate bytecode, which is then interpreted by the Java Virtual Machine (JVM).
Types of Programming Languages
Programming languages can be categorized by their level of abstraction from machine code, their paradigms, and their intended applications.
1. Low-Level Languages
Low-level languages provide little abstraction from a computer’s instruction set architecture. They offer fine control over hardware but require a deep understanding of the underlying hardware.
- Machine Code: The most basic level of programming, consisting of binary code (0s and 1s) that the computer can execute directly. It is not human-readable.
- Assembly Language: A slight abstraction over machine code that uses mnemonic codes (like MOV, ADD) instead of binary. It requires an assembler to translate the code into machine code. Assembly is used in performance-critical applications, such as operating systems and embedded systems.
2. High-Level Languages
High-level languages provide more abstraction, making them easier to read and write. They focus on programming logic rather than hardware details.
Popular Programming Languages and Their Applications
Here’s an overview of some widely-used programming languages, highlighting their key features and typical use cases:
1. Python
- Description: Python is an interpreted, high-level programming language known for its clear syntax and readability. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
- Key Features: Extensive standard library, dynamic typing, and strong community support.
- Use Cases: Data science, web development (using frameworks like Django and Flask), artificial intelligence, automation, and scientific computing.
- Example Application: A data analyst uses Python libraries such as Pandas for data manipulation and Matplotlib for data visualization to analyze trends in a dataset.
2. JavaScript
- Description: JavaScript is a high-level, interpreted programming language that is essential for front-end web development. It allows developers to create interactive and dynamic web pages.
- Key Features: Event-driven, functional programming capabilities, and extensive ecosystem of libraries and frameworks like React and Angular.
- Use Cases: Web development, mobile app development (using frameworks like React Native), and server-side development (with Node.js).
- Example Application: An e-commerce website uses JavaScript to handle user interactions, such as form submissions and dynamic content updates without needing to reload the page.
3. Java
- Description: Java is a versatile, object-oriented programming language designed to have minimal implementation dependencies. Its philosophy of “write once, run anywhere” allows Java applications to run on any device with a Java Virtual Machine (JVM).
- Key Features: Strongly typed, automatic memory management (garbage collection), and a rich API.
- Use Cases: Enterprise applications, mobile applications (Android development), web applications, and large-scale systems.
- Example Application: A banking application developed in Java handles transactions securely, leveraging Java’s robust libraries for cryptography and data management.
4. C++
- Description: C++ is an extension of the C programming language that incorporates object-oriented features. It is widely used for system/software development due to its performance and efficiency.
- Key Features: Direct memory manipulation, multiple inheritance, and support for both procedural and object-oriented programming.
- Use Cases: Game development, system programming, embedded systems, and real-time simulations.
- Example Application: A game developed in C++ utilizes complex algorithms to render 3D graphics and manage real-time interactions with users.
5. C#
- Description: C# is a modern, object-oriented programming language developed by Microsoft as part of its .NET initiative. It is designed for building Windows applications and enterprise solutions.
- Key Features: Strongly typed, rich library support, and interoperability with other languages in the .NET ecosystem.
- Use Cases: Game development (using Unity), enterprise applications, web applications (using ASP.NET), and desktop applications.
- Example Application: A mobile game developed using Unity leverages C# for game logic, physics, and user interactions.
6. SQL (Structured Query Language)
- Description: SQL is a domain-specific language used for managing and manipulating relational databases. It enables developers to query, insert, update, and delete data.
- Key Features: Declarative syntax, ability to handle complex queries, and support for transactions.
- Use Cases: Data analysis, database management, business intelligence, and reporting.
- Example Application: A business analyst uses SQL to extract sales data from a database to generate reports and visualize trends over time.
7. Ruby
- Description: Ruby is a dynamic, object-oriented language known for its simplicity and productivity. It emphasizes human-readable code and is often used in web development.
- Key Features: Flexible syntax, built-in support for object-oriented programming, and a rich set of libraries (gems).
- Use Cases: Web development (especially with the Ruby on Rails framework), automation scripts, and prototyping.
- Example Application: A startup builds its web application using Ruby on Rails to rapidly develop features and iterate based on user feedback.
8. PHP
- Description: PHP (Hypertext Preprocessor) is a server-side scripting language primarily used for web development. It is embedded within HTML code and is widely used for building dynamic websites.
- Key Features: Easy integration with databases, extensive libraries, and good support for various web servers.
- Use Cases: Web applications, content management systems (like WordPress), and server-side scripting.
- Example Application: A blogging platform developed in PHP allows users to create, edit, and manage posts easily.
9. Swift
- Description: Swift is a powerful programming language developed by Apple for iOS, macOS, watchOS, and tvOS application development. It is designed to be safe, fast, and expressive.
- Key Features: Type safety, optionals for handling null values, and modern syntax.
- Use Cases: Mobile app development for iPhone and iPad, server-side development, and system programming.
- Example Application: An iOS app developed in Swift provides a smooth user experience with responsive UI components and integration with device features.
10. R
- Description: R is a language and environment specifically designed for statistical computing and graphics. It is widely used among statisticians and data miners.
- Key Features: Extensive package ecosystem for statistical analysis, data visualization capabilities, and interactive graphics.
- Use Cases: Data analysis, statistical modeling, and academic research.
- Example Application: A research team uses R to analyze clinical trial data, employing statistical tests and creating visualizations to present their findings.
11. Go (Golang)
- Description: Go, also known as Golang, is an open-source programming language developed by Google. It is designed for simplicity and efficiency, especially in concurrent programming.
- Key Features: Strongly typed, garbage collected, and built-in support for concurrent programming with goroutines.
- Use Cases: Cloud services, server-side applications, and microservices architecture.
- Example Application: A cloud-based service built in Go efficiently handles thousands of concurrent requests, thanks to its lightweight goroutines.
12. Rust
- Description: Rust is a systems programming language focused on performance, safety, and concurrency. It is designed to prevent memory-related bugs, such as buffer overflows.
- Key Features: Strong emphasis on memory safety, zero-cost abstractions, and ownership model.
- Use Cases: Systems programming, game development, and web assembly.
- Example Application: A game engine developed in Rust utilizes its performance and safety features to manage resources and run complex game logic without crashes.
13. Kotlin
- Description: Kotlin is a modern programming language that is fully interoperable with Java and is officially supported for Android development. It aims to enhance developer productivity and reduce boilerplate code.
- Key Features: Concise syntax, null safety, and support for functional programming.
- Use Cases: Android app development, server-side applications, and web development.
- Example Application: An Android application built in Kotlin leverages its modern features for a streamlined user interface and efficient background processing.
14. Solidity
- Description: Solidity is a high-level programming language used for writing smart contracts on the Ethereum blockchain. It is statically typed and supports inheritance and complex user-defined types.
- Key Features: Designed for developing decentralized applications (dApps), extensive documentation, and support for Ethereum’s robust ecosystem.
- Use Cases: Blockchain development, decentralized finance (DeFi), and token creation.
- Example Application: A decentralized application (dApp) developed in Solidity allows users to trade tokens directly on the blockchain without intermediaries.
15. Qiskit
- Description: Qiskit is an open-source quantum computing framework developed by IBM. It provides tools for creating and running quantum programs on quantum computers.
- Key Features: Allows for easy circuit creation, simulation, and execution on real quantum devices.
- Use Cases: Quantum computing research, optimization problems, and complex simulations.
- Example Application: A researcher uses Qiskit to develop quantum algorithms that solve optimization problems faster than classical methods.
Conclusion
Programming languages are the backbone of software development, each offering unique capabilities tailored to various applications and industries. From web development with JavaScript to data analysis with Python and systems programming with Rust, understanding these languages and their appropriate use cases equips developers with the skills needed to thrive in the ever-evolving tech landscape. As technology continues to advance, new languages will emerge, shaping the future of software development and enabling innovative solutions to complex challenges.
By mastering a variety of programming languages, developers can adapt to changing industry demands, contribute to diverse projects, and ultimately drive technological innovation.
Here are the key takeaways from the blog post on programming languages:
Key Takeaways
- Definition and Importance: A programming language is a set of rules and syntax that allows developers to communicate with computers, making it essential for software development.
- Types of Programming Languages: Programming languages are categorized into low-level (machine and assembly) and high-level languages, each serving different purposes and providing varying levels of abstraction.
- Compiled vs. Interpreted Languages: Compiled languages (like C++) translate code into machine code before execution, offering performance benefits, while interpreted languages (like Python) execute code line by line, prioritizing ease of use and flexibility.
- Diversity of Languages: There is a wide array of programming languages tailored for specific applications, including:
- Python: Widely used in data science, web development, and automation.
- JavaScript: Essential for front-end web development and building interactive websites.
- Java: Common in enterprise applications and Android development.
- C++: Known for system programming and game development.
- Ruby: Popular for web development, especially with Ruby on Rails.
- Go: Designed for efficiency and scalability in cloud services and microservices.
- Rust: Focused on performance and safety in systems programming.
- Emerging Languages: New programming languages like Solidity (for blockchain development) and Qiskit (for quantum computing) are shaping future technologies, highlighting the industry’s evolving landscape.
- Language Choice and Project Needs: The choice of programming language depends on the project’s technical requirements, desired outcomes, and the specific features offered by the language.
- Versatile Skills for Developers: Understanding multiple programming languages equips developers with versatile skills to meet the dynamic needs of the tech industry, enabling them to adapt to emerging technologies and innovations.
These takeaways highlight the significance of programming languages in software development and the diverse ecosystem that developers can leverage to build innovative solutions in various domains.
🌟 Join Ignisys IT for Comprehensive Training Opportunities! 🌟
Are you looking to enhance your skills and advance your career in the tech industry? Ignisys IT offers a range of training programs designed to equip you with the knowledge and hands-on experience needed to thrive in today’s competitive job market.
📅 Start Your Journey Today! Don’t miss out on the opportunity to enhance your skills and propel your career forward. For more details on our training programs and to enroll, visit our website or contact us.
Leave a Reply